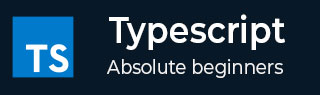
- TypeScript Basics
- TypeScript - Home
- TypeScript - Overview
- TypeScript - Environment Setup
- TypeScript - Basic Syntax
- TypeScript vs. JavaScript
- TypeScript - Features
- TypeScript - Variables
- TypeScript - let & const
- TypeScript - Operators
- TypeScript Basic Types
- TypeScript - Types
- TypeScript - Type Annotations
- TypeScript - Type Inference
- TypeScript - Numbers
- TypeScript - Strings
- TypeScript - Boolean
- TypeScript - Arrays
- TypeScript - Tuples
- TypeScript - Enums
- TypeScript - Any
- TypeScript - Never
- TypeScript - Union
- TypeScript - Literal Types
- TypeScript - Symbols
- TypeScript - null vs. undefined
- TypeScript - Type Aliases
- TypeScript Control Flow
- TypeScript - Decision Making
- TypeScript - If Statement
- TypeScript - If Else Statement
- TypeScript - Nested If Statements
- TypeScript - Switch Statement
- TypeScript - Loops
- TypeScript - For Loop
- TypeScript - While Loop
- TypeScript - Do While Loop
- TypeScript Functions
- TypeScript - Functions
- TypeScript - Function Types
- TypeScript - Optional Parameters
- TypeScript - Default Parameters
- TypeScript - Anonymous Functions
- TypeScript - Function Constructor
- TypeScript - Rest Parameter
- TypeScript - Parameter Destructuring
- TypeScript - Arrow Functions
- TypeScript Interfaces
- TypeScript - Interfaces
- TypeScript - Extending Interfaces
- TypeScript Classes and Objects
- TypeScript - Classes
- TypeScript - Objects
- TypeScript - Access Modifiers
- TypeScript - Readonly Properties
- TypeScript - Inheritance
- TypeScript - Static Methods and Properties
- TypeScript - Abstract Classes
- TypeScript - Accessors
- TypeScript - Duck-Typing
- TypeScript Advanced Types
- TypeScript - Intersection Types
- TypeScript - Type Guards
- TypeScript - Type Assertions
- TypeScript Type Manipulation
- TypeScript - Creating Types from Types
- TypeScript - Keyof Type Operator
- TypeScript - Typeof Type Operator
- TypeScript - Indexed Access Types
- TypeScript - Conditional Types
- TypeScript - Mapped Types
- TypeScript - Template Literal Types
- TypeScript Generics
- TypeScript - Generics
- TypeScript - Generic Constraints
- TypeScript - Generic Interfaces
- TypeScript - Generic Classes
- TypeScript Miscellaneous
- TypeScript - Triple-Slash Directives
- TypeScript - Namespaces
- TypeScript - Modules
- TypeScript - Ambients
- TypeScript - Decorators
- TypeScript - Type Compatibility
- TypeScript - Date Object
- TypeScript - Iterators and Generators
- TypeScript - Mixins
- TypeScript - Utility Types
- TypeScript - Boxing and Unboxing
- TypeScript - tsconfig.json
- From JavaScript To TypeScript
- TypeScript Useful Resources
- TypeScript - Quick Guide
- TypeScript - Useful Resources
- TypeScript - Discussion
TypeScript - Triple-Slash Directives
Triple-slash directives are similar to single-line comments which contain a single XML tag. They are used to provide instructions to the TypeScript compiler about processing the TypeScript file. Triple-slash directives start with three forward slashes (///) and are mainly placed at the top of the code file.
Triple-slash directives are mostly used in TypeScript. However, they can also be used with JavaScript projects that use TypeScript.
In TypeScript, you can use triple-slash directives for two purposes.
Reference Directives
Reference directives are mainly used to tell the compiler to include another TypeScript file in the compilation process. They specify the dependencies between multiple files. Furthermore, they are also used to declare dependency packages and include libraries in the TypeScript file.
Syntax
/// <reference path="file_path" />
In the above syntax, we have used three forward slashes first to define the triple-slash directives. After that, we used the XML tag <reference /> and added a path attribute to it. The path attribute takes the file path as a value.
Similarly, you can also use other XML tags with three forward slashes.
Types of Triple-Slash Reference Directives
Here are the most commonly used triple-slash reference directives in a JavaScript/TypeScript environment:
///<reference path="..." /> − It is used to add a reference of one TypeScript file in another file.
///<reference types="..." /> − It is used to declare a dependency on a package.
///<reference lib="..." /> − It is used to include a library file that is part of the compilation context.
Example: Referencing File Paths
The main purpose of the triple-slash directives is to reference other files in particular TypeScript files.
Filename: MathFunctions.ts
In the below code, we have defined the add() function that takes two numbers as a parameter and returns the sum of them. We also export that function using the export keyword to use it in a different file.
// This file will be referenced in app.ts export function add(a: number, b: number): number { return a + b; }
Filename: app.ts
In this file, we have imported the add() function from the mathFunctions file. We have also used the reference directive in this file to tell the file path to the compiler.
/// <reference path="mathFunctions.ts" /> import { add } from './mathFunctions'; console.log('Addition of 10 and 20 is:', add(10, 20));
Output
Addition of 10 and 20 is 30
Example: Referencing Type Definition
The triple-slash directives can also be used to specify the reference type definition for the external module. You can use the <reference type="module_type" /> directive to specify the type of the module.
App.ts
In the code below, we have imported the ‘fs’ module. Before that, we have used the triple-slash directive to specify the type of the module.
Next, we used the readFileSync() method of the ‘fs’ module to read the file content.
/// <reference types="node" /> import * as fs from 'fs'; const content = fs.readFileSync('sample.txt', 'utf8'); console.log('File content:', content);
Sample.txt
The below file contains plain text.
Hello, this is a sample text file.
Output
File content: Hello, this is a sample text file.
Example: Including Libraries
When you want to use a specific library during the TypeScript compilation, you can use the ///<reference lib="lib_name" /> triple-slash directive to specify it.
In the code below, we have used the triple-slash directive to specify the “es2015.array” library.
/// <reference lib="es2015.array" /> const str = ["Hello", "1", "World", "2"]; const includesTwo = str.includes("2"); console.log(includesTwo);
Output
true
Module System Directives
Module system directives are used to specify how modules are loaded in the TypeScript file. They are used to load modules asynchronously.
There are two types of module system directives in TypeScript:
- ///<amd-module name="..." />: It is used to specify the module name for loading.
- ///<amd-dependency path="..." />: It is used to specify the path for the dependencies of the AMD module.
Example
In the code below, we have used the AMD-module triple-slash directive which specifies to load the ‘MyModule’ module asynchronously.
/// <amd-module name="MyModule" /> export function displayMessage() { return "Hello from MyModule"; }
Triple-slash directives allow developers to enhance JavaScript projects by providing advanced compilation options, module loading, and integration with various types and libraries.