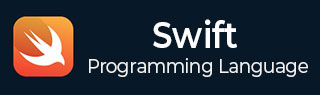
- Swift Tutorial
- Swift - Home
- Swift - Overview
- Swift - Environment
- Swift - Basic Syntax
- Swift - Variables
- Swift - Constants
- Swift - Literals
- Swift - Comments
- Swift Operators
- Swift - Operators
- Swift - Arithmetic Operators
- Swift - Comparison Operators
- Swift - Logical Operators
- Swift - Assignment Operators
- Swift - Bitwise Operators
- Swift - Misc Operators
- Swift Advanced Operators
- Swift - Operator Overloading
- Swift - Arithmetic Overflow Operators
- Swift - Identity Operators
- Swift - Range Operators
- Swift Data Types
- Swift - Data Types
- Swift - Integers
- Swift - Floating-Point Numbers
- Swift - Double
- Swift - Boolean
- Swift - Strings
- Swift - Characters
- Swift - Type Aliases
- Swift - Optionals
- Swift - Tuples
- Swift - Assertions and Precondition
- Swift Control Flow
- Swift - Decision Making
- Swift - if statement
- Swift - if...else if...else Statement
- Swift - if-else Statement
- Swift - nested if statements
- Swift - switch statement
- Swift - Loops
- Swift - for in loop
- Swift - While loop
- Swift - repeat...while loop
- Swift - continue statement
- Swift - break statement
- Swift - fall through statement
- Swift Collections
- Swift - Arrays
- Swift - Sets
- Swift - Dictionaries
- Swift Functions
- Swift - Functions
- Swift - Nested Functions
- Swift - Function Overloading
- Swift - Recursion
- Swift - Higher-Order Functions
- Swift Closures
- Swift - Closures
- Swift-Escaping and Non-escaping closure
- Swift - Auto Closures
- Swift OOps
- Swift - Enumerations
- Swift - Structures
- Swift - Classes
- Swift - Properties
- Swift - Methods
- Swift - Subscripts
- Swift - Inheritance
- Swift-Overriding
- Swift - Initialization
- Swift - Deinitialization
- Swift Advanced
- Swift - ARC Overview
- Swift - Optional Chaining
- Swift - Error handling
- Swift - Concurrency
- Swift - Type Casting
- Swift - Nested Types
- Swift - Extensions
- Swift - Protocols
- Swift - Generics
- Swift - Access Control
- Swift - Function vs Method
- Swift - SwiftyJSON
- Swift - Singleton class
- Swift Random Numbers
- Swift Opaque and Boxed Type
- Swift Useful Resources
- Swift - Compile Online
- Swift - Quick Guide
- Swift - Useful Resources
- Swift - Discussion
Swift - Nested Functions
A Function is a block of statements that is called to perform a specific task. When a function is defined inside another function, such type of function is known as a nested function. The scope of the nested function is limited to the function in which they are defined means they are only accessible from within the outer function. If we try to access them outside the outer function, then we will get the error.
In Swift, a single function can contain multiple nested functions and they must be defined before they are called.
The nested function increases the readability and organizes complex tasks by breaking them into smaller and reusable components.
Nested functions encapsulate the logic inside the limited scope and can prevent external access.
They can only access variables from their outer function.
Syntax of Nested Functions
Following is the syntax of the Nested Function −
func outerFunction(){ func nestedFunction(){ // code } func nestedFunction2(){ //code } nestedFunction1() nestedFunction2() }
Nested Function Without Parameter in Swift
In Swift, we can define a nested function without any parameters. Such a type of function does not take any parameter while calling them and may or may not return any value.
Syntax
Following is the syntax of the nested function without a parameter list −
func nestedFunction(){ // Code }
Example
Swift program to display the World Cup winner's name using nested function.
import Foundation // Outer function func worldCupTrophy(){ // Nested function without parameter list func winnerName() { print("Winner of World Cup is INDIA") } // Calling nested function winnerName() } // Calling the outer function to display the result worldCupTrophy()
Output
It will produce the following output −
Winner of World Cup is INDIA
Nested Function with Parameter in Swift
A nested function can contain a parameter list without any return type. Such types of nested functions can only access variables and parameters of the outer function. A nested function can contain multiple parameters and they are separated by their argument labels.
Syntax
Following is the syntax of Nested Function with Parameters −
func nestedFunction(name1: Int, name2: Int){ // code }
Example
Swift program to add two strings using nested function.
import Foundation // Outer function func concatenateStrings(str1: String, str2: String){ // Nested function with parameter list func displayResult(finalStr: String) { print("Concatenated String: \(finalStr)") } let result = str1 + str2 // Calling nested function displayResult(finalStr: result) } // Input strings var string1 = "Welcome " var string2 = "TutorialsPoint" // Calling the outer function to display the result concatenateStrings(str1: string1, str2: string2)
Output
It will produce the following output −
Concatenated String: Welcome TutorialsPoint
Nested Function without Return Type in Swift
A nested function without any return type does not return any value. It may or may not contain parameters.
Syntax
Following is the syntax of Nested Function without return type −
func nestedFunction(name: Int){ // code return }
Example
Swift program to calculate the area of a rectangle using nested function.
import Foundation // Outer function func Rectangle(length: Int, width: Int){ // Nested function without return type func displayArea(finalArea: Int) { print("Area of Rectangle: \(finalArea)") } let result = length * width // Calling nested function displayArea(finalArea: result) } // Input strings var l = 10 var w = 12 // Calling the outer function to display the result Rectangle(length: l, width: w)
Output
It will produce the following output −
Area of Rectangle: 120
Nested Function with Return Type in Swift
A nested function can contain a parameter list without any return type. A nested function with a return type always returns something.
Syntax
Following is the syntax of Nested Function with Return Type −
func nestedFunction(name1: Int, name2: Int) -> ReturnType{ // code return }
Example
Swift program to calculate the sum of two figures(rectangle and square) using nested function.
import Foundation // Outer function without return type func Areas(length: Int, width: Int){ // Nested function with return type func sumOfAreas(area1: Int, area2: Int)-> Int { let Sum = area1 + area2 return Sum } let areaOfRectangle = length * width let areaOfSquare = length * length // Calling nested function print("Total Area: \(sumOfAreas(area1: areaOfRectangle, area2: areaOfSquare))") } // Calling the outer function to display the result Areas(length: 23, width: 25)
Output
It will produce the following output −
Total Area: 1104