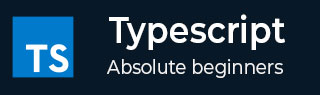
- TypeScript Basics
- TypeScript - Home
- TypeScript - Overview
- TypeScript - Environment Setup
- TypeScript - Basic Syntax
- TypeScript vs. JavaScript
- TypeScript - Features
- TypeScript - Variables
- TypeScript - let & const
- TypeScript - Operators
- TypeScript Basic Types
- TypeScript - Types
- TypeScript - Type Annotations
- TypeScript - Type Inference
- TypeScript - Numbers
- TypeScript - Strings
- TypeScript - Boolean
- TypeScript - Arrays
- TypeScript - Tuples
- TypeScript - Enums
- TypeScript - Any
- TypeScript - Never
- TypeScript - Union
- TypeScript - Literal Types
- TypeScript - Symbols
- TypeScript - null vs. undefined
- TypeScript - Type Aliases
- TypeScript Control Flow
- TypeScript - Decision Making
- TypeScript - If Statement
- TypeScript - If Else Statement
- TypeScript - Nested If Statements
- TypeScript - Switch Statement
- TypeScript - Loops
- TypeScript - For Loop
- TypeScript - While Loop
- TypeScript - Do While Loop
- TypeScript Functions
- TypeScript - Functions
- TypeScript - Function Types
- TypeScript - Optional Parameters
- TypeScript - Default Parameters
- TypeScript - Anonymous Functions
- TypeScript - Function Constructor
- TypeScript - Rest Parameter
- TypeScript - Parameter Destructuring
- TypeScript - Arrow Functions
- TypeScript Interfaces
- TypeScript - Interfaces
- TypeScript - Extending Interfaces
- TypeScript Classes and Objects
- TypeScript - Classes
- TypeScript - Objects
- TypeScript - Access Modifiers
- TypeScript - Readonly Properties
- TypeScript - Inheritance
- TypeScript - Static Methods and Properties
- TypeScript - Abstract Classes
- TypeScript - Accessors
- TypeScript - Duck-Typing
- TypeScript Advanced Types
- TypeScript - Intersection Types
- TypeScript - Type Guards
- TypeScript - Type Assertions
- TypeScript Type Manipulation
- TypeScript - Creating Types from Types
- TypeScript - Keyof Type Operator
- TypeScript - Typeof Type Operator
- TypeScript - Indexed Access Types
- TypeScript - Conditional Types
- TypeScript - Mapped Types
- TypeScript - Template Literal Types
- TypeScript Generics
- TypeScript - Generics
- TypeScript - Generic Constraints
- TypeScript - Generic Interfaces
- TypeScript - Generic Classes
- TypeScript Miscellaneous
- TypeScript - Triple-Slash Directives
- TypeScript - Namespaces
- TypeScript - Modules
- TypeScript - Ambients
- TypeScript - Decorators
- TypeScript - Type Compatibility
- TypeScript - Date Object
- TypeScript - Iterators and Generators
- TypeScript - Mixins
- TypeScript - Utility Types
- TypeScript - Boxing and Unboxing
- TypeScript - tsconfig.json
- From JavaScript To TypeScript
- TypeScript Useful Resources
- TypeScript - Quick Guide
- TypeScript - Useful Resources
- TypeScript - Discussion
TypeScript - Indexed Access Types
In TypeScript, Indexed access types are also known as lookup types. It is used to access the type of property of another type. It is a powerful way and helpful to access the type properties of the complex type structure. This feature allows you to use the type system more effectively, ensuring type safety in scenarios involving property access and manipulation.
Syntax
You can follow the syntax below to use the index access types in TypeScript.
type new_type = existing_type[key];
In the above syntax, 'type' is a keyword to define a new type.
'existing_type' is an existing type.
The 'key' is a property of the existing_type whose type we need to get.
Examples
Let's understand the indexed access types with the help of some examples in TypeScript.
Example: Accessing Object Property Types
Indexed access types are very useful when you need to get the type of the specific property of the existing type.
In the code below, we have defined the User type which contains the id, and age properties of the number type and name property of the string type.
The User['name'] is used to access the type of the 'name' property of the User type, which is a string.
Next, we have defined the userName variable whose type is 'UserNameType' (string). If you try to assign a number to the userName variable, it will throw an error.
type User = { id: number; name: string; age: number; }; // Access the type of 'name' property from User type UserNameType = User['name']; // type is string let userName: UserNameType; userName = "Alice"; // Ok console.log(userName); // Alice // userName = 123; // Error: Type 'number' is not assignable to type 'string'.
On compiling, it will generate the following JavaScript code.
let userName; userName = "Alice"; // Ok console.log(userName); // Alice // userName = 123; // Error: Type 'number' is not assignable to type 'string'.
Output
Alice
Example: Accessing Nested Object Property Types
Indexed access types are more useful when you need to get the type of any property of the complex type structure.
In the code below, the 'Product' type has id and details properties. The details property is a nested type containing the name and price properties.
We used the Product['details']['price'] to access the type of the 'price' property of the 'details' property, which is a number.
type Product = { id: number; details: { name: string; price: number; }; }; // Access the type of the nested 'price' property type ProductPriceType = Product['details']['price']; // type is number let price: ProductPriceType; price = 19.99; // Correct usage console.log(price); // Output: 19.99 // price = "20.00"; // Error: Type 'string' is not assignable to type 'number'.
On compiling, it will generate the following JavaScript code.
let price; price = 19.99; // Correct usage console.log(price); // Output: 19.99 // price = "20.00"; // Error: Type 'string' is not assignable to type 'number'.
Output
19.99
Example: Using the keyof operator with indexed access types
This example is similar to the first example. Here, we have used the 'keyof' operator to access all keys of the User type.
When you use the Keyof operator with the type and use it as an index value, it gets the union of type of all properties of the object. Here, 'keyof User' gives us the number | string, which is a type of all properties of the User type.
type User = { id: number; name: string; age: number; }; // Access the type of 'name' property from User type UserNameType = User[keyof User]; // type is number | string let userName: UserNameType; userName = "Hello"; // Correct usage console.log(userName); // Output: Hello userName = 123; // Correct usage console.log(userName); // Output: 123
On compiling, it will generate the following JavaScript code.
let userName; userName = "Hello"; // Correct usage console.log(userName); // Output: Hello userName = 123; // Correct usage console.log(userName); // Output: 123
Output
Hello 123
Example: Indexed Access with Arrays
When you want to access the type of the array elements, you can use the 'number' as an index.
In the code below, we have defined the StringArray type, which contains the array of the string.
The 'StringArray[number]' gets the type of the array element, which is a string.
type StringArray = string[]; // Access the type of an array element type ElementType = StringArray[number]; // type is string let element: ElementType; element = "Hello"; // Correct usage console.log(element); // element = 123; // Error: Type 'number' is not assignable to type 'string'.
On compiling, it will generate the following JavaScript code.
let element; element = "Hello"; // Correct usage console.log(element); // element = 123; // Error: Type 'number' is not assignable to type 'string'.
Output
Hello
This way you can access the type of the properties of the existing types using the indexed access type. You can also use the 'number' property to access the type of the array elements.