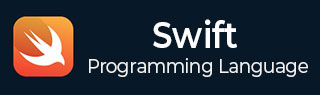
- Swift Tutorial
- Swift - Home
- Swift - Overview
- Swift - Environment
- Swift - Basic Syntax
- Swift - Variables
- Swift - Constants
- Swift - Literals
- Swift - Comments
- Swift Operators
- Swift - Operators
- Swift - Arithmetic Operators
- Swift - Comparison Operators
- Swift - Logical Operators
- Swift - Assignment Operators
- Swift - Bitwise Operators
- Swift - Misc Operators
- Swift Advanced Operators
- Swift - Operator Overloading
- Swift - Arithmetic Overflow Operators
- Swift - Identity Operators
- Swift - Range Operators
- Swift Data Types
- Swift - Data Types
- Swift - Integers
- Swift - Floating-Point Numbers
- Swift - Double
- Swift - Boolean
- Swift - Strings
- Swift - Characters
- Swift - Type Aliases
- Swift - Optionals
- Swift - Tuples
- Swift - Assertions and Precondition
- Swift Control Flow
- Swift - Decision Making
- Swift - if statement
- Swift - if...else if...else Statement
- Swift - if-else Statement
- Swift - nested if statements
- Swift - switch statement
- Swift - Loops
- Swift - for in loop
- Swift - While loop
- Swift - repeat...while loop
- Swift - continue statement
- Swift - break statement
- Swift - fall through statement
- Swift Collections
- Swift - Arrays
- Swift - Sets
- Swift - Dictionaries
- Swift Functions
- Swift - Functions
- Swift - Nested Functions
- Swift - Function Overloading
- Swift - Recursion
- Swift - Higher-Order Functions
- Swift Closures
- Swift - Closures
- Swift-Escaping and Non-escaping closure
- Swift - Auto Closures
- Swift OOps
- Swift - Enumerations
- Swift - Structures
- Swift - Classes
- Swift - Properties
- Swift - Methods
- Swift - Subscripts
- Swift - Inheritance
- Swift-Overriding
- Swift - Initialization
- Swift - Deinitialization
- Swift Advanced
- Swift - ARC Overview
- Swift - Optional Chaining
- Swift - Error handling
- Swift - Concurrency
- Swift - Type Casting
- Swift - Nested Types
- Swift - Extensions
- Swift - Protocols
- Swift - Generics
- Swift - Access Control
- Swift - Function vs Method
- Swift - SwiftyJSON
- Swift - Singleton class
- Swift Random Numbers
- Swift Opaque and Boxed Type
- Swift Useful Resources
- Swift - Compile Online
- Swift - Quick Guide
- Swift - Useful Resources
- Swift - Discussion
Swift - Recursion
Recursion in Swift
Recursion is a technique in which a function calls itself directly or indirectly to solve a problem. Instead of solving the entire problem at once it divides the problem into smaller sub-problems and then solves them by calling itself repeatedly until it reaches to the base condition. A recursive function has two main components −
Base condition − The base condition is responsible for stopping the recursive call. Or it is a termination point where it prevents the function from calling itself infinitely. If we do not specify the base condition in the recursive function, then it will call itself infinitely and the program will never end.
Recursive call − Recursive call is where the function calls itself with modified parameters to solve the task. With iteration, the recursive call should move towards the base condition so that it terminates successfully without entering into infinite recursion.
Syntax
Following is the syntax of the recursive function −
func functionName(){ // body functionName() } functionName()
Working of Recursion in Swift
If we call a function within itself, it is known as a recursive function. Swift supports recursion in functions. Let's understand the working of the recursion with the help of an example.
Example
Swift program to find the factorial of the given number using recursion −
import Foundation // Function to find the factorial of the specified number func factorial(number: Int) -> Int { // Base condition if number == 0 || number == 1 { return 1 } else { // Recursive call with modified parameters return number * factorial(number:number - 1) } } let num = 4 let output = factorial(number: num) print("Factorial of \(num) is: \(output)")
Output
It will produce the following output −
Factorial of 4 is: 24
In the above code, we have a recursive function named factorial(). So the working of this function is −
1st function call with 4: factorial(4) = 4 * factorial(3) 2nd function call with 3: factorial(3) = 3 * factorial(2) 3rd function call with 2: factorial(2) = 2 * factorial(1) 4th function call with 1: factorial(1) = 1(Here the value meets the base condition and the recursive call terminated) Returned from 4th function call: 1 * 1 = 1 Returned from 3rd function call: 2 * 1 = 2 Returned from 2nd function call: 3 * 2 = 6 Returned from 1st function call: 4 * 6 = 24 Hence the factorial of 4 is 24.
Example
Swift program to find the index of the specified element using binary search −
import Foundation // Function to find a number from the given array using binary search func binarySearchAlgorithm(_ arr: [Int], num: Int, leftNum: Int, rightNum: Int) -> Int? { if leftNum > rightNum { return nil } let midValue = leftNum + (rightNum - leftNum) / 2 if arr[midValue] == num { return midValue } else if arr[midValue] < num { return binarySearchAlgorithm(arr, num: num, leftNum: midValue + 1, rightNum: rightNum) } else { return binarySearchAlgorithm(arr, num: num, leftNum: leftNum, rightNum: midValue - 1) } } let myArray = [11, 12, 13, 14, 15, 16, 17, 18, 19] if let resIndex = binarySearchAlgorithm(myArray, num: 16, leftNum: 0, rightNum: myArray.count - 1) { print("Element found at index \(resIndex)") } else { print("Element Not found") }
Output
It will produce the following output −
Element found at index 5
Need for Recursion in Swift
Recursion is a compelling technique for solving larger programming problems very easily. We can use recursion in the following scenarios −
Divide and Conquer − Recursive algorithms use divide and conquer approaches, they divide the complex problems into small identical sub-problems and solve them in each recursive call.
Tree and Graph Traversal − Recursive functions are generally used to traverse trees and graphs in data structures.
Code Readability − Recursion results are much more readable and manageable as compared to iterative approaches.
Mathematical calculations − Using recursion we can solve various mathematical problems like factorial, Fibonacci numbers, etc.
Natural Representation − Recursion gives a natural and intuitive representation of the problems that exhibit the recursive properties.
Advantages and Disadvantages of Recursion
The following are the advantages of recursion −
Recursive functions are easy to debug because each recursive call focuses on a small part of the task which allows more target testing and debugging.
Recursive functions generally use parallel processing which improves their performance.
Recursive solutions are much more readable and easy to maintain.
Recursive functions are flexible and allow abstraction.
The following are the disadvantages of Recursion −
While working with a more complex problem, the execution flow of the recursion is difficult to track.
It has more overhead than iterative loops.
If we do not provide the correct base condition, it will lead to infinite recursion.
Recursion is not best suitable for all types of problems some problems are better to solve with the iteration method.