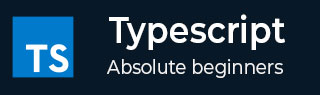
- TypeScript Basics
- TypeScript - Home
- TypeScript - Overview
- TypeScript - Environment Setup
- TypeScript - Basic Syntax
- TypeScript vs. JavaScript
- TypeScript - Features
- TypeScript - Variables
- TypeScript - let & const
- TypeScript - Operators
- TypeScript Basic Types
- TypeScript - Types
- TypeScript - Type Annotations
- TypeScript - Type Inference
- TypeScript - Numbers
- TypeScript - Strings
- TypeScript - Boolean
- TypeScript - Arrays
- TypeScript - Tuples
- TypeScript - Enums
- TypeScript - Any
- TypeScript - Never
- TypeScript - Union
- TypeScript - Literal Types
- TypeScript - Symbols
- TypeScript - null vs. undefined
- TypeScript - Type Aliases
- TypeScript Control Flow
- TypeScript - Decision Making
- TypeScript - If Statement
- TypeScript - If Else Statement
- TypeScript - Nested If Statements
- TypeScript - Switch Statement
- TypeScript - Loops
- TypeScript - For Loop
- TypeScript - While Loop
- TypeScript - Do While Loop
- TypeScript Functions
- TypeScript - Functions
- TypeScript - Function Types
- TypeScript - Optional Parameters
- TypeScript - Default Parameters
- TypeScript - Anonymous Functions
- TypeScript - Function Constructor
- TypeScript - Rest Parameter
- TypeScript - Parameter Destructuring
- TypeScript - Arrow Functions
- TypeScript Interfaces
- TypeScript - Interfaces
- TypeScript - Extending Interfaces
- TypeScript Classes and Objects
- TypeScript - Classes
- TypeScript - Objects
- TypeScript - Access Modifiers
- TypeScript - Readonly Properties
- TypeScript - Inheritance
- TypeScript - Static Methods and Properties
- TypeScript - Abstract Classes
- TypeScript - Accessors
- TypeScript - Duck-Typing
- TypeScript Advanced Types
- TypeScript - Intersection Types
- TypeScript - Type Guards
- TypeScript - Type Assertions
- TypeScript Type Manipulation
- TypeScript - Creating Types from Types
- TypeScript - Keyof Type Operator
- TypeScript - Typeof Type Operator
- TypeScript - Indexed Access Types
- TypeScript - Conditional Types
- TypeScript - Mapped Types
- TypeScript - Template Literal Types
- TypeScript Generics
- TypeScript - Generics
- TypeScript - Generic Constraints
- TypeScript - Generic Interfaces
- TypeScript - Generic Classes
- TypeScript Miscellaneous
- TypeScript - Triple-Slash Directives
- TypeScript - Namespaces
- TypeScript - Modules
- TypeScript - Ambients
- TypeScript - Decorators
- TypeScript - Type Compatibility
- TypeScript - Date Object
- TypeScript - Iterators and Generators
- TypeScript - Mixins
- TypeScript - Utility Types
- TypeScript - Boxing and Unboxing
- TypeScript - tsconfig.json
- From JavaScript To TypeScript
- TypeScript Useful Resources
- TypeScript - Quick Guide
- TypeScript - Useful Resources
- TypeScript - Discussion
TypeScript - Features
TypeScript is a superset of JavaScript. So, it contains all the features that JavaScript has. However, it also contains some advanced features that JavaScript doesn't have like static typing, interface, etc.
Let's discuss some of the important features of TypeScript.
Type Annotation
In TypeScript, type annotation allows you to declare the type of variable, function parameters, and return value. The static typing feature of TypeScript allows you to catch type-related errors while writing the code instead of at the compile time. This way developers can write more reliable code.
Example
In the code below, we have defined the variable 'a' of the number data type. The printNumber() function takes the 'num' parameter of the 'number' type.
The function prints the parameter value.
