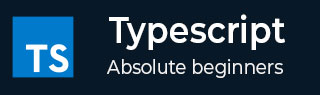
- TypeScript Basics
- TypeScript - Home
- TypeScript - Overview
- TypeScript - Environment Setup
- TypeScript - Basic Syntax
- TypeScript vs. JavaScript
- TypeScript - Features
- TypeScript - Variables
- TypeScript - let & const
- TypeScript - Operators
- TypeScript Basic Types
- TypeScript - Types
- TypeScript - Type Annotations
- TypeScript - Type Inference
- TypeScript - Numbers
- TypeScript - Strings
- TypeScript - Boolean
- TypeScript - Arrays
- TypeScript - Tuples
- TypeScript - Enums
- TypeScript - Any
- TypeScript - Never
- TypeScript - Union
- TypeScript - Literal Types
- TypeScript - Symbols
- TypeScript - null vs. undefined
- TypeScript - Type Aliases
- TypeScript Control Flow
- TypeScript - Decision Making
- TypeScript - If Statement
- TypeScript - If Else Statement
- TypeScript - Nested If Statements
- TypeScript - Switch Statement
- TypeScript - Loops
- TypeScript - For Loop
- TypeScript - While Loop
- TypeScript - Do While Loop
- TypeScript Functions
- TypeScript - Functions
- TypeScript - Function Types
- TypeScript - Optional Parameters
- TypeScript - Default Parameters
- TypeScript - Anonymous Functions
- TypeScript - Function Constructor
- TypeScript - Rest Parameter
- TypeScript - Parameter Destructuring
- TypeScript - Arrow Functions
- TypeScript Interfaces
- TypeScript - Interfaces
- TypeScript - Extending Interfaces
- TypeScript Classes and Objects
- TypeScript - Classes
- TypeScript - Objects
- TypeScript - Access Modifiers
- TypeScript - Readonly Properties
- TypeScript - Inheritance
- TypeScript - Static Methods and Properties
- TypeScript - Abstract Classes
- TypeScript - Accessors
- TypeScript - Duck-Typing
- TypeScript Advanced Types
- TypeScript - Intersection Types
- TypeScript - Type Guards
- TypeScript - Type Assertions
- TypeScript Type Manipulation
- TypeScript - Creating Types from Types
- TypeScript - Keyof Type Operator
- TypeScript - Typeof Type Operator
- TypeScript - Indexed Access Types
- TypeScript - Conditional Types
- TypeScript - Mapped Types
- TypeScript - Template Literal Types
- TypeScript Generics
- TypeScript - Generics
- TypeScript - Generic Constraints
- TypeScript - Generic Interfaces
- TypeScript - Generic Classes
- TypeScript Miscellaneous
- TypeScript - Triple-Slash Directives
- TypeScript - Namespaces
- TypeScript - Modules
- TypeScript - Ambients
- TypeScript - Decorators
- TypeScript - Type Compatibility
- TypeScript - Date Object
- TypeScript - Iterators and Generators
- TypeScript - Mixins
- TypeScript - Utility Types
- TypeScript - Boxing and Unboxing
- TypeScript - tsconfig.json
- From JavaScript To TypeScript
- TypeScript Useful Resources
- TypeScript - Quick Guide
- TypeScript - Useful Resources
- TypeScript - Discussion
TypeScript - Creating Types from Types
TypeScript allows you to create custom types from the existing or built-in types. Creating types from existing types helps you to make code more maintainable and error-resistant.
TypeScript is a statically typed language. This means that ideally, each variable, function parameter, and object property should be explicitly assigned a type.
Here we will discuss different ways to create custom types from the existing types.
Union Types
If you want to define a single variable that can store the value of multiple data types, you can use the union operator to combine multiple operators. The union operator is '|', and using that you can combine multiple types.
Syntax
You can follow the syntax below to create custom types by using the union operator.
type unionTypes = type1 | type2 | type3 | ...;
In the above syntax, type1, type2, type3, etc. are data types.
Example
In the code below, we have defined the 'StringOrNumber' custom type which is a union of the string and number data type.
The processValue() function takes the single parameter of the type 'StringOrNumber'. In the function body, we check the type of the parameter using the 'typeof' operator, and based on the string or numeric type, we print the value in the output console.
// Defining a union type type StringOrNumber = string | number; // Function that accepts a union type as an argument function processValue(value: StringOrNumber) { // Check if the value is a string or number type if (typeof value === "string") { console.log(`String: ${value}`); } else { console.log(`Number: ${value}`); } } processValue("hello"); // Output: String: hello processValue(123); // Output: Number: 123
On compiling, it will generate the following JavaScript code.
