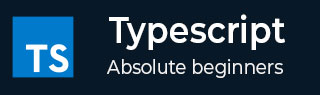
- TypeScript Basics
- TypeScript - Home
- TypeScript - Overview
- TypeScript - Environment Setup
- TypeScript - Basic Syntax
- TypeScript vs. JavaScript
- TypeScript - Features
- TypeScript - Variables
- TypeScript - let & const
- TypeScript - Operators
- TypeScript Basic Types
- TypeScript - Types
- TypeScript - Type Annotations
- TypeScript - Type Inference
- TypeScript - Numbers
- TypeScript - Strings
- TypeScript - Boolean
- TypeScript - Arrays
- TypeScript - Tuples
- TypeScript - Enums
- TypeScript - Any
- TypeScript - Never
- TypeScript - Union
- TypeScript - Literal Types
- TypeScript - Symbols
- TypeScript - null vs. undefined
- TypeScript - Type Aliases
- TypeScript Control Flow
- TypeScript - Decision Making
- TypeScript - If Statement
- TypeScript - If Else Statement
- TypeScript - Nested If Statements
- TypeScript - Switch Statement
- TypeScript - Loops
- TypeScript - For Loop
- TypeScript - While Loop
- TypeScript - Do While Loop
- TypeScript Functions
- TypeScript - Functions
- TypeScript - Function Types
- TypeScript - Optional Parameters
- TypeScript - Default Parameters
- TypeScript - Anonymous Functions
- TypeScript - Function Constructor
- TypeScript - Rest Parameter
- TypeScript - Parameter Destructuring
- TypeScript - Arrow Functions
- TypeScript Interfaces
- TypeScript - Interfaces
- TypeScript - Extending Interfaces
- TypeScript Classes and Objects
- TypeScript - Classes
- TypeScript - Objects
- TypeScript - Access Modifiers
- TypeScript - Readonly Properties
- TypeScript - Inheritance
- TypeScript - Static Methods and Properties
- TypeScript - Abstract Classes
- TypeScript - Accessors
- TypeScript - Duck-Typing
- TypeScript Advanced Types
- TypeScript - Intersection Types
- TypeScript - Type Guards
- TypeScript - Type Assertions
- TypeScript Type Manipulation
- TypeScript - Creating Types from Types
- TypeScript - Keyof Type Operator
- TypeScript - Typeof Type Operator
- TypeScript - Indexed Access Types
- TypeScript - Conditional Types
- TypeScript - Mapped Types
- TypeScript - Template Literal Types
- TypeScript Generics
- TypeScript - Generics
- TypeScript - Generic Constraints
- TypeScript - Generic Interfaces
- TypeScript - Generic Classes
- TypeScript Miscellaneous
- TypeScript - Triple-Slash Directives
- TypeScript - Namespaces
- TypeScript - Modules
- TypeScript - Ambients
- TypeScript - Decorators
- TypeScript - Type Compatibility
- TypeScript - Date Object
- TypeScript - Iterators and Generators
- TypeScript - Mixins
- TypeScript - Utility Types
- TypeScript - Boxing and Unboxing
- TypeScript - tsconfig.json
- From JavaScript To TypeScript
- TypeScript Useful Resources
- TypeScript - Quick Guide
- TypeScript - Useful Resources
- TypeScript - Discussion
TypeScript - Intersection Types
In TypeScript, an intersection type combines multiple types into one. Although intersection and union types in TypeScript are similar, they are used in very different ways. A type that combines different types into one is called an intersection type. This enables you to combine many types to produce a single type with all the necessary attributes. Members from each of the provided types will be present in an object of this type. The intersection type is made using the '&' operator.
When two types intersect in TypeScript, the intersection type will inherit the characteristics of both intersecting types. Take caution when combining types that share property names with different kinds.
Syntax
We can write the below syntax to create an Intersection type in TypeScript.
type intersepted_Type = Type1 & Type2;
Example
In the example below, we have created two interfaces named "Book" and "Author". Now inside the Book, we have created two fields named "book_id", which is of number type, and "book_name", which is of string type. And inside the Author, we have also created two fields named "author_id", which is of number type, and "author_name", which is of string type. Next, we intersected the Book and Author interface and stored it into intersected_types. Finally, values are retrieved from an object of the intersection type created.
interface Book { book_id: number book_name: string } interface Author { author_id: number author_name: string } type intersected_type = Book & Author let intersected_type_object1: intersected_type = { book_id: 101, book_name: 'Typescript is Awesome', author_id: 202, author_name: 'Tutorialspoint!', } console.log('Book Id: ' + intersected_type_object1.book_id) console.log('Book name: ' + intersected_type_object1.book_name) console.log('Author Id: ' + intersected_type_object1.author_id) console.log('Author name: ' + intersected_type_object1.author_name)
On compiling, it will generate the following JavaScript code −
var intersected_type_object1 = { book_id: 101, book_name: 'Typescript is Awesome', author_id: 202, author_name: 'Tutorialspoint!' }; console.log('Book Id: ' + intersected_type_object1.book_id); console.log('Book name: ' + intersected_type_object1.book_name); console.log('Author Id: ' + intersected_type_object1.author_id); console.log('Author name: ' + intersected_type_object1.author_name);
Output
The above code will produce the following output –
Book Id: 101 Book name: Typescript is Awesome Author Id: 202 Author name: Tutorialspoint!
As users can see in the output, all the values of two different interfaces are combined and displayed.
Intersection types are Associative and Commutative
The commutative property indicates that an equation's factors can be freely rearranged without altering the equation's outcome.
commutative property: (A & B) = (B & A)
Associative property asserts that altering how integers are grouped during an operation will not affect the equation's solution.
associative property: (A & B) & C = A & (B & C)
When we cross two or more kinds, it doesn't matter what order they are in. The 'typeof' operator is used to verify that the attributes of the intersected objects are also the same, regardless of how the items are intersected or in what order.
Example
As we can see in the below example, here we have created three interfaces named "Student", "Class", and "Subject". Now inside the Student, we have created two fields called "student_id", which is of number type, and "sudent_name", which is of string type. Inside the "Class" instance, we have also created two fields named "class_id", which is of number type, and "class_name", which is of string type. Inside the "Subject" instance, we have also created two fields named "subject_id", which is of number type, and "subject_name", which is of string type.
Next, we intersected the Book, Author, and Subject interface using associative property and stored it into intersected types. After that, values are retrieved from an object of the intersection type created. Finally, we checked the objects using the typeof operator and logged in to the console.
interface Student { student_id: number student_name: string } interface Class { class_id: number class_name: string } interface Subject { subject_id: number subject_name: string } type intersected_type_1 = (Student & Class) & Subject type intersected_type_2 = Student & (Class & Subject) let intersected_type_object1: intersected_type_1 = { student_id: 101, student_name: 'Typescript', class_id: 10, } let intersected_type_object2: intersected_type_2 = { student_id: 102, student_name: 'Typescript2', class_id: 11, } console.log(typeof intersected_type_object1 === typeof intersected_type_object2)
On compiling, it will generate the following JavaScript code −
var intersected_type_object1 = { student_id: 101, student_name: 'Typescript', class_id: 10 }; var intersected_type_object2 = { student_id: 102, student_name: 'Typescript2', class_id: 11 }; console.log(typeof intersected_type_object1 === typeof intersected_type_object2);
Output
The above code will produce the following output −
true
As users can see in the output, both the attributes of the objects are identical, showing the true value.