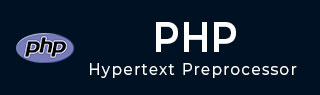
- PHP Tutorial
- PHP - Home
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Features
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP Operators
- PHP - Operators
- PHP - Arithmatic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - Loop Types
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP Arrays
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP AJAX
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP XML
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - JSON
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
- PHP Useful Resources
- PHP - Questions & Answers
- PHP - Quick Guide
- PHP - Useful Resources
- PHP - Discussion
PHP - Object Iteration
A foreach loop may be employed to iterate through all the publicly visible members of an object of a PHP class. This feature has been available in versions of PHP 5 onwards. You can of course access the list of private properties inside an instance method. PHP also defines Iterator interface which can be used for the purpose.
Using foreach Loop
In the example below, the public properties of the class are listed with the use of foreach loop.
Example
<?php class myclass { private $var; protected $var1; public $x, $y, $z; public function __construct() { $this->var="Hello World"; $this->var1=array(1,2,3); $this->x=100; $this->y=200; $this->z=300; } } $obj = new myclass(); foreach($obj as $key => $value) { print "$key => $value\n"; } ?>
It will produce the following output −
x => 100 y => 200 z => 300
Note that only the public members are accessible outside the class. If the class includes a method, all the members (public, private or protected) can be traversed with a foreach loop from inside it.
Let us add an iterate method in the above myclass.
public function iterate() { foreach ($this as $k=>$v) { if (is_array($v)) { var_dump($v); echo PHP_EOL; } else { echo "$k : $v". PHP_EOL; } } }
Call this instance method to get the list of all the members.
It will produce the following output −
var : Hello World array(3) { [0]=> int(1) [1]=> int(2) [2]=> int(3) } x : 100 y : 200 z : 300
Using Iterator Interface
PHP provides Iterator interface for external iterators or objects that can be iterated themselves internally. It defines following abstract methods which need to be implemented in the user defined class.
interface Iterator extends Traversable { /* Methods */ public current(): mixed public key(): mixed public next(): void public rewind(): void public valid(): bool }
The rewind() method rewinds the Iterator to the first element. This is the first method called when starting a foreach loop. It will not be executed after foreach loops.
The current() method returns the current element.
The key() method returns the key of the current element on each iteration of foreach loop.
The next() method is called after each iteration of foreach loop and moves forward to next element.
The valid() method checks if current position is valid.
Example
The following example demonstrates object iteration by implementing Iterator interface
<?php class myclass implements Iterator { private $arr = array('a','b','c'); public function rewind():void { echo "rewinding\n"; reset($this->arr); } public function current() { $var = current($this->arr); echo "current: $var\n"; return $var; } public function key() { $var = key($this->arr); echo "key: $var\n"; return $var; } public function next() : void { $var = next($this->arr); echo "next: $var\n"; # return $var; } public function valid() : bool { $key = key($this->arr); $var = ($key !== NULL && $key !== FALSE); echo "valid: $var\n"; return $var; } } $obj = new myclass(); foreach ($obj as $k => $v) { print "$k: $v\n"; } ?>
It will produce the following output −
rewinding valid: 1 current: a key: 0 0: a next: b valid: 1 current: b key: 1 1: b next: c valid: 1 current: c key: 2 2: c next: