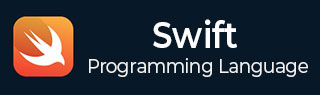
- Swift Tutorial
- Swift - Home
- Swift - Overview
- Swift - Environment
- Swift - Basic Syntax
- Swift - Variables
- Swift - Constants
- Swift - Literals
- Swift - Comments
- Swift Operators
- Swift - Operators
- Swift - Arithmetic Operators
- Swift - Comparison Operators
- Swift - Logical Operators
- Swift - Assignment Operators
- Swift - Bitwise Operators
- Swift - Misc Operators
- Swift Advanced Operators
- Swift - Operator Overloading
- Swift - Arithmetic Overflow Operators
- Swift - Identity Operators
- Swift - Range Operators
- Swift Data Types
- Swift - Data Types
- Swift - Integers
- Swift - Floating-Point Numbers
- Swift - Double
- Swift - Boolean
- Swift - Strings
- Swift - Characters
- Swift - Type Aliases
- Swift - Optionals
- Swift - Tuples
- Swift - Assertions and Precondition
- Swift Control Flow
- Swift - Decision Making
- Swift - if statement
- Swift - if...else if...else Statement
- Swift - if-else Statement
- Swift - nested if statements
- Swift - switch statement
- Swift - Loops
- Swift - for in loop
- Swift - While loop
- Swift - repeat...while loop
- Swift - continue statement
- Swift - break statement
- Swift - fall through statement
- Swift Collections
- Swift - Arrays
- Swift - Sets
- Swift - Dictionaries
- Swift Functions
- Swift - Functions
- Swift - Nested Functions
- Swift - Function Overloading
- Swift - Recursion
- Swift - Higher-Order Functions
- Swift Closures
- Swift - Closures
- Swift-Escaping and Non-escaping closure
- Swift - Auto Closures
- Swift OOps
- Swift - Enumerations
- Swift - Structures
- Swift - Classes
- Swift - Properties
- Swift - Methods
- Swift - Subscripts
- Swift - Inheritance
- Swift-Overriding
- Swift - Initialization
- Swift - Deinitialization
- Swift Advanced
- Swift - ARC Overview
- Swift - Optional Chaining
- Swift - Error handling
- Swift - Concurrency
- Swift - Type Casting
- Swift - Nested Types
- Swift - Extensions
- Swift - Protocols
- Swift - Generics
- Swift - Access Control
- Swift - Function vs Method
- Swift - SwiftyJSON
- Swift - Singleton class
- Swift Random Numbers
- Swift Opaque and Boxed Type
- Swift Useful Resources
- Swift - Compile Online
- Swift - Quick Guide
- Swift - Useful Resources
- Swift - Discussion
Swift - Literals
What are Literals?
A literal is the source code representation of a value of an integer, floating-point number, or string type. Or we can say that literals are used to represent the actual values that are used to assign a constant or variable. For example, 34 is an integer literal, 23.45 is a floating point literal. They are directly used in the program. We cannot directly perform operations on the literals because they are fixed values, but we can perform operations on the variables or constants (initialized with literals).
Type of Swift Literals
Swift supports the following types of literals −
Integer literals
Floating-point literals
String literals
Boolean literals
Swift Integer Literals
An integer literal is used to represent an integer value or whole number. We can specify a negative or positive value in the integer literal, for example, -10 or 10. An integer literal can contain leading zeros but they do not have any effect on the value of the literal, for example, 010 and 10 both are the same.
Integer literals are of the following types −
Decimal Integer Literal − It is the most commonly used form of the integer literal. For example, 34.
Binary Integer Literal − It is used to represent base 2 or binary values. It is prefixed with “0b”. For example, 0b1011.
Octal Integer Literal − It is used to represent base 8 or octal values. It is prefixed with “0o”. For example, 0o53.
Hexadecimal Integer Literal − It is used to represent base 16 or hexadecimal values. It is prefixed with “0x”. For example, 0xFF.
Example
Swift program to demonstrate integer literals −
import Foundation // Decimal integer literal let num1 = 34 print("Decimal Integer: \(num1)") // Binary integer literal let num2 = 0b101011 print("Binary Integer: \(num2)") // Octal integer literal let num3 = 0o52 print("Octal Integer: \(num3)") // Hexadecimal integer literal let num4 = 0x2C print("Hexadecimal Integer: \(num4)")
Output
Decimal Integer: 34 Binary Integer: 43 Octal Integer: 42 Hexadecimal Integer: 44
Swift Floating-point Literals
A floating-point literal is used to represent a number with the fractional component, for example, 34.567. We can specify a negative or positive value in the floating-point literal, for example, -23.45 or 2.34. A floating point literal can contain an underscore(_), but it does not have any effect on the overall value of the literal, for example, 0.2_3 and 23 both are the same. Floating point literals are of the following types −
Decimal Floating-point Literals − It consists of a sequence of decimal digits followed by either a decimal fraction, a decimal exponent, or both. For example, 12.1875.
Hexadecimal Floating-point Literals − It consists of a 0x prefix, followed by an optional hexadecimal fraction, followed by a hexadecimal exponent. For example, 0xC.3p0.
Exponential Literal − It is used to represent the power of 10. It contains the letter “e”. For example, 1.243e4.
Example
Swift program to demonstrate floating point literals.
import Foundation // Decimal floating-point literal let num1 = 32.14 print("Decimal Float: \(num1)") // Exponential notation floating-point literal let num2 = 2.5e3 print("Exponential Float: \(num2)") // Hexadecimal floating-point literal let num3 = 0x1p-2 print("Hexadecimal Float: \(num3)")
Output
Decimal Float: 32.14 Exponential Float: 2500.0 Hexadecimal Float: 0.25
Swift String Literals
A string literal is a sequence of characters surrounded by double quotes, for example, "characters". String literals can be represented in the following ways −
Double Quoted String − It is used to represent single-line literals. For example, "hello".
Multiline String − It is used to represent multiline literals. It can contain multiple lines without any escape character. For example −
"""Hello I like your car """
String literals cannot contain an un escaped double quote ("), an un escaped backslash (\), a carriage return, or a line feed. Special characters can be included in string literals using the following escape sequences &miinus;
Escape Sequence | Name |
---|---|
\0 | Null Character |
\\ | \character |
\b | Backspace |
\f | Form feed |
\n | Newline |
\r | Carriage return |
\t | Horizontal tab |
\v | Vertical tab |
\' | Single Quote |
\" | Double Quote |
\000 | Octal number of one to three digits |
\xhh... | Hexadecimal number of one or more digits |
Example
Swift program to demonstrate string literals.
import Foundation // Double-quoted string literal let str1 = "Swift is good programming language" print(str1) // Multi-line string literal let str2 = """ Hello priya is at Swift programming """ print(str2) // Special characters in a string literal let str3 = "Escape characters: \n\t\"\'\\" print(str3)
Output
Hello priya is at Swift programming Escape characters: "'\
Swift Boolean Literals
TBoolean literals are used to represent Boolean values true and false. They are generally used to represent conditions. Boolean literals are of two types −
true − It represents a true condition.
false − It represents a true condition.
Example
Swift program to demonstrate Boolean literals.
import Foundation // Boolean literals in Swift let value1 = true let value2 = false print("Boolean Literal: \(value1)") print("Boolean Literal: \(value2)")
Output
Boolean Literal: true Boolean Literal: false