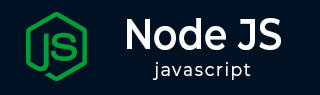
- Node.js Tutorial
- Node.js - Home
- Node.js - Introduction
- Node.js - Environment Setup
- Node.js - First Application
- Node.js - REPL Terminal
- Node.js - Command Line Options
- Node.js - Package Manager (NPM)
- Node.js - Callbacks Concept
- Node.js - Upload Files
- Node.js - Send an Email
- Node.js - Events
- Node.js - Event Loop
- Node.js - Event Emitter
- Node.js - Debugger
- Node.js - Global Objects
- Node.js - Console
- Node.js - Process
- Node.js - Scaling Application
- Node.js - Packaging
- Node.js - Express Framework
- Node.js - RESTFul API
- Node.js - Buffers
- Node.js - Streams
- Node.js - File System
- Node.js MySQL
- Node.js - MySQL Get Started
- Node.js - MySQL Create Database
- Node.js - MySQL Create Table
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB Get Started
- Node.js - MongoDB Create Database
- Node.js - MongoDB Create Collection
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB Query
- Node.js - MongoDB Sort
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js Modules
- Node.js - Modules
- Node.js - Built-in Modules
- Node.js - Utility Modules
- Node.js - Web Module
- Node.js Useful Resources
- Node.js - Quick Guide
- Node.js - Useful Resources
- Node.js - Dicussion
Node.js - Buffer.writeInt16LE() Method
The Buffer.writeInt16LE() method helps in writing a signed 16-bit integer at a given offset to the buffer in little endian form. The integer written to the buffer is represented as two's complement signed values.
The range of values to be stored with a 16-bit signed integer is −32768 to 32767.
Two's complement is mathematical operation on binary numbers. To get the two's complete of any given binary number it will be as follows −
Binary No: 101011
Step 1 − First find the one's complement of binary number: 101011. To get the one's complete you need to make 1 as 0 and 0 as 1. So the one's complement of the above binary number is: 010100.
Step 2 − Now for the one's complement from step 1 add 1 to it.
I.e. 010100 + 1 = 010101. So 010101 is the two's complement of binary number 101011.
Syntax
Following is the syntax of the Node.JS Buffer.writeInt16LE() Method −
buf.writeInt16LE(value[, offset])
Parameters
This method accepts two parameters. The same is explained below.
value − (required)A signed 16-bit integer to be written to the buffer.
offset − The offset that indicates the position to start reading. The offset is greater than or equal to 0 and also less than or equal to buffer.length-2. The default value is 0.
Return value
The method buffer.writeInt16LE() writes the given value and returns offset plus the number of bytes written.
Example
To create a buffer, we are going to make use of NodeJS Buffer.alloc() method −
const buffer = Buffer.alloc(10); buffer.writeInt16LE(0x0910, 0); console.log(buffer);
Output
The offset we have used is 0. On execution the value starting from 0th position will be written to the buffer created. The buffer length created for the above is 10. So we can only use the offset with values from 0 to 8. If any value >8 it will give an error ERR_OUT_OF_RANGE.
<Buffer 10 09 00 00 00 00 00 00 00 00>
Example
In this example will use an offset that is greater than buffer.length - 2. It should throw an error as shown below.
const buffer = Buffer.alloc(10); buffer.writeInt16LE(0x0910, 9); console.log(buffer);
Output
internal/buffer.js:83 throw new ERR_OUT_OF_RANGE(type || 'offset', ^ RangeError [ERR_OUT_OF_RANGE]: The value of "offset" is out of range. It must be >= 0 and <= 8. Received 9 at boundsError (internal/buffer.js:83:9) at checkBounds (internal/buffer.js:52:5) at checkInt (internal/buffer.js:71:3) at writeU_Int16LE (internal/buffer.js:719:3) at Buffer.writeInt16LE (internal/buffer.js:862:10) at Object.<anonymous> (C:\nodejsProject\src\testbuffer.js:2:8) at Module._compile (internal/modules/cjs/loader.js:1063:30) at Object.Module._extensions..js (internal/modules/cjs/loader.js:1092:10) at Module.load (internal/modules/cjs/loader.js:928:32) at Function.Module._load (internal/modules/cjs/loader.js:769:14) { code: 'ERR_OUT_OF_RANGE' }
Example
To create a buffer, we are going to make use of Buffer.allocUnsafe() method −
const buffer = Buffer.allocUnsafe(10); buffer.writeInt16LE(0x0910); console.log(buffer);
Output
The offset is not used, by default it will be taken as 0. So the output for above is as shown below −
<Buffer 00 00 11 45 01 00 00 00 00 00>
To Continue Learning Please Login