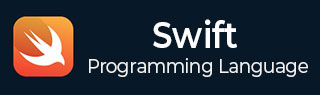
- Swift Tutorial
- Swift - Home
- Swift - Overview
- Swift - Environment
- Swift - Basic Syntax
- Swift - Variables
- Swift - Constants
- Swift - Literals
- Swift - Comments
- Swift Operators
- Swift - Operators
- Swift - Arithmetic Operators
- Swift - Comparison Operators
- Swift - Logical Operators
- Swift - Assignment Operators
- Swift - Bitwise Operators
- Swift - Misc Operators
- Swift Advanced Operators
- Swift - Operator Overloading
- Swift - Arithmetic Overflow Operators
- Swift - Identity Operators
- Swift - Range Operators
- Swift Data Types
- Swift - Data Types
- Swift - Integers
- Swift - Floating-Point Numbers
- Swift - Double
- Swift - Boolean
- Swift - Strings
- Swift - Characters
- Swift - Type Aliases
- Swift - Optionals
- Swift - Tuples
- Swift - Assertions and Precondition
- Swift Control Flow
- Swift - Decision Making
- Swift - if statement
- Swift - if...else if...else Statement
- Swift - if-else Statement
- Swift - nested if statements
- Swift - switch statement
- Swift - Loops
- Swift - for in loop
- Swift - While loop
- Swift - repeat...while loop
- Swift - continue statement
- Swift - break statement
- Swift - fall through statement
- Swift Collections
- Swift - Arrays
- Swift - Sets
- Swift - Dictionaries
- Swift Functions
- Swift - Functions
- Swift - Nested Functions
- Swift - Function Overloading
- Swift - Recursion
- Swift - Higher-Order Functions
- Swift Closures
- Swift - Closures
- Swift-Escaping and Non-escaping closure
- Swift - Auto Closures
- Swift OOps
- Swift - Enumerations
- Swift - Structures
- Swift - Classes
- Swift - Properties
- Swift - Methods
- Swift - Subscripts
- Swift - Inheritance
- Swift-Overriding
- Swift - Initialization
- Swift - Deinitialization
- Swift Advanced
- Swift - ARC Overview
- Swift - Optional Chaining
- Swift - Error handling
- Swift - Concurrency
- Swift - Type Casting
- Swift - Nested Types
- Swift - Extensions
- Swift - Protocols
- Swift - Generics
- Swift - Access Control
- Swift - Function vs Method
- Swift - SwiftyJSON
- Swift - Singleton class
- Swift Random Numbers
- Swift Opaque and Boxed Type
- Swift Useful Resources
- Swift - Compile Online
- Swift - Quick Guide
- Swift - Useful Resources
- Swift - Discussion
Swift - Misc Operators
Miscellaneous Operators Swift
Swift supports different types of operators like arithmetic, comparison, logical, bitwise, assignment and range operators. Apart from these operators it has miscellaneous operators are they are −
Operator | Name | Example |
---|---|---|
- | Unary Minus | -23 |
+ | Unary Plus | 32 |
Condition ? X : Y | Ternary operator | X>Y ? 43 : 21= 43 |
Unary Minus Operator in Swift
A unary minus operator is used to represent a negative(-) sign that is placed before the numeric value. It converts a positive number into a negative and a negative number into a positive. It is a prefix operator, which means it is placed before the value without any white space.
Syntax
Following is the syntax of the unary minus operator −
-x
Example
Swift program to find the sum of two numbers using the unary minus operator.
import Foundation let x = 23 // Specifying sign using unary minus operator let y = -2 var sum = 0 sum = x + y // 23 + (-2) print("Sum of \(x) and \(y) = \(sum)")
Output
Sum of 23 and -2 = 21
Unary Plus Operator in Swift
A unary plus operator is used to make a numeric expression positive. It only adds a positive (+) sign before the numeric value but does not change the value. It is also a prefix operator.
Syntax
Following is the syntax of the unary plus operator −
+x
Example
Swift program to find the sum of two numbers using the unary plus operator.
import Foundation let x = 20 // Specifying sign using unary plus operator let y = +2 var sum = 0 sum = x + y // 23 + (+2) print("Sum of \(x) and \(y) = \(sum)")
Output
Sum of 20 and 2 = 22
Ternary Conditional Operator in Swift
A ternary conditional operator is a shorthand of an if-else statement. It has three parts: condition ? expression1 : espression2. It is the most effective way to decide between two expressions.
Because it evaluates one of the two given expressions according to whether the given condition is true or false. If the given condition is true, then it evaluates expression1. Otherwise, it will evaluate expression2.
Syntax
Following is the syntax of the ternary conditional operator −
Condition ? Expression1 : Expression2
Example
Swift program to explain ternary conditional operator.
import Foundation let x = 20 let y = 2 // If x is greater than y then it will return 34 // Otherwise return 56 var result = x > y ? 34 : 56 print(result)
Output
34