// Function that accepts a union type as an argument function processValue(value) { // Check if the value is a string or number type if (typeof value === "string") { console.log(`String: ${value}`); } else { console.log(`Number: ${value}`); } } processValue("hello"); // Output: String: hello processValue(123); // Output: Number: 123
The output of the above example code is as follows –
String: hello Number: 123
Intersection Types
The intersection operator (&) allows you to combine the multiple types in the single types. For example, if you have two interfaces or types for Business and contactDetails, and want to combine both types, you can use the intersection operator.
Syntax
You can follow the syntax below to create custom types by using the intersection operator.
type intersectionTypes = type1 & type2 & type3 & ...;
In the above syntax, we have combined multiple types using the intersection operator.
Example
In the code below, we have defined the 'Business' interface containing the name and turnover properties. We have also defined the contactDetails interface containing the email and phone properties.
After that, we combined both types using the intersection operator and stored them in the 'BusinessContact' type. Next, we have defined the object of the type 'BusinessContact', and logged it in the console.
// Defining a business type interface Business { name: string; turnover: number; } // Defining a contact details type interface ContactDetails { email: string; phone: string; } // Intersection of two types type BusinessContact = Business & ContactDetails; // Creating an object of the type BusinessContact let contact: BusinessContact = { name: "EnviroFront", turnover: 5000000, email: "abc@gmail.com", phone: "1234567890" }; console.log(contact);
On compiling, it will generate the following JavaScript code.
// Creating an object of the type BusinessContact let contact = { name: "EnviroFront", turnover: 5000000, email: "abc@gmail.com", phone: "1234567890" }; console.log(contact);
The output of the above example code is as follows –
{ name: 'EnviroFront', turnover: 5000000, email: 'abc@gmail.com', phone: '1234567890' }
Utility types
TypeScript contains multiple utility types, which makes it easy to transform the particular types and create new types. Let's look at some of the utility types with examples.
Syntax
You can follow the syntax below to use any utility type.
Utility_type_name<type or interface>
In the above syntax, 'utility_type_name' can be replaced with 'Partial', 'Pick', 'Record', etc. based on which utility type you are using. Here, 'type' or 'interface' is the name of the interface from which you want to create a new custom type.
Partial Utility Type
The partial utility type makes all properties of the interface or type option. So, you can create a new type having all properties optional from the existing type.
Example
In the code below, we have defined the 'Todo' interface. After that, we used the Partial utility type to create a new type using the Todo interface by making all properties of it optional.
Next, we have defined the object of the 'OptionalTodo' type, which contains only the 'title' property, as the 'description' property is optional.
// Defining an interface for Todo list interface Todo { title: string; description: string; } // Defining a custom type using the Partial utility type type OptionalTodo = Partial<Todo>; // Creating an object of type OptionalTodo let todo: OptionalTodo = { title: "Buy milk" }; // 'description' is optional console.log(todo);
On compiling, it will generate the following JavaScript code.
// Creating an object of type OptionalTodo let todo = { title: "Buy milk" }; // 'description' is optional console.log(todo);
The output of the above example code is as follows –
{ title: 'Buy milk' }
Pick Utility Type
The Pick utility type allows one to pick a subset of properties from the existing types. Let's understand it via the example below.
Example
In the code below, we have defined the 'ToDo' interface and used the Pick utility type to create a new type with only the 'title' property from the 'ToDo' interface. Here, the 'ToDoPick' type contains only the 'title' property.
// Defining an interface for Todo list interface Todo { title: string; description: string; } // Defining a custom type using the Pick utility type type TodoPick = Pick<Todo, "title">; let myTodo: TodoPick = { title: "Write a code" }; console.log(myTodo.title);
On compiling, it will generate the following JavaScript code.
let myTodo = { title: "Write a code" }; console.log(myTodo.title);
The output of the above example code is as follows –
Write a code
Typeof Type Operator
The typeof operator is used to get the type of the particular variable. You can use the typeOf variable to extract the data type of the particular variable, object, function, etc., and use the data type for other variables.
Syntax
You can follow the syntax below to create custom types by using the typeof type operator.
Typeof variable;
In the above syntax, 'variable' can be an object, function, etc.
Example
In the code below, we have defined the person object containing the name and gender properties. After that, we used the 'typeof' operator to get the type of the person object and stored it in the 'employee' type variable.
After that, we have defined the 'employee1' object of the 'employee' type, which contains the name and gender properties.
// Defining a person object const person = { name: "John", gender: "male" }; // Defining a type employee type employee = typeof person; // Type is { url: string; timeout: number; } // Defining an employee object let employee1: employee = { name: "Sbv", gender: "male" }; console.log(employee1);
On compiling, it will generate the following JavaScript code.
// Defining a person object const person = { name: "John", gender: "male" }; // Defining an employee object let employee1 = { name: "Sak", gender: "male" }; console.log(employee1);
The output is as follows –
{ name: 'Sak', gender: 'male' }
Creating a custom type allows you to reuse the existing types and write maintainable code. You may use the union or intersection operator if you want to provide an optional type or combine multiple types. Furthermore, you can use the utility types and typeof operator to create custom types from the existing types.