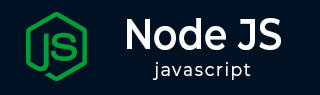
- Node.js Tutorial
- Node.js - Home
- Node.js - Introduction
- Node.js - Environment Setup
- Node.js - First Application
- Node.js - REPL Terminal
- Node.js - Command Line Options
- Node.js - Package Manager (NPM)
- Node.js - Callbacks Concept
- Node.js - Upload Files
- Node.js - Send an Email
- Node.js - Events
- Node.js - Event Loop
- Node.js - Event Emitter
- Node.js - Debugger
- Node.js - Global Objects
- Node.js - Console
- Node.js - Process
- Node.js - Scaling Application
- Node.js - Packaging
- Node.js - Express Framework
- Node.js - RESTFul API
- Node.js - Buffers
- Node.js - Streams
- Node.js - File System
- Node.js MySQL
- Node.js - MySQL Get Started
- Node.js - MySQL Create Database
- Node.js - MySQL Create Table
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB Get Started
- Node.js - MongoDB Create Database
- Node.js - MongoDB Create Collection
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB Query
- Node.js - MongoDB Sort
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js Modules
- Node.js - Modules
- Node.js - Built-in Modules
- Node.js - Utility Modules
- Node.js - Web Module
- Node.js Useful Resources
- Node.js - Quick Guide
- Node.js - Useful Resources
- Node.js - Dicussion
Node.js - Buffer.fill() Method
The NodeJS buffer.fill() method will fill the buffer with the given value. If the range inside the buffer is not specified it will fill the entire buffer.
Syntax
Following is the syntax of the NodeJS fill() method −
buf.fill(value[, offset[, end]][, encoding])
Parameters
The method buffer.fill() takes in four parameters. The first parameter value is mandatory, while the rest are optional.
value − This is a mandatory parameter. It's the value that you want the buffer to be filled with.
offset − It's an optional field. The offset tells the start point from which the value can be filled. The default value is 0.
end − It's an optional field. The end tells where the buffer filling has to stop. The default value is the length of the buffer.
encoding − It's an optional field. The encoding to be considered if the value provided is a string. The default is utf8.
Return value
It returns a buffer object that will be filled with the value given.
Example
In the example below we have created a buffer using NodeJS Buffer.alloc() and assigned 10 bytes to it. Later using NodeJS buffer.fill() the value 'ab' is filled in the 10 bytes memory space.
const buffer = Buffer.alloc(10); buffer.fill('ab'); console.log("The final string from buffer is "+buffer.toString());
Output
Following is the output of the above program −
The final string from buffer is ababababab
Example
In this example let us fill the buffer partly using the offset and end parameters.
The space allocated using Buffer.alloc() is 10 bytes. The string 'ab' is used to fill the buffer starting from 5 to 10.
const buffer = Buffer.alloc(10); buffer.fill('ab', 5, 10); console.log(buffer); console.log("The final string from buffer is "+buffer.toString())
Output
<Buffer 00 00 00 00 00 61 62 61 62 61> The final string from buffer is ababa
Example
In this example let us fill the buffer with integer values as shown below −
const buffer = Buffer.alloc(10); console.log(buffer); buffer.fill(1); console.log(buffer);
Output
Prior to filling the buffer, you will see all zeros when you console the buffer. Later the integer 1 is used to fill the buffer.The output is as follows −
<Buffer 00 00 00 00 00 00 00 00 00 00> <Buffer 01 01 01 01 01 01 01 01 01 01>
Example
In this example will make use of encoding.The encoding can be used only if the value is string.
const buffer = Buffer.alloc(10); console.log(buffer); buffer.fill("aazz","hex"); console.log(buffer);
Output
The hex encoding is used in the above example. The output after encoding is applied is as shown above.
<Buffer 00 00 00 00 00 00 00 00 00 00> <Buffer aa aa aa aa aa aa aa aa aa aa>
Example
You can also make use of a buffer as a value to fill another buffer.
const buffer1 = Buffer.allocUnsafe(8); buffer1.fill(Buffer.from('Hello')); console.log("The string is "+buffer1.toString());
Output
In the example we have created a buffer called buffer1 and allocated 8 bytes to it. Another buffer is created using Buffer.from() method with string value "Hello" and used as a value to buffer1.
The string is HelloHel
To Continue Learning Please Login