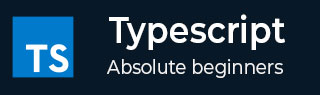
- TypeScript Basics
- TypeScript - Home
- TypeScript - Overview
- TypeScript - Environment Setup
- TypeScript - Basic Syntax
- TypeScript vs. JavaScript
- TypeScript - Features
- TypeScript - Variables
- TypeScript - let & const
- TypeScript - Operators
- TypeScript Basic Types
- TypeScript - Types
- TypeScript - Type Annotations
- TypeScript - Type Inference
- TypeScript - Numbers
- TypeScript - Strings
- TypeScript - Boolean
- TypeScript - Arrays
- TypeScript - Tuples
- TypeScript - Enums
- TypeScript - Any
- TypeScript - Never
- TypeScript - Union
- TypeScript - Literal Types
- TypeScript - Symbols
- TypeScript - null vs. undefined
- TypeScript - Type Aliases
- TypeScript Control Flow
- TypeScript - Decision Making
- TypeScript - If Statement
- TypeScript - If Else Statement
- TypeScript - Nested If Statements
- TypeScript - Switch Statement
- TypeScript - Loops
- TypeScript - For Loop
- TypeScript - While Loop
- TypeScript - Do While Loop
- TypeScript Functions
- TypeScript - Functions
- TypeScript - Function Types
- TypeScript - Optional Parameters
- TypeScript - Default Parameters
- TypeScript - Anonymous Functions
- TypeScript - Function Constructor
- TypeScript - Rest Parameter
- TypeScript - Parameter Destructuring
- TypeScript - Arrow Functions
- TypeScript Interfaces
- TypeScript - Interfaces
- TypeScript - Extending Interfaces
- TypeScript Classes and Objects
- TypeScript - Classes
- TypeScript - Objects
- TypeScript - Access Modifiers
- TypeScript - Readonly Properties
- TypeScript - Inheritance
- TypeScript - Static Methods and Properties
- TypeScript - Abstract Classes
- TypeScript - Accessors
- TypeScript - Duck-Typing
- TypeScript Advanced Types
- TypeScript - Intersection Types
- TypeScript - Type Guards
- TypeScript - Type Assertions
- TypeScript Type Manipulation
- TypeScript - Creating Types from Types
- TypeScript - Keyof Type Operator
- TypeScript - Typeof Type Operator
- TypeScript - Indexed Access Types
- TypeScript - Conditional Types
- TypeScript - Mapped Types
- TypeScript - Template Literal Types
- TypeScript Generics
- TypeScript - Generics
- TypeScript - Generic Constraints
- TypeScript - Generic Interfaces
- TypeScript - Generic Classes
- TypeScript Miscellaneous
- TypeScript - Triple-Slash Directives
- TypeScript - Namespaces
- TypeScript - Modules
- TypeScript - Ambients
- TypeScript - Decorators
- TypeScript - Type Compatibility
- TypeScript - Date Object
- TypeScript - Iterators and Generators
- TypeScript - Mixins
- TypeScript - Utility Types
- TypeScript - Boxing and Unboxing
- TypeScript - tsconfig.json
- From JavaScript To TypeScript
- TypeScript Useful Resources
- TypeScript - Quick Guide
- TypeScript - Useful Resources
- TypeScript - Discussion
TypeScript - Anonymous Functions
Anonymous Functions
In TypeScript, the anonymous functions are the functions defined without a specific name. These functions are dynamically created at runtime. We can store them in variables and call them using those variables.
We can define an anonymous function as a function expression.
We can define a function in TypeScript using function declaration and function expression.
Function declaration defined a named function. While to define an anonymous function, using function expression.
Functions expression can be named but when a function expression is defined without name it called anonymous function.
Defining Anonymous Functions with function keyword
You can see the general syntax to create a named function in TypeScript.
function funcName (param1: string, param2: number): void { // code for the function }
The funcName is the identifier in the above function. We can make the above function anonymous by removing the funcName identifier. We can define the anonymous function by using the function keyword only, and the parameter in the parentheses after that. Also, we can store the anonymous function in the variable.
You can follow the syntax below to create an anonymous function in TypeScript.
