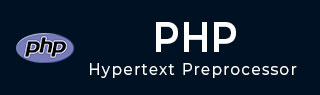
- PHP Tutorial
- PHP - Home
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP Operators
- PHP - Operators
- PHP - Arithmatic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP Arrays
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP AJAX
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP XML
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - JSON
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
- PHP Useful Resources
- PHP - Questions & Answers
- PHP - Useful Resources
- PHP - Discussion
PHP - Array Destructuring
In PHP, the term Array destructuring refers to the mechanism of extracting the array elements into individual variables. It can also be called unpacking of array. PHP’s list() construct is used to destrucrure the given array assign its items to a list of variables in one statement.
list($var1, $var2, $var3, . . . ) = array(val1, val2, val3, . . .);
As a result, val1 is assigned to $var1, val2 to $var2 and so on. Even though because of the parentheses, you may think list() is a function, but it’s not as it doesn’t have return value. PHP treats a string as an array, however it cannot be unpacked with list(). Moreover, the parenthesis in list() cannot be empty.
Instead of list(), you can also use the square brackets [] as a shortcut for destructuring the array.
[$var1, $var2, $var3, . . . ] = array(val1, val2, val3, . . .);
Example
Take a look at the following example −
<?php $marks = array(50, 56, 70); list($p, $c, $m) = $marks; echo "Physics: $p Chemistry: $c Maths: $m" . PHP_EOL; # shortcut notation [$p, $c, $m] = $marks; echo "Physics: $p Chemistry: $c Maths: $m" . PHP_EOL; ?>
It will produce the following output −
Physics: 50 Chemistry: 56 Maths: 70 Physics: 50 Chemistry: 56 Maths: 70
Destructuring an Associative Array
Before PHP 7.1.0, list() only worked on numerical arrays with numerical indices start at 0. PHP 7.1, array destructuring works with associative arrays as well.
Let us try to destructure (or unpack) the following associative array, an array with non-numeric indices.
$marks = array('p'=>50, 'c'=>56, 'm'=>70);
To destructure this array the list() statement associates each array key with a independent variable.
list('p'=>$p, 'c'=>$c, 'm'=>$m) = $marks;
Instead, you can also use the [] alternative destructuring notation.
['p'=>$p, 'c'=>$c, 'm'=>$m] = $marks;
Try and execute the following PHP script −
<?php $marks = array('p'=>50, 'c'=>56, 'm'=>70); list('p'=>$p, 'c'=>$c, 'm'=>$m) = $marks; echo "Physics: $p Chemistry: $c Maths: $m" . PHP_EOL; # shortcut notation ['p'=>$p, 'c'=>$c, 'm'=>$m] = $marks; echo "Physics: $p Chemistry: $c Maths: $m" . PHP_EOL; ?>
Skipping Array Elements
In case of an indexed array, you can skip some of its elements in assign only others to the required variables
<?php $marks = array(50, 56, 70); list($p, , $m) = $marks; echo "Physics: $p Maths: $m" . PHP_EOL; # shortcut notation [$p, , $m] = $marks; echo "Physics: $p Maths: $m" . PHP_EOL; ?>
In case of an associative array, since the indices are not incremental starting from 0, it is not necessary to follow the order of elements while assigning.
<?php $marks = array('p'=>50, 'c'=>56, 'm'=>70); list('c'=>$c, 'p'=>$p, 'm'=>$m) = $marks; echo "Physics: $p Chemistry: $c Maths: $m" . PHP_EOL; ['c'=>$c, 'm'=>$m, 'p'=>$p] = $marks; # shortcut notation echo "Physics: $p Chemistry: $c Maths: $m" . PHP_EOL; ?>
Destructuring a Nested Array
You can extend the concept of array destructuring to nested arrays as well. In the following example, the subarray nested inside is an indexed array.
<?php $marks = ['marks' => [50, 60, 70]]; ['marks' => [$p, $c, $m]] = $marks; echo "Physics: $p Chemistry: $c Maths: $m" . PHP_EOL; ?>
Destructuring works well even if the nested array is also an associative array.
<?php $marks = ['marks' => ['p'=>50, 'c'=>60, 'm'=>70]]; ['marks' => ['p'=>$p, 'c'=>$c, 'm'=>$m]] = $marks; echo "Physics: $p Chemistry: $c Maths: $m" . PHP_EOL; ?>