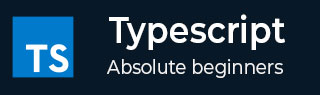
- TypeScript Basics
- TypeScript - Home
- TypeScript - Overview
- TypeScript - Environment Setup
- TypeScript - Basic Syntax
- TypeScript vs. JavaScript
- TypeScript - Features
- TypeScript - Variables
- TypeScript - let & const
- TypeScript - Operators
- TypeScript Basic Types
- TypeScript - Types
- TypeScript - Type Annotations
- TypeScript - Type Inference
- TypeScript - Numbers
- TypeScript - Strings
- TypeScript - Boolean
- TypeScript - Arrays
- TypeScript - Tuples
- TypeScript - Enums
- TypeScript - Any
- TypeScript - Never
- TypeScript - Union
- TypeScript - Literal Types
- TypeScript - Symbols
- TypeScript - null vs. undefined
- TypeScript - Type Aliases
- TypeScript Control Flow
- TypeScript - Decision Making
- TypeScript - If Statement
- TypeScript - If Else Statement
- TypeScript - Nested If Statements
- TypeScript - Switch Statement
- TypeScript - Loops
- TypeScript - For Loop
- TypeScript - While Loop
- TypeScript - Do While Loop
- TypeScript Functions
- TypeScript - Functions
- TypeScript - Function Types
- TypeScript - Optional Parameters
- TypeScript - Default Parameters
- TypeScript - Anonymous Functions
- TypeScript - Function Constructor
- TypeScript - Rest Parameter
- TypeScript - Parameter Destructuring
- TypeScript - Arrow Functions
- TypeScript Interfaces
- TypeScript - Interfaces
- TypeScript - Extending Interfaces
- TypeScript Classes and Objects
- TypeScript - Classes
- TypeScript - Objects
- TypeScript - Access Modifiers
- TypeScript - Readonly Properties
- TypeScript - Inheritance
- TypeScript - Static Methods and Properties
- TypeScript - Abstract Classes
- TypeScript - Accessors
- TypeScript - Duck-Typing
- TypeScript Advanced Types
- TypeScript - Intersection Types
- TypeScript - Type Guards
- TypeScript - Type Assertions
- TypeScript Type Manipulation
- TypeScript - Creating Types from Types
- TypeScript - Keyof Type Operator
- TypeScript - Typeof Type Operator
- TypeScript - Indexed Access Types
- TypeScript - Conditional Types
- TypeScript - Mapped Types
- TypeScript - Template Literal Types
- TypeScript Generics
- TypeScript - Generics
- TypeScript - Generic Constraints
- TypeScript - Generic Interfaces
- TypeScript - Generic Classes
- TypeScript Miscellaneous
- TypeScript - Triple-Slash Directives
- TypeScript - Namespaces
- TypeScript - Modules
- TypeScript - Ambients
- TypeScript - Decorators
- TypeScript - Type Compatibility
- TypeScript - Date Object
- TypeScript - Iterators and Generators
- TypeScript - Mixins
- TypeScript - Utility Types
- TypeScript - Boxing and Unboxing
- TypeScript - tsconfig.json
- From JavaScript To TypeScript
- TypeScript Useful Resources
- TypeScript - Quick Guide
- TypeScript - Useful Resources
- TypeScript - Discussion
TypeScript - Access Modifiers
The concept access modifiers are used to implement encapsulation or data hiding in TypeScript. The access modifiers define the visibility class members outside the defining class. The class members are properties and functions. The access modifiers are also known as access specifiers.
TypeScript supports three types of access modifiers – public, private and protected. These modifiers are the keywords that are used to declare a class member as public, private or protected.
A public class member is accessible from anywhere in the code. A private member is only accessible within the class that defines it. And a protected member is accessible from within the class and derived classes.
Let's understand each of the three access modifiers discussed above in details.
Public Access Modifier
A public access modifier in TypeScript defines a public class member. By default, a member of class is public. So it's optional to use public keyword to declare a member public. The public members are accessible from anywhere within and outside the class that defines the member.
Example
In the example below, we have created a class named Person. The class Person has two members, one a public property name and other a public method greet(). As these members are declared as public, these can be accessed from anywhere in the program.
We also created an instance of the Person class as person. We access the name property of the person object and assign a value to it. Finally, we called the greet function to display the greeting with new name.
class Person { public name: string = ""; public greet(): void { console.log(`Hello, my name is ${this.name}!`); } } const person = new Person(); person.name = "John"; person.greet();
On compiling, it will generate the following JavaScript code.
class Person { constructor() { this.name = ""; } greet() { console.log(`Hello, my name is ${this.name}!`); } } const person = new Person(); person.name = "John"; person.greet();
The output of the above example code is as follows –
Hello, my name is John!
Private Access Modifiers
A private access modifier restricts the access of the class member (property or method) to the class where it is declared. When you add the private modifier to the property or method, you can access that property or method within the same class only.
A private access modifier in TypeScript defines a private class member. Private members are accessible from within the class that defines them.
Example
In this example, we have created a BankAccount class with a private property balance, which can only be accessed and modified within the class. Additionally, we have a private method calculateInterest(), that calculates the interest based on the balance.
You can observe in the output that attempting to access the private members will result in a TypeError.
class BankAccount { private balance: number; constructor(initialBalance: number) { this.balance = initialBalance; } private calculateInterest(): number { const interestRate = 0.05; return this.balance * interestRate; } } // Creating an instance of the BankAccount class const account = new BankAccount(1000); // Attempting to access private members console.log(account.balance); console.log(account.calculateInterest());
On compiling, it will generate the following JavaScript code.
class BankAccount { constructor(initialBalance) { this.balance = initialBalance; } calculateInterest() { const interestRate = 0.05; return this.balance * interestRate; } } // Creating an instance of the BankAccount class const account = new BankAccount(1000); // Attempting to access private members console.log(account.balance); console.log(account.calculateInterest());
And it will give the following error –
Property 'balance' is private and only accessible within class 'BankAccount'. Property 'calculateInterest' is private and only accessible within class 'BankAccount'.
The output of the above example code is as follows –
1000 50
Protected Access Modifiers
The protected access modifier is used to define a protected class member (property or method). A protected data member is accessible only within the class that defines it or any class that extends it.
Example
In this example, we create a base class Animal with a protected property name, which can be accessed and modified within the class and its derived classes. We also have a protected method makeSound(), that simply logs a message.
We then create a derived class Dog that extends the Animal class. The Dog class adds a public method bark(), which utilizes the name property inherited from the base class to output a bark message.
Finally, we create an instance of the Dog class named dog with the name "Buddy" and call the bark() method.
The output will show the dog's name followed by the bark message.
class Animal { protected name: string; constructor(name: string) { this.name = name; } protected makeSound(): void { console.log("The animal makes a sound"); } } class Dog extends Animal { public bark(): void { console.log(`${this.name} barks!`); } } // Creating an instance of the Dog class const dog = new Dog("Buddy"); dog.bark();
On compiling, it will generate the following JavaScript code.
class Animal { constructor(name) { this.name = name; } makeSound() { console.log("The animal makes a sound"); } } class Dog extends Animal { bark() { console.log(`${this.name} barks!`); } } // Creating an instance of the Dog class const dog = new Dog("Buddy"); dog.bark();
The output of the above example code is as follows –
Buddy barks!