// Type annotation in TypeScript var a: number = 10; function printNumber(num: number) { console.log(num); } printNumber(a);
On compiling, it will generate the following JavaScript code.
// Type annotation in TypeScript var a = 10; function printNumber(num) { console.log(num); } printNumber(a);
Output
The above example code will produce the following output –
10
Interfaces
Interfaces are similar to the abstract classes in other programming languages like Java. It allows developers to define the structure of the object but doesn't provide the implementation. This way developers can adhere to the same structure of the object for similar kinds of objects.
Example
In the example below, we have defined the 'Iperson' interface that contains the 'firstName', and 'lastName' properties and getFullName() method. The interface declares the properties and methods only, defining the structure of the object.
For the 'obj' object, we have used the 'IPerson' interface as a type. After that, we initialized the properties of an object and implemented the getFullName() method which returns the string value.
// Interfaces in TypeScript interface IPerson { firstName: string; lastName: string; getFullName(): string; } // Define an object that implements the interface let obj: IPerson = { firstName: "John", lastName: "Doe", getFullName(): string { return this.firstName + " " + this.lastName; } }; console.log(obj.getFullName());
On compiling, it will generate the following JavaScript code.
// Define an object that implements the interface let obj = { firstName: "John", lastName: "Doe", getFullName() { return this.firstName + " " + this.lastName; } }; console.log(obj.getFullName());
Output
The output of the above example code is as follows –
John Doe
Classes
Classes are a blueprint of the objects. Classes can contain properties, and methods which can be accessed using an instance of classes. You can use class constructor() to initialize the properties of the class while creating the instance of the class. Furthermore, you can also have static members inside the classes which can be accessed through the class name and without using an instance of the class.
Example
In the code below, we have created the Greeter class, which contains the 'greeting' property. The constructor() method takes the 'message' parameter and initializes the 'greeting' property values with it.
The greet() method returns the string value, representing the greeting message. After that, we have created the instance of the Greeter class and called the greet() method using it.
// Basic example of class class Greeter { greeting: string; // Constructor method constructor(message: string) { this.greeting = message; } // Class Method greet() { return "Hello, " + this.greeting; } } // Create an instance of the class let greeter = new Greeter("world"); console.log(greeter.greet()); // Hello, world
On compiling, it will generate the following JavaScript code.
// Basic example of class class Greeter { // Constructor method constructor(message) { this.greeting = message; } // Class Method greet() { return "Hello, " + this.greeting; } } // Create an instance of the class let greeter = new Greeter("world"); console.log(greeter.greet()); // Hello, world
Output
The output of the above example code is as follows –
Hello, world
Inheritance
TypeScript supports all features of the object-oriented programming language like polymorphism, abstraction, encapsulation, inheritance etc. However, we have covered inheritance only in this lesson.
Inheritance allows you to reuse the properties and methods of other classes.
Example
In the code below, the 'Person' class is a base class. It contains the 'name' property, which we initialize in the constructor() method. The display() method prints the name in the console.
The Employee class inherits the properties of the Parent class using the 'extends' keyword. It contains the 'empCode' property and show() method. It also contains all properties and methods of the Person class.
Next, we created the instance of the Employee class and accessed the method of the Person class using it.
// Base class class Person { name: string; constructor(name: string) { this.name = name; } display(): void { console.log(this.name); } } // Derived class class Employee extends Person { empCode: number; constructor(name: string, code: number) { super(name); this.empCode = code; } show(): void { console.log(this.empCode); } } let emp: Employee = new Employee("John", 123); emp.display(); // John emp.show(); // 123
On compiling, it will produce the following JavaScript code.
// Base class class Person { constructor(name) { this.name = name; } display() { console.log(this.name); } } // Derived class class Employee extends Person { constructor(name, code) { super(name); this.empCode = code; } show() { console.log(this.empCode); } } let emp = new Employee("John", 123); emp.display(); // John emp.show(); // 123
Output
The output of the above example code is as follows –
John 123
Enums
Enums are used to define the named constants in TypeScript. It allows you to give names to the constant values, which makes code more reliable and readable.
