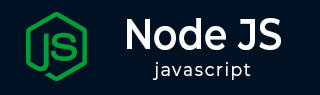
- Node.js Tutorial
- Node.js - Home
- Node.js - Introduction
- Node.js - Environment Setup
- Node.js - First Application
- Node.js - REPL Terminal
- Node.js - Command Line Options
- Node.js - Package Manager (NPM)
- Node.js - Callbacks Concept
- Node.js - Upload Files
- Node.js - Send an Email
- Node.js - Events
- Node.js - Event Loop
- Node.js - Event Emitter
- Node.js - Debugger
- Node.js - Global Objects
- Node.js - Console
- Node.js - Process
- Node.js - Scaling Application
- Node.js - Packaging
- Node.js - Express Framework
- Node.js - RESTFul API
- Node.js - Buffers
- Node.js - Streams
- Node.js - File System
- Node.js MySQL
- Node.js - MySQL Get Started
- Node.js - MySQL Create Database
- Node.js - MySQL Create Table
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB Get Started
- Node.js - MongoDB Create Database
- Node.js - MongoDB Create Collection
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB Query
- Node.js - MongoDB Sort
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js Modules
- Node.js - Modules
- Node.js - Built-in Modules
- Node.js - Utility Modules
- Node.js - Web Module
- Node.js Useful Resources
- Node.js - Quick Guide
- Node.js - Useful Resources
- Node.js - Dicussion
Node.js - Buffer.readBigUInt64BE() Method
The Node.JS Buffer.readBigUInt64BE() method helps to read an unsigned big-endian 64-bit integer at a given offset from the current buffer object.
In computer programming unsigned integers refers to a positive integer and signed integer refers to positive and negative values.
The minimum value with unsigned integers for 64-bit is 0 and maximum value is $2^{64}$-1.
Syntax
Following is the syntax of the Node.JS Buffer.readBigUInt64BE() Method -
buf.readBigUInt64BE([offset])
Parameters
offset − The offset that indicates the position to start reading. The offset is greater than or equal to 0 and also less than or equal to buffer.length-8. The default value is 0.
Return value
This method returns the 64-bit unsigned integer value of the contents of the current buffer at the given offset.
You can also make use of the alias function available: buf.readBigUint64BE(offset).
Example
In the following example, we are going to create a buffer and we are going to make use of NodeJS Buffer.from() method.
The offset we have used with this method is 0. The 64-bit unsigned integer at the 0th position will be returned. The buffer length created for the above is 8. So we can only use the offset with the value 0. If any value >0 it will give an error ERR_OUT_OF_RANGE.
const buffer = Buffer.from([0x00, 0x00, 0x00, 0x00, 0xff, 0xff, 0xff, 0xff]); console.log("buffer stored in memory as", buffer); console.log("Reading at big unsigned integer at offset 0:", buffer.readBigUInt64BE(0));
Output
buffer stored in memory as <Buffer 00 00 00 00 ff ff ff ff> Reading at big unsigned integer at offset 0: 4294967295n
Example
Let us create a buffer with 16 bits and see the value returned using the Node.JS Buffer.readBigUInt64BE() method.
const buffer = Buffer.from([0x00, 0x00, 0x00, 0x00, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff,0xff, 0xff, 0xff, 0xff]); console.log("Length of buffer is ", buffer.length); console.log("Reading at big integer at offset 2:", buffer.readBigUInt64BE(2));
Output
In the above example, the length of the buffer created is 16. So for offset, we can use the value from 0 to 8. The output of the above code, when executed, is as shown below −
Length of buffer is 16 Reading at big integer at offset 2: 281474976710655n
Example
This example will check on the error if the offset is greater than the buffer.length -8. Let us create a buffer having a length of 8 and with that, you can offset the value as 0.
const buffer = Buffer.from([0x00, 0x00, 0x00, 0x00, 0xff, 0xff, 0xff, 0xff]); console.log("buffer length is ", buffer.length); console.log("Reading at big integer at offset 1:", buffer.readBigUInt64BE(1));
Output
Since we have used an offset greater than 0 in the above program, it will throw an error as shown below −
buffer length is 8 internal/buffer.js:83 throw new ERR_OUT_OF_RANGE(type || 'offset', ^ RangeError [ERR_OUT_OF_RANGE]: The value of "offset" is out of range. It must be >= 0 and <= 0. Received 1 at boundsError (internal/buffer.js:83:9) at Buffer.readBigUInt64BE (internal/buffer.js:114:5) at Object.<anonymous> (C:\nodejsProject\src\testbuffer.js:3:59) at Module._compile (internal/modules/cjs/loader.js:1063:30) at Object.Module._extensions..js (internal/modules/cjs/loader.js:1092:10) at Module.load (internal/modules/cjs/loader.js:928:32) at Function.Module._load (internal/modules/cjs/loader.js:769:14) at Function.executeUserEntryPoint [as runMain] (internal/modules/run_main.js:72:12) at internal/main/run_main_module.js:17:47 { code: 'ERR_OUT_OF_RANGE' }
Example
Let us also check the alias function buffer.readBigUint64BE() method.
const buffer = Buffer.from([0x00, 0x00, 0x00, 0x00, 0xff, 0xff, 0xff, 0xff]); console.log("buffer length is ", buffer.length); console.log("Reading at big integer at offset 0:", buffer.readBigUint64BE(0));
Output
We have used the offset 0 to get the unsigned big integer.
buffer length is 8 Reading at big integer at offset 0: 4294967295n