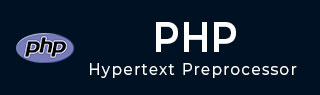
- PHP Tutorial
- PHP - Home
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Features
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP Operators
- PHP - Operators
- PHP - Arithmatic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - Loop Types
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP Arrays
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP AJAX
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP XML
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - JSON
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
- PHP Useful Resources
- PHP - Questions & Answers
- PHP - Quick Guide
- PHP - Useful Resources
- PHP - Discussion
PHP - Global Variables
In PHP, any variable that can be accessed from anywhere in a PHP script is called as a global variable. If the variable is declared outside all the functions or classes in the script, it becomes a global variable.
While global variables can be accessed directly outside a function, they aren’t automatically available inside a function.
Example
In the script below, $name is global for the function sayhello().
<?php $name = "Amar"; function sayhello() { echo "Hello " . $name; } sayhello(); ?>
However, the variable is not accessible inside the function. Hence, you will get an error message "Undefined variable $name".
Hello PHP Warning: Undefined variable $name in /home/cg/root/93427/main.php on line 5
Example
To get access within a function, you need to use the "global" keyword before the variable.
<?php $name = "Amar"; function sayhello() { GLOBAL $name; echo "Hello " . $name; } sayhello(); ?>
It will produce the following output −
Hello Amar
If a function accesses a global variable and modifies it, the modified value is available everywhere after the function call is completed.
Let us change the value of $name inside the sayhello() function and check its value after the function is called.
Example
Take a look at this following example −
<?php $name = "Amar"; function sayhello() { GLOBAL $name; echo "Global variable name: $name" .PHP_EOL; $name = "Amarjyot"; echo "Global variable name changed to: $name" .PHP_EOL; } sayhello(); echo "Global variable name after function call: $name" .PHP_EOL; ?>
It will produce the following output −
Global variable name: Amar Global variable name changed to: Amarjyot Global variable name after function call: Amarjyot
The $GLOBALS Array
PHP maintains an associative array named $GLOBALS that holds all the variables and their values declared in a global scope. The $GLOBALS array also stores many predefined variables called as superglobals, along with the user defined global variables.
Any of the global variables can also be accessed inside any function with the help of a regular syntax of accessing an arrow element. For example, the value of the global variable $name is given by $GLOBALS["name"].
Example
In the following example, two global variable $x and $y are accessed inside the addition() function.
<?php $x = 10; $y = 20; function addition() { $z = $GLOBALS['x']+$GLOBALS['y']; echo "Addition: $z" .PHP_EOL; } addition(); ?>
It will produce the following output −
Addition: 30
Example
You can also add any local variable into the global scope by adding it in the $GLOBALS array. Let us add $z in the global scope.
<?php $x = 10; $y = 20; function addition() { $z = $GLOBALS['x']+$GLOBALS['y']; $GLOBALS['z'] = $z; } addition(); echo "Now z is the global variable. Addition: $z" .PHP_EOL; ?>
It will produce the following output −
Now z is the global variable. Addition: 30
Including One PHP Script in Another
You can include one PHP script in another. Variables declared in the included script are added in the global scope of the PHP script in which it is included.
Here is "a.php" file −
<?php include 'b.php'; function addition() { $z = $GLOBALS['x']+$GLOBALS['y']; echo "Addition: $z" .PHP_EOL; } addition(); ?>
It includes "b.php" that has the $x and $y variables, so they become the global variables for the addition() function in "a.php" script.
<?php $x = 10; $y = 20; ?>
Global variables are generally used while implementing singleton patterns, and accessing registers in embedded systems and also when a variable is being used by many functions.