Example
In the code below, we have used the 'enum' keyword to define the enum. In our case, the integer value represents the directions, but we have given names to the directions for better readability.
After that, you can use the constant name to access the value of the Directions.
// Enums in TypeScript enum Direction { Up = 1, Down, Left, Right } console.log(Direction.Up); // 1 console.log(Direction.Down); // 2 console.log(Direction.Left); // 3 console.log(Direction.Right); // 4
On compiling, it will produce the following JavaScript code-
var Direction; (function (Direction) { Direction[Direction["Up"] = 1] = "Up"; Direction[Direction["Down"] = 2] = "Down"; Direction[Direction["Left"] = 3] = "Left"; Direction[Direction["Right"] = 4] = "Right"; })(Direction || (Direction = {})); console.log(Direction.Up); // 1 console.log(Direction.Down); // 2 console.log(Direction.Left); // 3 console.log(Direction.Right); // 4
Output
The output of the above example code is as follows –
1 2 3 4
Generics
Generic types allow you to create reusable components, function codes, or classes that can work with different types, rather than working with the specific types. This way developers can use the same function or classes with multiple types.
Example
In the code below, printArray() is a generic function that has a type parameter
Next, we have called the function by passing the number and string array. You can observe that the function takes an array of any type as a parameter. This way, developers can use the same code with different types.
// Generics in TypeScript function printArray(arr: T[]): void { for (let i = 0; i < arr.length; i++) { console.log(arr[i]); } } printArray ([1, 2, 3]); // Array of numbers printArray (["a", "b", "c"]); // Array of strings
On compiling, it will produce the following JavaScript code.
// Generics in TypeScript function printArray(arr) { for (let i = 0; i < arr.length; i++) { console.log(arr[i]); } } printArray([1, 2, 3]); // Array of numbers printArray(["a", "b", "c"]); // Array of strings
Output
The output of the above example code is as follows –
1 2 3 a b c
Union Types
The union types allow you to declare the multiple types for variables. Sometimes, developers are required to define a single variable that supports number, string, null, etc. types. In this case, they can use union types.
Example
In the code below, the 'unionType' has string and number type. It can store both types of values but if you try to store the value of any other type like Boolean, the TypeScript compiler will throw an error.
// Union types in TypeScript var unionType: string | number; unionType = "Hello World"; // Assigning a string value console.log("String value: " + unionType); unionType = 500; // Assigning a number value console.log("Number value: " + unionType); // unionType = true; // Error: Type 'boolean' is not assignable to type 'string | number'
On compiling, it will generate the following JavaScript code.
// Union types in TypeScript var unionType; unionType = "Hello World"; // Assigning a string value console.log("String value: " + unionType); unionType = 500; // Assigning a number value console.log("Number value: " + unionType); // unionType = true; // Error: Type 'boolean' is not assignable to type 'string | number'
Output
The output of the above exmaple code is as follows –
String value: Hello World Number value: 500
Type Guards
Type guards allow you to get the type of variables. After that, you can perform multiple operations based on the type of the particular variables. This also ensures the type-safety.
Example
In the code below, we have defined the variable 'a' of type number and string.
After that, we used the 'typeof' operator with the if-else statement to get the type of the variable 'a'. Based on the type of the 'a', we print the string value in the console.
let a: number | string = 10; // Type Guard if (typeof a === 'number') { console.log('a is a number'); } else { console.log('a is a string'); }
On compiling, it will generate the following JavaScript code.
let a = 10; // Type Guard if (typeof a === 'number') { console.log('a is a number'); } else { console.log('a is a string'); }
Output
The output of the above example code is as follows −
a is a number
We have covered the most important features of TypeScript in this lesson. TypeScript also contains features like optional chaining, decorators, modules, type interfaces, etc. which we will explore through this TypeScript course.