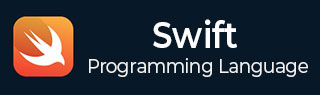
- Swift Tutorial
- Swift - Home
- Swift - Overview
- Swift - Environment
- Swift - Basic Syntax
- Swift - Variables
- Swift - Constants
- Swift - Literals
- Swift - Comments
- Swift Operators
- Swift - Operators
- Swift - Arithmetic Operators
- Swift - Comparison Operators
- Swift - Logical Operators
- Swift - Assignment Operators
- Swift - Bitwise Operators
- Swift - Misc Operators
- Swift Advanced Operators
- Swift - Operator Overloading
- Swift - Arithmetic Overflow Operators
- Swift - Identity Operators
- Swift - Range Operators
- Swift Data Types
- Swift - Data Types
- Swift - Integers
- Swift - Floating-Point Numbers
- Swift - Double
- Swift - Boolean
- Swift - Strings
- Swift - Characters
- Swift - Type Aliases
- Swift - Optionals
- Swift - Tuples
- Swift - Assertions and Precondition
- Swift Control Flow
- Swift - Decision Making
- Swift - if statement
- Swift - if...else if...else Statement
- Swift - if-else Statement
- Swift - nested if statements
- Swift - switch statement
- Swift - Loops
- Swift - for in loop
- Swift - While loop
- Swift - repeat...while loop
- Swift - continue statement
- Swift - break statement
- Swift - fall through statement
- Swift Collections
- Swift - Arrays
- Swift - Sets
- Swift - Dictionaries
- Swift Functions
- Swift - Functions
- Swift - Nested Functions
- Swift - Function Overloading
- Swift - Recursion
- Swift - Higher-Order Functions
- Swift Closures
- Swift - Closures
- Swift-Escaping and Non-escaping closure
- Swift - Auto Closures
- Swift OOps
- Swift - Enumerations
- Swift - Structures
- Swift - Classes
- Swift - Properties
- Swift - Methods
- Swift - Subscripts
- Swift - Inheritance
- Swift-Overriding
- Swift - Initialization
- Swift - Deinitialization
- Swift Advanced
- Swift - ARC Overview
- Swift - Optional Chaining
- Swift - Error handling
- Swift - Concurrency
- Swift - Type Casting
- Swift - Nested Types
- Swift - Extensions
- Swift - Protocols
- Swift - Generics
- Swift - Access Control
- Swift - Function vs Method
- Swift - SwiftyJSON
- Swift - Singleton class
- Swift Random Numbers
- Swift Opaque and Boxed Type
- Swift Useful Resources
- Swift - Compile Online
- Swift - Quick Guide
- Swift - Useful Resources
- Swift - Discussion
Swift - Integers
Integers data type is used to store whole numbers such as 23, -543, 0, 332, etc. It can store positive, negative and zero numbers. It does not store fractional numbers like 3.43, 4.423, etc. Swift divides integers into two categories −
Signed Integers − Signed integers are used to store zero and positive integers. They are available in 8, 16, 23, and 64-bit forms. They are represented by Int like an 8-bit signed integer of type Int8.
Unsigned Integers − Unsigned integers are used to store negative integers. They are also available in 8, 16, 23, and 64-bit forms. It is represented by Uint like a 32-bit unsigned integer of type UInt32.
Int
Swift provides a special integer type named Int. Using Int type we do not need to explicitly specify the size of the integer. The size of Int is the same as the size of the platform such as if we have a 32-bit platform then the size of Int is Int32 and if we have a 64-bit platform then the size of the Int is Int64. It can store values between -2, 147, 483, 648 and 2, 147, 483, 647.
Syntax
Following is the syntax of the Int −
var value : Int
Example
Swift program to calculate the sum of two integers.
import Foundation // Defining integer data type let num1 : Int = 232 let num2 : Int = 31 // Store the sum var sum = 0 // Calculate the sum sum = num1 + num2 print("Sum of \(num1) and \(num2) = \(sum)")
Output
Sum of 232 and 31 = 263
UInt
Using UInt we can also store unsigned integer data types without explicitly specifying their size. The size of Uint is also the same as the size of the platform such as if we have a 32-bit platform, then the size is UInt32 whereas if we have a 64-bit platform, then the size is UInt64.
Syntax
Following is the syntax of UInt −
var num : Uint = 32
Example
Swift program to add two unsigned integers.
import Foundation // Defining Unsigned integer data type let num1 : UInt = 32 let num2 : UInt = 22 // Store the sum var sum : UInt // Calculate the sum sum = num1 + num2 print("Sum of \(num1) and \(num2) = \(sum)")
Output
Sum of 32 and 22 = 54
Integer Bounds
The minimum and maximum size of the integer data type are as follows −
Type | Size | Range |
---|---|---|
Int8 | 1 bytes | -128 to 127 |
Int16 | 2 bytes | -32768 to 32767 |
Int32 | 4 bytes | -2147483648 to 2147483647 |
Int64 | 8 bytes | -9223372036854775808 to 9223372036854775807 |
UInt8 | 1 bytes | 0 to 255 |
UInt16 | 2 bytes | 0 to 65535 |
UInt32 | 4 bytes | 0 to 4294967295 |
UInt64 | 8 bytes | 0 to 18446744073709551615 |
Minimum and Maximum size of Integer
We can explicitly calculate the size of the integer with the help of the pre-defined properties of Swift min and max.
The min property is used to calculate the minimum size of the integer and the max property is used to calculate the maximum size of the integer.
Example
Swift program to calculate the minimum size of the Int8 and UInt16.
import Foundation // Minimum size of Int8 and UInt16 let result1 = Int8.min let result2 = UInt16.min print("Minimum Size of Int8 is \(result1)") print("Minimum Size of UInt16 is \(result2)")
Output
Minimum Size of Int8 is -128 Minimum Size of UInt16 is 0
Example
Swift program to calculate the maximum size of the Int16 and UInt64.
import Foundation // Maximum size of Int16 and UInt64 let result1 = Int16.max let result2 = UInt64.max print("Maximum Size of Int16 is \(result1)") print("Maximum Size of UInt64 is \(result2)")
Output
Maximum Size of Int16 is 32767 Maximum Size of UInt64 is 18446744073709551615