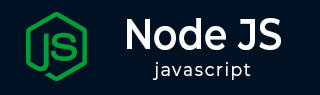
- Node.js Tutorial
- Node.js - Home
- Node.js - Introduction
- Node.js - Environment Setup
- Node.js - First Application
- Node.js - REPL Terminal
- Node.js - Command Line Options
- Node.js - Package Manager (NPM)
- Node.js - Callbacks Concept
- Node.js - Upload Files
- Node.js - Send an Email
- Node.js - Events
- Node.js - Event Loop
- Node.js - Event Emitter
- Node.js - Debugger
- Node.js - Global Objects
- Node.js - Console
- Node.js - Process
- Node.js - Scaling Application
- Node.js - Packaging
- Node.js - Express Framework
- Node.js - RESTFul API
- Node.js - Buffers
- Node.js - Streams
- Node.js - File System
- Node.js MySQL
- Node.js - MySQL Get Started
- Node.js - MySQL Create Database
- Node.js - MySQL Create Table
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB Get Started
- Node.js - MongoDB Create Database
- Node.js - MongoDB Create Collection
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB Query
- Node.js - MongoDB Sort
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js Modules
- Node.js - Modules
- Node.js - Built-in Modules
- Node.js - Utility Modules
- Node.js - Web Module
- Node.js Useful Resources
- Node.js - Quick Guide
- Node.js - Useful Resources
- Node.js - Dicussion
Node.js - Buffer.entries() Method
The NodeJS buffer.entries() method creates an iterator object from the buffer given and returns back a pair with [index, byte] from the contents of the buffer.
Syntax
Following is the syntax of the NodeJS entries() method −
buffer.entries()
Parameters
This method does not have any parameters.
Return value
The method buffer.entries() returns an iterator object that has a pair of index and byte from the contents of the buffer given.
The returned value is an iterator, so to access it you can make use of the for-of() loop. You can also access the value by using the next() method.
Example
In the Example below, the i when consoled will give you the index and Unicode byte value for the letters H, E, L, L and 0.
var buffer = Buffer.from('HELLO'); for (var i of buffer.entries()) { console.log(i); }
Output
The 0 is the index and 72 is the Unicode value for character H is 72, for E is 69 and index: 1, for L it is 76 and O it is 79.
[ 0, 72 ] [ 1, 69 ] [ 2, 76 ] [ 3, 76 ] [ 4, 79 ]
Example
In this example will access the iterator returned from buffer.entries() using next() method.
var buffer1 = Buffer.from('HELLO'); const bufferiterator = buffer1.entries(); let myitr = bufferiterator.next(); while(!myitr.done){ console.log(myitr.value); myitr = bufferiterator.next(); }
Output
An iterator is looped by continuously calling the next() method until the value of done comes to true. Having the done: true indicates that we have reached the end of the iterator.
[ 0, 72 ] [ 1, 69 ] [ 2, 76 ] [ 3, 76 ] [ 4, 79 ]
Example
We can also copy the contents of buffer1 to another buffer using Buffer.entries() method.
const buffer1 = Buffer.from("HELLO"); const buffer2 = Buffer.alloc(buffer1.length); for (const [index, bytevalue] of buffer1.entries()) { buffer2[index] = bytevalue; } console.log("The string in buffer2 is "+buffer2.toString());
Output
In the example above we have created a buffer using string: HELLO, the same length of bytes is allocated for buffer2. Later we have looped the iterator for buffer1 and updated the buffer2 with index and value as shown in the example.
The string in buffer2 is HELLO
To Continue Learning Please Login