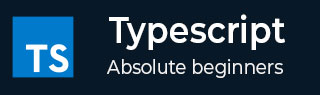
- TypeScript Basics
- TypeScript - Home
- TypeScript - Overview
- TypeScript - Environment Setup
- TypeScript - Basic Syntax
- TypeScript vs. JavaScript
- TypeScript - Features
- TypeScript - Variables
- TypeScript - let & const
- TypeScript - Operators
- TypeScript Basic Types
- TypeScript - Types
- TypeScript - Type Annotations
- TypeScript - Type Inference
- TypeScript - Numbers
- TypeScript - Strings
- TypeScript - Boolean
- TypeScript - Arrays
- TypeScript - Tuples
- TypeScript - Enums
- TypeScript - Any
- TypeScript - Never
- TypeScript - Union
- TypeScript - Literal Types
- TypeScript - Symbols
- TypeScript - null vs. undefined
- TypeScript - Type Aliases
- TypeScript Control Flow
- TypeScript - Decision Making
- TypeScript - If Statement
- TypeScript - If Else Statement
- TypeScript - Nested If Statements
- TypeScript - Switch Statement
- TypeScript - Loops
- TypeScript - For Loop
- TypeScript - While Loop
- TypeScript - Do While Loop
- TypeScript Functions
- TypeScript - Functions
- TypeScript - Function Types
- TypeScript - Optional Parameters
- TypeScript - Default Parameters
- TypeScript - Anonymous Functions
- TypeScript - Function Constructor
- TypeScript - Rest Parameter
- TypeScript - Parameter Destructuring
- TypeScript - Arrow Functions
- TypeScript Interfaces
- TypeScript - Interfaces
- TypeScript - Extending Interfaces
- TypeScript Classes and Objects
- TypeScript - Classes
- TypeScript - Objects
- TypeScript - Access Modifiers
- TypeScript - Readonly Properties
- TypeScript - Inheritance
- TypeScript - Static Methods and Properties
- TypeScript - Abstract Classes
- TypeScript - Accessors
- TypeScript - Duck-Typing
- TypeScript Advanced Types
- TypeScript - Intersection Types
- TypeScript - Type Guards
- TypeScript - Type Assertions
- TypeScript Type Manipulation
- TypeScript - Creating Types from Types
- TypeScript - Keyof Type Operator
- TypeScript - Typeof Type Operator
- TypeScript - Indexed Access Types
- TypeScript - Conditional Types
- TypeScript - Mapped Types
- TypeScript - Template Literal Types
- TypeScript Generics
- TypeScript - Generics
- TypeScript - Generic Constraints
- TypeScript - Generic Interfaces
- TypeScript - Generic Classes
- TypeScript Miscellaneous
- TypeScript - Triple-Slash Directives
- TypeScript - Namespaces
- TypeScript - Modules
- TypeScript - Ambients
- TypeScript - Decorators
- TypeScript - Type Compatibility
- TypeScript - Date Object
- TypeScript - Iterators and Generators
- TypeScript - Mixins
- TypeScript - Utility Types
- TypeScript - Boxing and Unboxing
- TypeScript - tsconfig.json
- From JavaScript To TypeScript
- TypeScript Useful Resources
- TypeScript - Quick Guide
- TypeScript - Useful Resources
- TypeScript - Discussion
TypeScript - Arrow Functions
Arrow functions are a concise way to write the anonymous functions in TypeScript. They are introduced in ES6 (ECMAScript 2015). They offer a shorter and more readable syntax compared to traditional function declarations. Arrow functions are also called lambda functions. Lambda refers to the anonymous functions in programming.
Syntax
You can define an arrow function using a fat arrow (=>). The fat arrow is used to separate function parameters from the function body (statements).
(param1, param2, ..., paramN) => statement
There are 3 parts to an arrow function −
Parameters − A function may optionally have parameters, param1, param2, ..., paramN.
The fat arrow notation/lambda notation (=>) − It is also called as the goes to operator.
Statements − represent the function’s instruction set
Examples
Let's understand the arrow functions/ lambda function with the help of some examples in TypeScript −
Example: Arrow function with single statement
We can write an arrow function with single statement as follows −
(x: number, y: number): number => x +y;
For a single statement, it's optional to write the return keyword to return a value and braces (curly brackets. But if you are using braces, it is required to write the return keyword to return a value.
