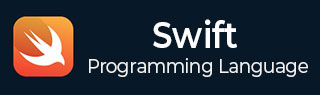
- Swift Tutorial
- Swift - Home
- Swift - Overview
- Swift - Environment
- Swift - Basic Syntax
- Swift - Variables
- Swift - Constants
- Swift - Literals
- Swift - Comments
- Swift Operators
- Swift - Operators
- Swift - Arithmetic Operators
- Swift - Comparison Operators
- Swift - Logical Operators
- Swift - Assignment Operators
- Swift - Bitwise Operators
- Swift - Misc Operators
- Swift Advanced Operators
- Swift - Operator Overloading
- Swift - Arithmetic Overflow Operators
- Swift - Identity Operators
- Swift - Range Operators
- Swift Data Types
- Swift - Data Types
- Swift - Integers
- Swift - Floating-Point Numbers
- Swift - Double
- Swift - Boolean
- Swift - Strings
- Swift - Characters
- Swift - Type Aliases
- Swift - Optionals
- Swift - Tuples
- Swift - Assertions and Precondition
- Swift Control Flow
- Swift - Decision Making
- Swift - if statement
- Swift - if...else if...else Statement
- Swift - if-else Statement
- Swift - nested if statements
- Swift - switch statement
- Swift - Loops
- Swift - for in loop
- Swift - While loop
- Swift - repeat...while loop
- Swift - continue statement
- Swift - break statement
- Swift - fall through statement
- Swift Collections
- Swift - Arrays
- Swift - Sets
- Swift - Dictionaries
- Swift Functions
- Swift - Functions
- Swift - Nested Functions
- Swift - Function Overloading
- Swift - Recursion
- Swift - Higher-Order Functions
- Swift Closures
- Swift - Closures
- Swift-Escaping and Non-escaping closure
- Swift - Auto Closures
- Swift OOps
- Swift - Enumerations
- Swift - Structures
- Swift - Classes
- Swift - Properties
- Swift - Methods
- Swift - Subscripts
- Swift - Inheritance
- Swift-Overriding
- Swift - Initialization
- Swift - Deinitialization
- Swift Advanced
- Swift - ARC Overview
- Swift - Optional Chaining
- Swift - Error handling
- Swift - Concurrency
- Swift - Type Casting
- Swift - Nested Types
- Swift - Extensions
- Swift - Protocols
- Swift - Generics
- Swift - Access Control
- Swift - Function vs Method
- Swift - SwiftyJSON
- Swift - Singleton class
- Swift Random Numbers
- Swift Opaque and Boxed Type
- Swift Useful Resources
- Swift - Compile Online
- Swift - Quick Guide
- Swift - Useful Resources
- Swift - Discussion
Swift - Optionals
Swift introduced a new feature named Optional type. The optional type handles the value that may be absent or undefined. It allows variables to represent a value or nil. The Optional are similar to using nil with pointers in Objective-C, but they work for any type, not just classes. To specify the optional type we have to use question marks(?) after the name of the type.
Syntax
Following is the syntax for the optional variable −
var perhapsInt: Int?
Example
Swift program to demonstrate how to create optional variable.
// Declaring an optional Integer var number: Int? // Assigning a value to the optional number = 34 // Using optional binding to safely unwrap its value if let x = number { print("Value is \(x)!") } else { print("Value is unknown") }
Output
Value is 34!
Optionals with nil
If we want to specify an optional variable to a valueless state or no value, then we can assign nil to that variable. Or if we do not assign any value to an optional variable, then Swift will automatically set the value of that variable to nil. In Swift, we are only allowed to assign nil to an optional variable, if we try to assign nil to a non-optional variable or constant, then we will get an error.
Syntax
Following is the syntax for assigning optional to nil −
var perhapsStr: Int? = nil
Example
Swift program to demonstrate how to assign nil to an optional variable.
// Optional variable var num: Int? = 42 // Setting variable to nil num = nil // Check if the optional has a value or not if num != nil { print("Optional has a value: \(num!)") } else { print("Optional is nil.") }
Output
Optional is nil.
Forced Unwrapping
If you defined a variable as optional, then to get the value from this variable, you will have to unwrap it. So to unwarp the value we have to put an exclamation mark(!) at the end of the variable. It tells the compiler that you are sure that the optional contains some value.
Syntax
Following is the syntax for forced unwrapping −
let variableName = optionalValue!
Example
Swift program to demonstrate how to unwrap optional value.
// Declaring an optional Integer var number: Int? // Assigning a value to the optional number = 34 // Using exclamation mark (!) to forcefully unwrapping the optional let value: Int = number! // Displaying the unwrapped value print(value)
Output
34
Implicitly Unwrapping Optional
You can declare optional variables using an exclamation mark instead of a question mark. Such optional variables will unwrap automatically and you do not need to use any further exclamation mark at the end of the variable to get the assigned value.
Example
import Cocoa var myString:String! myString = "Hello, Swift 4!" if myString != nil { print(myString) }else{ print("myString has nil value") }
Output
Hello, Swift 4!
Optional Binding
Use optional binding to find out whether an optional contains a value, and if so, to make that value available as a temporary constant or variable. Or we can say that optional binding allows us to unwarp and work with the value present in the optional. It prevents us from unwrapping nil values and runtime crashes.
We can perform optional binding using if let, guard, and while statements. We are allowed to use multiple optional binding and boolean conditions in a single if statement, separated by a comma. If any of the optional binding or boolean conditions among the given multiple optional bindings and boolean conditions are nil, then the whole if statement is considered to be false.
Syntax
Following is the syntax for the optional binding −
if let constantName = someOptional, let variableName = someOptional { statements }
Example
// Declaring an optional Integer var number: Int? // Assigning a value to the optional number = 50 // Using optional binding to safely unwrap the optional if let x = number { print("Number is \(x)!") } else { print("Number is unknown") }
Output
Number is 50!
Nil-Coalescing Operator(??)
In Swift, we can also handle missing values and provide a default value if the optional is nil using the nil-coalescing operator(??). It is the shorthand method of using optional binding along with default value.
Syntax
Following is the syntax for the nil-coalescing operator −
Let output = optionalValue ?? defaultValue
The nil-coalescing operator (??) operator checks if the value on the left hand-side operand is not nil. If it is not nil, then it unwraps the value. If the value is nil, then it will provide the value (or as a fallback value) present in the right hand-side operand.
Example
// Function to fetch employee salary func getEmpSalary() -> Int? { // Return nil when salary is not available return nil } // Using the nil-coalescing operator to provide a valid salary let salary = getEmpSalary() ?? 5000 // Displaying the result print("Salary: \(salary)")
Output
Salary: 5000