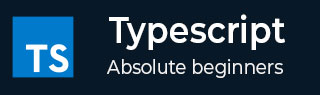
- TypeScript Basics
- TypeScript - Home
- TypeScript - Overview
- TypeScript - Environment Setup
- TypeScript - Basic Syntax
- TypeScript vs. JavaScript
- TypeScript - Features
- TypeScript - Variables
- TypeScript - let & const
- TypeScript - Operators
- TypeScript Basic Types
- TypeScript - Types
- TypeScript - Type Annotations
- TypeScript - Type Inference
- TypeScript - Numbers
- TypeScript - Strings
- TypeScript - Boolean
- TypeScript - Arrays
- TypeScript - Tuples
- TypeScript - Enums
- TypeScript - Any
- TypeScript - Never
- TypeScript - Union
- TypeScript - Literal Types
- TypeScript - Symbols
- TypeScript - null vs. undefined
- TypeScript - Type Aliases
- TypeScript Control Flow
- TypeScript - Decision Making
- TypeScript - If Statement
- TypeScript - If Else Statement
- TypeScript - Nested If Statements
- TypeScript - Switch Statement
- TypeScript - Loops
- TypeScript - For Loop
- TypeScript - While Loop
- TypeScript - Do While Loop
- TypeScript Functions
- TypeScript - Functions
- TypeScript - Function Types
- TypeScript - Optional Parameters
- TypeScript - Default Parameters
- TypeScript - Anonymous Functions
- TypeScript - Function Constructor
- TypeScript - Rest Parameter
- TypeScript - Parameter Destructuring
- TypeScript - Arrow Functions
- TypeScript Interfaces
- TypeScript - Interfaces
- TypeScript - Extending Interfaces
- TypeScript Classes and Objects
- TypeScript - Classes
- TypeScript - Objects
- TypeScript - Access Modifiers
- TypeScript - Readonly Properties
- TypeScript - Inheritance
- TypeScript - Static Methods and Properties
- TypeScript - Abstract Classes
- TypeScript - Accessors
- TypeScript - Duck-Typing
- TypeScript Advanced Types
- TypeScript - Intersection Types
- TypeScript - Type Guards
- TypeScript - Type Assertions
- TypeScript Type Manipulation
- TypeScript - Creating Types from Types
- TypeScript - Keyof Type Operator
- TypeScript - Typeof Type Operator
- TypeScript - Indexed Access Types
- TypeScript - Conditional Types
- TypeScript - Mapped Types
- TypeScript - Template Literal Types
- TypeScript Generics
- TypeScript - Generics
- TypeScript - Generic Constraints
- TypeScript - Generic Interfaces
- TypeScript - Generic Classes
- TypeScript Miscellaneous
- TypeScript - Triple-Slash Directives
- TypeScript - Namespaces
- TypeScript - Modules
- TypeScript - Ambients
- TypeScript - Decorators
- TypeScript - Type Compatibility
- TypeScript - Date Object
- TypeScript - Iterators and Generators
- TypeScript - Mixins
- TypeScript - Utility Types
- TypeScript - Boxing and Unboxing
- TypeScript - tsconfig.json
- From JavaScript To TypeScript
- TypeScript Useful Resources
- TypeScript - Quick Guide
- TypeScript - Useful Resources
- TypeScript - Discussion
TypeScript - Inheritance
Inheritance in TypeScript is a concept that allows us to reuse the properties and methods of a single class with other classes. So, it increases the code readability by allowing the reuse of the codes of different classes.
TypeScript is an object-oriented programming language, and it supports all features of the OOP. Object-oriented programming has four main pillars and inheritance is one of them.
TypeScript mainly supports two types of inheritance: single-class inheritance and multilevel inheritance. We will explore each of them in this chapter.
Single Class Inheritance
In single-class inheritance, one class inherits the properties of another class. The class that inherits the properties of the other class is called the base class or child class. The class whose properties are inherited is called a parent or superclass.
You can use the 'extend' keyword to achieve inheritance in TypeScript.
