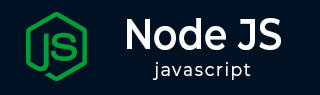
- Node.js Tutorial
- Node.js - Home
- Node.js - Introduction
- Node.js - Environment Setup
- Node.js - First Application
- Node.js - REPL Terminal
- Node.js - Command Line Options
- Node.js - Package Manager (NPM)
- Node.js - Callbacks Concept
- Node.js - Upload Files
- Node.js - Send an Email
- Node.js - Events
- Node.js - Event Loop
- Node.js - Event Emitter
- Node.js - Debugger
- Node.js - Global Objects
- Node.js - Console
- Node.js - Process
- Node.js - Scaling Application
- Node.js - Packaging
- Node.js - Express Framework
- Node.js - RESTFul API
- Node.js - Buffers
- Node.js - Streams
- Node.js - File System
- Node.js MySQL
- Node.js - MySQL Get Started
- Node.js - MySQL Create Database
- Node.js - MySQL Create Table
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB Get Started
- Node.js - MongoDB Create Database
- Node.js - MongoDB Create Collection
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB Query
- Node.js - MongoDB Sort
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js Modules
- Node.js - Modules
- Node.js - Built-in Modules
- Node.js - Utility Modules
- Node.js - Web Module
- Node.js Useful Resources
- Node.js - Quick Guide
- Node.js - Useful Resources
- Node.js - Dicussion
Node.js - Buffer.writeDoubleBE() Method
The Node.JS Buffer.writeDoubleBE() method helps to write a big-endian double number at a given offset to the buffer created.
A double 64-bit is also called FP64 or float64. When used it takes up 64-bit of memory in your computer.
The double 64-bit is divided as sign, exponent, and significand precision. The sign takes 1 bit, exponent takes 11 bit and the rest 53 (52 used for explicit storage) bits are taken by significand precision.
The sign bit tells about the sign of the number positive or negative.
The exponent is an 11-bit unsigned integer and has minimum value as 0 and maximum value as 2047.
The significand is 53 bits. For example, a number is 120.53. So here the integer value 12053 is the significand, $10^{-2}$ is power term, with −2 as the exponent.
Syntax
Following is the syntax of the Node.JS Buffer.writeDoubleBE() Method −
buf.writeDoubleBE(value[, offset])
Parameters
This method accepts two parameters. The same is explained below.
value − (required) The number to be written to the buffer.
offset − (optional) The offset here indicates the position to start writing. The offset has to be greater than or equal to 0 and less than or equal to buffer.length-8. The default value is 0.
Return value
The method Buffer.writeDoubleBE() writes the given number and returns offset plus the number of bytes written.
Example
To create a buffer, we are going to make use of NodeJS Buffer.alloc() method −
const buffer = Buffer.alloc(10); buffer.writeDoubleBE(167.8989, 0); console.log(buffer);
Output
The offset we have used with this method is 0. On execution the given number will be written from the 0th position to the buffer created. The buffer length created for the above is 10. So we can only use the offset with values from 0 to 2. If any value >2 it will give an error ERR_OUT_OF_RANGE.
<Buffer 40 64 fc c3 c9 ee cb fb 00 00>
Example
In this example will use an offset that is greater than buffer.length − 8. It should throw an error as shown below.
const buffer = Buffer.alloc(10); buffer.writeDoubleBE(167.8989, 3); console.log(buffer);
Output
internal/buffer.js:83 throw new ERR_OUT_OF_RANGE(type || 'offset', ^ RangeError [ERR_OUT_OF_RANGE]: The value of "offset" is out of range. It must be >= 0 and <= 2. Received 3 at boundsError (internal/buffer.js:83:9) at checkBounds (internal/buffer.js:52:5) at Buffer.writeDoubleBackwards [as writeDoubleBE] (internal/buffer.js:913:3) at Object.<anonymous> (C:\nodejsProject\src\testbuffer.js:2:8) at Module._compile (internal/modules/cjs/loader.js:1063:30) at Object.Module._extensions..js (internal/modules/cjs/loader.js:1092:10) at Module.load (internal/modules/cjs/loader.js:928:32) at Function.Module._load (internal/modules/cjs/loader.js:769:14) at Function.executeUserEntryPoint [as runMain] (internal/modules/run_main.js:72:12) at internal/main/run_main_module.js:17:47 { code: 'ERR_OUT_OF_RANGE' }
Example
To create a buffer, we are going to make use of Buffer.allocUnsafe() method −
const buffer = Buffer.allocUnsafe(10); buffer.writeDoubleBE(167.8989); console.log(buffer);
Output
The offset is not used, by default it will be taken as 0. So the output for above is as shown below.
<Buffer 40 64 fc c3 c9 ee cb fb 00 00>