(x: number, y: number): number => {return x +y;}
Let's look at the following example −
const add = (x: number, y: number): number => x +y; console.log(add(20,30));
On compiling, it will generate the following JavaScript code.
const add = (x, y) => x + y; console.log(add(20, 30));
It will produce the following result −
50
Example: Arrow function with multiple statement
You can write multiple statements using curly brackets. Also you need to write the return statement to return a value.
In the example below, we defined an arrow function that accepts two parameters and returns their product.
const multiply = (x: number, y: number): number => { let res: number = x * y; return res; }; console.log(multiply(5,6));
On compiling, it will generate the following JavaScript code.
const multiply = (x, y) => { let res = x * y; return res; }; console.log(multiply(5, 6));
It will produce the following output −
30
Example: Arrow function with no parameter
The parameters to the arrow functions are optional.
In the example below, we have defined an arrow function that takes no parameter and return a string value.
const greet = () => { return "Hello World!";} console.log(greet());
On compiling, it will generate the same JavaScript code.
The output of the above code example is as follows −
Hello World!
Example: Arrow function with a single parameter
The following example show an arrow function that take a single parameter and returns the sum of the parameter and 10.
const add = (x: number): number => {return x + 10;} console.log(add(5));
On compiling, it will generate the following JavaScript code.
const add = (x) => { return x + 10; }; console.log(add(5));
The output of the above code example is as follows −
15
Example: Arrow function with multiple parameters
In the below example, we defined an arrow function that accept three parameters and return their sum.
let sum: number; const add = (a: number, b: number, c: number) => { sum = a + b + c; return sum; }; let res = add(10, 30, 45); console.log("The result is: " + res);
On compiling, it will generate the following JavaScript code.
let sum; const add = (a, b, c) => { sum = a + b + c; return sum; }; let res = add(10, 30, 45); console.log("The result is: " + res);
The output of the above code example is as follows −
The result is: 85
Example: Arrow function with default parameters
In the below example, we passed the default value of the parameters to the arrow function. When you will not pass the corresponding argument values, these default values will be used inside the function body.
const mul = (a = 10, b = 15) => a * b; console.log("mul(5, 8) = " + mul(5, 8)); console.log("mul(6) = " + mul(6)); console.log("mul() = " + mul());
On compiling, it will generate the same JavaScript code.
The output of the above code example is as follows −
mul(5, 8) = 40 mul(6) = 90 mul() = 150
Applications of Arrow Functions
Let's now discuss some application examples of the arrow function in TypeScript.
Example: Arrow functions as callback function in other function
Arrow functions (Lambda expressions) are also useful as callbacks in functions such as array methods (e.g., map, filter, reduce). Here's an example demonstrating their usage with the "map" method −
const numbers = [1, 2, 3, 4, 5]; const squaredNumbers = numbers.map(x => x * x); console.log(`Original array: ${numbers}`); console.log(`Squared array: ${squaredNumbers}`);
On compiling, it will generate the same JavaScript code.
The output of the above code example is as follows −
Original array: 1,2,3,4,5 Squared array: 1,4,9,16,25
Example: Arrow functions in Class
Arrow functions (lambda expressions) can be employed as class methods or object properties in TypeScript. Below is an example of a class that includes a method composed as a lambda expression −
class Calculator { add = (x: number, y: number, z: number): number => x + y + z; } const obj = new Calculator(); console.log(`The result is: ${obj.add(4, 3, 2)}`);
On compiling, it will generate the following JavaScript code.
class Calculator { constructor() { this.add = (x, y, z) => x + y + z; } } const obj = new Calculator(); console.log(`The result is: ${obj.add(4, 3, 2)}`);
It will produce the following output −
The result is: 9
Example: Implementing Higher Order Functions
Higher-order functions which are functions that accept one or more functions as arguments and/or return a function as a result can be conveniently defined using lambda expressions in TypeScript.
const applyOp = ( x: number, y: number, operation: (a: number, b: number) => number ) => { return operation(x, y); }; const result1 = applyOp(10, 20, (a, b) => a + b); console.log(result1); const result2 = applyOp(10, 20, (a, b) => a * b); console.log(result2);
On compiling it will generate the following JavaScript code.
const applyOp = (x, y, operation) => { return operation(x, y); }; const result1 = applyOp(10, 20, (a, b) => a + b); console.log(result1); const result2 = applyOp(10, 20, (a, b) => a * b); console.log(result2);
The output of the above code example is as follows −
30 200
Arrow functions are very useful in writing the anonymous functions.