Syntax
You can follow the syntax below to use the single-class inheritance.
class Child extends Parent { // Define properties and methods for child class }
In the above syntax, we have used the 'extends' keyword between the name of the child and the parent class.
Example
In the code below, we have defined the 'Vehicle' class which contains the 'getType()' method, returning the vehicle type.
class Vehicle { getType() { return "Vehicle"; } } // Define a class Car that extends Vehicle class Car extends Vehicle { carName: string = "Innova"; getCarName() { return this.carName; } } // Create an object of Car class let car = new Car(); console.log(car.getType()); // Output: Vehicle console.log(car.getCarName()); // Output: Innova
On compiling, it will generate the following JavaScript code.
class Vehicle { getType() { return "Vehicle"; } } // Define a class Car that extends Vehicle class Car extends Vehicle { constructor() { super(...arguments); this.carName = "Innova"; } getCarName() { return this.carName; } } // Create an object of Car class let car = new Car(); console.log(car.getType()); // Output: Vehicle console.log(car.getCarName()); // Output: Innova
Output
Vehicle Innova
Super Keyword
The super keyword is used to call the constructor of the Parent class or access and call the method of the Parent class.
Syntax
You can follow the syntax below to use the super keyword to call the constructor and methods of the Parent class in the Child class.
class Child extends Parent { constructor() { super(); // To call the constructor of the parent class } super.method_name(); // To call method of the parent class }
In the above syntax, we have used the 'super()' inside the constructor of the child class to call the constructor of the parent class.
In 'super.method_name()', method_name is the name of the method of the parent class.
Example
In the code below, we have defined the Person class which contains the 'name' property, and initialized it inside the constructor() method. The display() method prints the value of the name property.
class Person { name: string; constructor(name: string) { this.name = name; } display(): void { console.log(this.name); } } // Employee class is inheriting the Person class class Employee extends Person { empCode: number; constructor(name: string, code: number) { super(name); this.empCode = code; } show(): void { super.display(); } } // Creating the object of Employee class let emp = new Employee("Sam", 123); emp.show(); // Sam
On compiling, it will generate the following JavaScript code.
class Person { constructor(name) { this.name = name; } display() { console.log(this.name); } } // Employee class is inheriting the Person class class Employee extends Person { constructor(name, code) { super(name); this.empCode = code; } show() { super.display(); } } // Creating the object of Employee class let emp = new Employee("Sam", 123); emp.show(); // Sam
Output
The above code example will produce the following result -
Sam
Method Overriding
The method overriding concept allows you to override the code of the particular method of the parent class inside the child class. This way, you can have a method with the same name in the parent and child classes, having different functionalities.
Example
In the code below, the Animal class has a move() method which works for any animal. After that, we have extended the Dog class with the Animal class. The Dog class has its own move() method, and that's how we do method overriding.
class Animal { move() { console.log("Animal is moving"); } } // Dog class is inheriting Animal class class Dog extends Animal { // Method overriding move() { console.log("Dog is moving"); } } let dog = new Dog(); dog.move(); // Output: Dog is moving
On compiling, it will generate the same JavaScript code.
Output
The above code example will produce the following result -
Dog is moving
Multilevel Inheritance
Multilevel inheritance allows you to inherit the properties of the parent class, where the parent class also inherits the other class.
Example
In the code below, the Parrot class inherits the properties and methods of the Bird class, and the Bird class inherits the properties and methods of the Animal class. However, you may have more levels of multilevel inheritance in real-time development.
class Animal { // Move method move() { console.log('This animal moves'); } } // Bird class extends Animal class class Bird extends Animal { // Bird can fly fly() { console.log('This bird flies'); } } // Parrot class inherits the Bird class class Parrot extends Bird { // Parrot can speak speak() { console.log('The parrot speaks'); } } // Creating an instance of the Parrot class let P1 = new Parrot(); P1.speak();
On compiling, it will generate the same JavaScript code.
Output
The above code example will produce the following result -
The parrot speaks
Multiple Inheritance, Hierarchical Inheritance, and Hybrid Inheritance are also supported by some object-oriented programming languages but not supported by TypeScript. You may use the interface or prototype-based inheritance to achieve multiple inheritance.