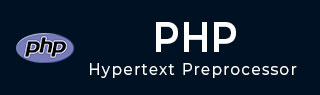
- PHP Tutorial
- PHP - Home
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Features
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP Operators
- PHP - Operators
- PHP - Arithmatic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - Loop Types
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP Arrays
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP AJAX
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP XML
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - JSON
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
- PHP Useful Resources
- PHP - Questions & Answers
- PHP - Quick Guide
- PHP - Useful Resources
- PHP - Discussion
PHP - Null Coalescing Operator
The Null Coalescing operator is one of the many new features introduced in PHP 7. The word "coalescing" means uniting many things into one. This operator is used to replace the ternary operation in conjunction with the isset() function.
Ternary Operator in PHP
PHP has a ternary operator represented by the "?" symbol. The ternary operator compares a Boolean expression and executes the first operand if it is true, otherwise it executes the second operand.
expr ? statement1 : statement2;
Example
Let us use the ternary operator to check if a certain variable is set or not with the help of the isset() function, which returns true if declared and false if not.
<?php $x = 1; $var = isset($x) ? $x : "not set"; echo "The value of x is $var"; ?>
It will produce the following output −
The value of x is 1
Now, let's remove the declaration of "x" and rerun the code −
<?php # $x = 1; $var = isset($x) ? $x : "not set"; echo "The value of x is $var"; ?>
The code will now produce the following output −
The value of x is not set
The Null Coalescing Operator
The Null Coalescing Operator is represented by the "??" symbol. It acts as a convenient shortcut to use a ternary in conjunction with isset(). It returns its first operand if it exists and is not null; otherwise it returns its second operand.
$Var = $operand1 ?? $operand2;
The first operand checks whether a certain variable is null or not (or is set or not). If it is not null, the first operand is returned, else the second operand is returned.
Example
Take a look at the following example −
<?php # $num = 10; $val = $num ?? 0; echo "The number is $val"; ?>
It will produce the following output −
The number is 0
Now uncomment the first statement that sets $num to 10 and rerun the code −
<?php $num = 10; $val = $num ?? 0; echo "The number is $val"; ?>
It will now produce the following output −
The number is 10
A useful application of Null Coalescing operator is while checking whether a username has been provided by the client browser.
Example
The following code reads the name variable from the URL. If indeed there is a value for the name parameter in the URL, a Welcome message for him is displayed. However, if not, the user is called Guest.
<?php $username = $_GET['name'] ?? 'Guest'; echo "Welcome $username"; ?>
Assuming that this script "hello.php" is in the htdocs folder of the PHP server, enter http://localhost/hello.php?name=Amar in the URL, the browser will show the following message −
Welcome Amar
If http://localhost/hello.php is the URL, the browser will show the following message −
Welcome Guest
The Null coalescing operator is used as a replacement for the ternary operator’s specific case of checking isset() function. Hence, the following statements give similar results −
<?php $username = isset($_GET['name']) ? $_GET['name'] : 'Guest'; echo "Welcome $username"; ?>
It will now produce the following output −
Welcome Guest
You can chain the "??" operators as shown below −
<?php $username = $_GET['name'] ?? $_POST['name'] ?? 'Guest'; echo "Welcome $username"; ?>
It will now produce the following output −
Welcome Guest
This will set the username to Guest if the variable $name is not set either by GET or by POST method.