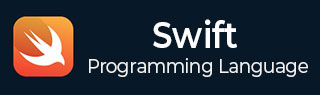
- Swift Tutorial
- Swift - Home
- Swift - Overview
- Swift - Environment
- Swift - Basic Syntax
- Swift - Variables
- Swift - Constants
- Swift - Literals
- Swift - Comments
- Swift Operators
- Swift - Operators
- Swift - Arithmetic Operators
- Swift - Comparison Operators
- Swift - Logical Operators
- Swift - Assignment Operators
- Swift - Bitwise Operators
- Swift - Misc Operators
- Swift Advanced Operators
- Swift - Operator Overloading
- Swift - Arithmetic Overflow Operators
- Swift - Identity Operators
- Swift - Range Operators
- Swift Data Types
- Swift - Data Types
- Swift - Integers
- Swift - Floating-Point Numbers
- Swift - Double
- Swift - Boolean
- Swift - Strings
- Swift - Characters
- Swift - Type Aliases
- Swift - Optionals
- Swift - Tuples
- Swift - Assertions and Precondition
- Swift Control Flow
- Swift - Decision Making
- Swift - if statement
- Swift - if...else if...else Statement
- Swift - if-else Statement
- Swift - nested if statements
- Swift - switch statement
- Swift - Loops
- Swift - for in loop
- Swift - While loop
- Swift - repeat...while loop
- Swift - continue statement
- Swift - break statement
- Swift - fall through statement
- Swift Collections
- Swift - Arrays
- Swift - Sets
- Swift - Dictionaries
- Swift Functions
- Swift - Functions
- Swift - Nested Functions
- Swift - Function Overloading
- Swift - Recursion
- Swift - Higher-Order Functions
- Swift Closures
- Swift - Closures
- Swift-Escaping and Non-escaping closure
- Swift - Auto Closures
- Swift OOps
- Swift - Enumerations
- Swift - Structures
- Swift - Classes
- Swift - Properties
- Swift - Methods
- Swift - Subscripts
- Swift - Inheritance
- Swift-Overriding
- Swift - Initialization
- Swift - Deinitialization
- Swift Advanced
- Swift - ARC Overview
- Swift - Optional Chaining
- Swift - Error handling
- Swift - Concurrency
- Swift - Type Casting
- Swift - Nested Types
- Swift - Extensions
- Swift - Protocols
- Swift - Generics
- Swift - Access Control
- Swift - Function vs Method
- Swift - SwiftyJSON
- Swift - Singleton class
- Swift Random Numbers
- Swift Opaque and Boxed Type
- Swift Useful Resources
- Swift - Compile Online
- Swift - Quick Guide
- Swift - Useful Resources
- Swift - Discussion
Swift-Escaping and Non-escaping closure
Swift Closure
Just like another programming language Swift also supports Closure. A closure is a self-contained block of functionality that can passed around and used inside the code to perform any specific task. It can assigned to a variable or can be passed as a parameter to a function. A closure can capture values from its surrounding context and can be used as a callback or inline code. Swift supports the following type of closure −
- Escaping Closure
- Non-Escaping Closure
Let us discuss both of them in detail.
Escaping Closures in Swift
When a closure is passed as an argument to the function but that closure is called after the function returns, then such type of closure is known as escaping closure. By default, the closure passed in the function as a parameter is a non-escaping parameter, which means the closure will execute during the execution of the function.
To declare a closure as an escaping closure we have to use the @escaping keyword before the parameter type that represents the escaping closure.
Syntax
Following is the syntax for escaping closure −
func methodname(closure: @escaping() -> Void){ // body // Calling the closure closure() }
Example
Swift program to demonstrate escaping closure.
import Foundation class Operation{ // Method that takes two numbers and an escaping closure to find their product func product(_ x: Int, _ y: Int, productResult: @escaping (Int) -> Void) { // Activating an asynchronous task DispatchQueue.global().async { let output = x * y // Calling escaping closure productResult(output) } } } // Creating object of Operation class let obj = Operation() // Accessing the method obj.product(10, 4) { res in print("Product of 10 * 4 = \(res)") } // Activating the passage of time DispatchQueue.main.asyncAfter(deadline: .now() + 2) { // It will be executed after a delay, simulating an asynchronous operation }
Output
It will produce the following output −
Product of 10 * 4 = 40
Non-Escaping Closure in Swift
Non-escaping closures are the default in Swift, we do not require any special notation to represent non-escaping closures. Such type of closure executes during the execution of the function in which they are passed, once the execution of the function ends the closure is no longer available for execution. They are not stored for later execution.
Syntax
Following is the syntax of non-escaping closure −
mutating func methodname(Parameters) -> returntype { Statement }
Example
Swift program to demonstrate non-escaping closure.
class Operation { // Function that takes two numbers and a non-escaping closure to calculate their sum func sum(_ X: Int, _ Y: Int, add: (Int) -> Void) { let result = X + Y // Calling the non-escaping closure add(result) } } // Creating the object of operation class let obj = Operation() obj.sum(10, 12) { add in print("sum: \(add)") }
Output
It will produce the following output −
Sum: 22
Escaping VS Non-Escaping Closure
Following are the major differences between the escaping and non-escaping closures −
Escaping Closure | Non-Escaping Closure |
---|---|
It can outlive the function in which it is passed. | It executes during the execution of the function. |
It can store as a property or can assigned to a variable. | It cannot stored and not allowed to used outside the function’s scope. |
It is commonly used for asynchronous operations like network request, etc. | It is commonly used for synchronous operations like simple computation, etc. |
@escaping keyword is used to created escaping clousre. | It is by default closure and doesnot required any special syntax. |