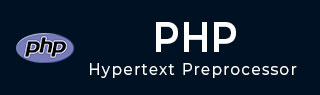
- PHP Tutorial
- PHP - Home
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP Operators
- PHP - Operators
- PHP - Arithmatic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP Arrays
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP AJAX
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP XML
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - JSON
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
- PHP Useful Resources
- PHP - Questions & Answers
- PHP - Useful Resources
- PHP - Discussion
PHP - Write File
PHP’s built-in function library provides two functions to perform write operations on a file stream. These functions are fwrite() and fputs().
To be able to write data in a file, it must be opened in write mode (w), append mode (a), read/write mode (r+ or w+) or binary write/append mode (rb+, wb+ or wa).
The fputs() Function
The fputs() function writes a string into the file opened in a writable mode.
fputs(resource $stream, string $string, int $length)
Here, the $stream parameter is a handle to a file opened in a writable mode. The $string parameter is the data to be written, and $length is an optional parameter that specifies the maximum number of bytes to be written.
The fputs() function returns the number of bytes written, or false if the function is unsuccessful.
Example
The following code opens a new file, writes a string in it, and returns the number of bytes written.
<?php $fp = fopen("hello.txt", "w"); $bytes = fputs($fp, "Hello World\n"); echo "bytes written: $bytes"; fclose($fp); ?>
It will produce the following output −
bytes written: 12
Example
If you need to add text in an earlier existing file, it must be opened in append mode (a). Let us add one more string in the same file in previous example.
<?php $fp = fopen("hello.txt", "a"); $bytes = fputs($fp, "Hello PHP"); echo "bytes written: $bytes"; fclose($fp); ?>
If you open the "hello.txt" file in a text editor, you should see both the lines in it.
Example
In the following PHP script, an already existing file (hello.txt) is read line by line in a loop, and each line is written to another file (new.txt)
It is assumed thar "hello.txt" consists of following text −
Hello World TutorialsPoint PHP Tutorials
Here is the PHP code to create a copy of an existing file −
<?php $file = fopen("hello.txt", "r"); $newfile = fopen("new.txt", "w"); while(! feof($file)) { $str = fgets($file); fputs($newfile, $str); } fclose($file); fclose($newfile); ?>
The newly created "new.txt" file should have exactly the same contents.
The fwrite() Function
The frwrite() function is a counterpart of fread() function. It performs binary-safe write operations.
fwrite(resource $stream, string $data, ?int $length = null): int|false
Here, the $stream parameter is a resource pointing to the file opened in a writable mode. Data to be written to the file is provided in the $data parameter. The optional $length parameter may be provided to specify the number of bytes to be written. It should be int, writing will stop after length bytes have been written or the end of data is reached, whichever comes first.
The fwrite() function returns the number of bytes written, or false on failure along with E_WARNING.
Example
The following program opens a new file, performs write operation and displays the number of bytes written.
<?php $file = fopen("/PhpProject/sample.txt", "w"); echo fwrite($file, "Hello Tutorialspoint!!!!!"); fclose($file); ?>
Example
In the example code given below, an existing file "welcome.png" in opened in binary read mode. The fread() function is used to read its bytes in "$data" variable, and in turn written to another file "new.png" −
<?php $name = "welcome.png"; $file = fopen($name, "rb"); $newfile = fopen("new.png", "wb"); $size = filesize($name); $data = fread($file, $size); fwrite($newfile, $data, $size); fclose($file); fclose($newfile); ?>
Run the above code. The current directory should now have a copy of the existing "welcome.png" file.