Syntax
We have converted the funcName() function to an anonymous in the below syntax:
var funcName: (param1: string, param2: string) => void = function ( param1: string, param2: string ): void { // code for anonymous function };
Now, the funcName is not a function identifier, but it stores a function. We have defined the type of the funcName variable, which is the function with two parameters of type string and return-type void. After that, we used the assignment operator to assign the anonymous function to the funcName variable.
Example
In the example below, we have defined the funcName variable and stored the anonymous function in that. You can observe how we have invoked the anonymous function using the funcName variable. We have also passed the two arguments while invoking the anonymous function using the funcName variable.
In this example, we have created an array of numbers. After that, we invoked the sort() method to sort the numbers array in the decreasing number. The sort() method takes the callback function which returns the number value to sort the array, and the callback function is the anonymous arrow function.
var funcName: (param1: string, param2: string) => void = function ( param1: string, param2: string ): void { // code for the anonymous function console.log("The value of the param1 is " + param1); console.log("The value of the param2 is " + param2); }; funcName("TutorialsPoint", "TypeScript");
On compiling, it will generate the following JavaScript code
var funcName = function (param1, param2) { // code for the anonymous function console.log("The value of the param1 is " + param1); console.log("The value of the param2 is " + param2); }; funcName("TutorialsPoint", "TypeScript");
The above code will produce the following output −
The value of the param1 is TutorialsPoint The value of the param2 is TypeScript
Defining Anonymous Function Using Arrow Function
The arrow function is another type of anonymous function. Using the arrow syntax, we can define the function without the function keyword and function identifier.
You can follow the syntax below to define the anonymous function using the arrow syntax and learn why it is called an arrow syntax.
Syntax
var test: function_Type = (parameters): return_type => { // anonymous function code }
In the above syntax, the test is a normal variable of the function type. Here, function_type is a type of arrow function. After that, () => {} is the syntax of the arrow function. Also, we can add parameters for the arrow function into the parentheses and can write code for the arrow function in the curly braces.
Example
In the example below, we have defined the test variable which stores the anonymous arrow function. The arrow function returns the number value after multiplying the value passed as a parameter.
We have invoked the arrow function using the test variable and stored its return value in the result variable.
In this example, we have created an array of numbers. After that, we invoked the sort() method to sort the numbers array in the decreasing number. The sort() method takes the callback function which returns the number value to sort the array, and the callback function is the anonymous arrow function.
var test: (valeu1: number) => number = (value1: number): number => { return 10 * value1; }; var result = test(12); console.log("The returned value from the test function is " + result);
On compiling, it will generate the following JavaScript code −
var test = function (value1) { return 10 * value1; }; var result = test(12); console.log("The returned value from the test function is " + result);
The above code will produce the following output −
The returned value from the test function is 120
Using the above syntaxes and examples, we have learned to work with anonymous functions. We will learn where anonymous functions are used while writing real-time code.
Using Anonymous Function as a Callback Function
While working with TypeScript, we often need to call a callback function when invoking any method or function. We can pass the callback function as a parameter of another function. We can use the anonymous arrow function to keep the syntax sort of the callback function.
You can follow the syntax below to use the arrow function as a callback function.
Syntax
Array.sort(()=>{ // code for the callback function })
In the above syntax, we have used the arrow function as a callback function.
Example
In this example, we have created an array of numbers. After that, we invoked the sort() method to sort the numbers array in the decreasing number. The sort() method takes the callback function which returns the number value to sort the array, and the callback function is the anonymous arrow function.
var numbers: Array<number> = [90, 64, 323, 322, 588, 668, 9, 121, 34, 1, 2]; numbers.sort((value1: number, value2: number): number => { return value1 < value2 ? 1 : -1; }); console.log(numbers);
On compiling, it will generate the following JavaScript code −
var numbers = [90, 64, 323, 322, 588, 668, 9, 121, 34, 1, 2]; numbers.sort(function (value1, value2) { return value1 < value2 ? 1 : -1 }); console.log(numbers);
The above code will produce the following output −
[ 668, 588, 323, 322, 121, 90, 64, 34, 9, 2, 1 ]
We can create the anonymous function using two ways, one is only using the function keyword, and another is using the arrow syntax. However, arrow syntax is the best as its syntax is very short.