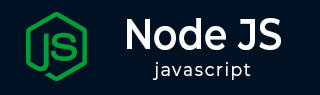
- Node.js Tutorial
- Node.js - Home
- Node.js - Introduction
- Node.js - Environment Setup
- Node.js - First Application
- Node.js - REPL Terminal
- Node.js - Command Line Options
- Node.js - Package Manager (NPM)
- Node.js - Callbacks Concept
- Node.js - Upload Files
- Node.js - Send an Email
- Node.js - Events
- Node.js - Event Loop
- Node.js - Event Emitter
- Node.js - Debugger
- Node.js - Global Objects
- Node.js - Console
- Node.js - Process
- Node.js - Scaling Application
- Node.js - Packaging
- Node.js - Express Framework
- Node.js - RESTFul API
- Node.js - Buffers
- Node.js - Streams
- Node.js - File System
- Node.js MySQL
- Node.js - MySQL Get Started
- Node.js - MySQL Create Database
- Node.js - MySQL Create Table
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB Get Started
- Node.js - MongoDB Create Database
- Node.js - MongoDB Create Collection
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB Query
- Node.js - MongoDB Sort
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js Modules
- Node.js - Modules
- Node.js - Built-in Modules
- Node.js - Utility Modules
- Node.js - Web Module
- Node.js Useful Resources
- Node.js - Quick Guide
- Node.js - Useful Resources
- Node.js - Dicussion
Node.js - Buffer.alloc() Method
The NodeJS Buffer.alloc() method helps to create a new buffer with the size given. If the size given happens to be greater than buffer.constants.MAX_LENGTH or less than 0, it will throw an error of type: ERR_INVALID_ARG_VALUE. It will throw a typeError if the size given is not a number.
Also creating a buffer using Buffer.alloc() is said to be slower than its alternative Buffer.allocUnsafe(), but it takes care of that the newly created buffer does not have any sensitive data from the previous buffers and also data that was previously allocated for buffer creation.
Syntax
Following is the syntax of the NodeJS Buffer.alloc() Method −
Buffer.alloc(size[, fill[, encoding]])
Parameters
This method accepts three parameters. The same is explained below.
size −(required) This is integer value and is the length for the buffer to be created.
fill −(optional) Here fill is the value you want the buffer to fill with.By default the value is 0.
encoding −(optional) The default encoding used is utf8. If you are using a string the encoding is required.
Return value
The method Buffer.alloc() will return the buffer of the given size.
Example
In the example will create a buffer using NodeJS Buffer.alloc() method −
const mybuffer = Buffer.alloc(5); console.log(mybuffer);
Output
The size of the buffer is 5. Since we have not used fill, the default value 0 is filled in the buffer of size 5.
<Buffer 00 00 00 00 00>
Example
In the example will create a buffer using Buffer.alloc() method and fill variable −
const mybuffer = Buffer.alloc(5, 5); console.log(mybuffer);
Output
The size of the buffer is 5. Since we have used fill as 5.
<Buffer 05 05 05 05 05>
Example
In the example will create a buffer using Buffer.alloc() method and use the fill as a string value −
const mybuffer = Buffer.alloc(5, 'a'); console.log(mybuffer); console.log(mybuffer.toString());
Output
The size of the buffer is 5. Since we have used fill is a string: 'a'.
<Buffer 61 61 61 61 61> aaaaa
Example
In this example will see the error thrown if the size used is not a number.
const mybuffer = Buffer.alloc('1'); console.log(mybuffer); console.log(mybuffer.toString());
Output
Since the size used is a string, it's an invalid value as size has to be an integer value. When you execute the above program, it will throw an error as shown below.
TypeError [ERR_INVALID_ARG_TYPE]: The "size" argument must be of type number. Received type string at Function.alloc (buffer.js:271:3) at Object.<anonymous> (C:\nodejsProject\src\testbuffer.js:1:25) at Module._compile (internal/modules/cjs/loader.js:816:30) at Object.Module._extensions..js (internal/modules/cjs/loader.js:827:10) at Module.load (internal/modules/cjs/loader.js:685:32) at Function.Module._load (internal/modules/cjs/loader.js:620:12) at Function.Module.runMain (internal/modules/cjs/loader.js:877:12) at internal/main/run_main_module.js:21:11
To Continue Learning Please Login