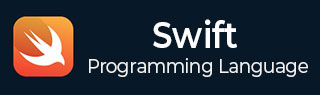
- Swift Tutorial
- Swift - Home
- Swift - Overview
- Swift - Environment
- Swift - Basic Syntax
- Swift - Variables
- Swift - Constants
- Swift - Literals
- Swift - Comments
- Swift Operators
- Swift - Operators
- Swift - Arithmetic Operators
- Swift - Comparison Operators
- Swift - Logical Operators
- Swift - Assignment Operators
- Swift - Bitwise Operators
- Swift - Misc Operators
- Swift Advanced Operators
- Swift - Operator Overloading
- Swift - Arithmetic Overflow Operators
- Swift - Identity Operators
- Swift - Range Operators
- Swift Data Types
- Swift - Data Types
- Swift - Integers
- Swift - Floating-Point Numbers
- Swift - Double
- Swift - Boolean
- Swift - Strings
- Swift - Characters
- Swift - Type Aliases
- Swift - Optionals
- Swift - Tuples
- Swift - Assertions and Precondition
- Swift Control Flow
- Swift - Decision Making
- Swift - if statement
- Swift - if...else if...else Statement
- Swift - if-else Statement
- Swift - nested if statements
- Swift - switch statement
- Swift - Loops
- Swift - for in loop
- Swift - While loop
- Swift - repeat...while loop
- Swift - continue statement
- Swift - break statement
- Swift - fall through statement
- Swift Collections
- Swift - Arrays
- Swift - Sets
- Swift - Dictionaries
- Swift Functions
- Swift - Functions
- Swift - Nested Functions
- Swift - Function Overloading
- Swift - Recursion
- Swift - Higher-Order Functions
- Swift Closures
- Swift - Closures
- Swift-Escaping and Non-escaping closure
- Swift - Auto Closures
- Swift OOps
- Swift - Enumerations
- Swift - Structures
- Swift - Classes
- Swift - Properties
- Swift - Methods
- Swift - Subscripts
- Swift - Inheritance
- Swift-Overriding
- Swift - Initialization
- Swift - Deinitialization
- Swift Advanced
- Swift - ARC Overview
- Swift - Optional Chaining
- Swift - Error handling
- Swift - Concurrency
- Swift - Type Casting
- Swift - Nested Types
- Swift - Extensions
- Swift - Protocols
- Swift - Generics
- Swift - Access Control
- Swift - Function vs Method
- Swift - SwiftyJSON
- Swift - Singleton class
- Swift Random Numbers
- Swift Opaque and Boxed Type
- Swift Useful Resources
- Swift - Compile Online
- Swift - Quick Guide
- Swift - Useful Resources
- Swift - Discussion
Swift - Decision Making
What is Decision Making Structure?
Decision-making structures require that the programmer specifies one or more conditions to be evaluated or tested by the program, along with a statement or statements to be executed if the condition is determined to be true, and optionally, other statements to be executed if the condition is determined to be false. Using decision-making structure programmers can able to create flexible and responsive programs, which allows the program to take different paths according to the condition.
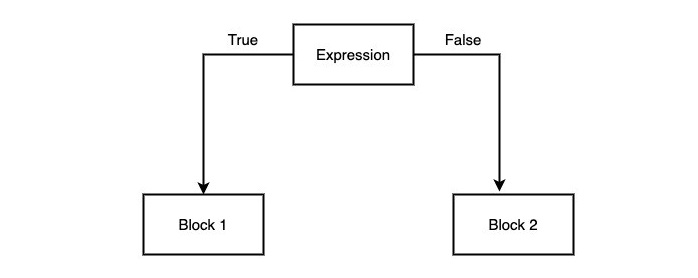
Type of Decision-Making Statement in Swift
Swift provides the following types of decision-making statements.
Statement | Description |
---|---|
if statement | An if statement allows you to execute a block of code if the given expression is true. |
if…else statement | The if-else statement allows you to execute a block of code when the if condition is true. Otherwise else block will execute. |
if…else if…else statements | The if…else if…else statements are used to check multiple conditions in sequence and execute the block of statement associated to the first true condition. |
Nested if statement | Nested if statement is used to specify statement inside another statement. |
Switch statement | A switch statement allows a variable to be tested for equality against a list of values. |
Example
Swift program to demonstrate the use of switch statements.
import Foundation var index = 10 switch index { case 100 : print( "Value of index is 100") case 10,15 : print( "Value of index is either 10 or 15") case 5 : print( "Value of index is 5") default : print( "default case") }
Output
It will produce the following output −
Value of index is either 10 or 15
Example
Swift program to demonstrate the use of if-else statements.
import Foundation let X = 40 let Y = 80 // Comparing two numbers if X > Y { print("X is greater than Y.") } else { print("Y is greater than or equal to X.") }
Output
It will produce the following output −
Y is greater than or equal to X.
Swift Ternary Conditional Operator
The ternary conditional operator is the shorthand representation of an if-else statement. It has three parts condition, result1 and result2. If the condition is true then it will execute the result1 and return its value. Otherwise, it will execute result2 and return its value.
Syntax
Following is the syntax of the ternary conditional Operator −
Exp1 ? Exp2 : Exp3
Where Exp1, Exp2, and Exp3 are expressions. The value of a ? expression is determined like this: Exp1 is evaluated. If it is true, then Exp2 is evaluated and becomes the value of the entire ? expression. If Exp1 is false, then Exp3 is evaluated and its value becomes the value of the expression.
Example
Swift program to check whether the number is odd or even using ternary conditional operator.
import Foundation let num = 34 // Checking whether the given number is odd or even // Using ternary conditional operator let result = num % 2 == 0 ? "Even" : "Odd" print("The number is \(result).")
Output
It will produce the following output −
The number is Even.
Example
Swift program to check the number is positive or negative using ternary conditional operator.
import Foundation let num = 34 // Checking whether the given number is positive or negative // Using ternary conditional operator let result = num >= 0 ? "Positive" : "Negative" print("Given number is \(result).")
Output
It will produce the following output −
Given number is Positive.