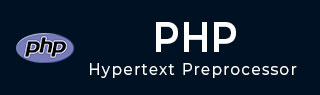
- PHP Tutorial
- PHP - Home
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP Operators
- PHP - Operators
- PHP - Arithmatic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP Arrays
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP AJAX
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP XML
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - JSON
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
- PHP Useful Resources
- PHP - Questions & Answers
- PHP - Useful Resources
- PHP - Discussion
PHP - Cloning Objects
A PHP statement such as "$obj1 = $obj2" merely creates another reference to the same object in memory. Hence, changes in attribute reflect both in original and duplicate object. The clone keyword in PHP creates a shallow copy of an object.
$obj2 = $obj1
Changes in the original object do not reflect in the shallow copy.
Example
Take a look at the following example −
<?php class foo { var $var1 = 'Hello'; } $x = new foo(); $y = $x; # reference copy echo $x->var1 . " " . $y->var1 . PHP_EOL; $x->var1 = "Hello World"; echo $x->var1 . " " . $y->var1 . PHP_EOL; ?>
It will produce the following output −
Hello Hello Hello World Hello World
In the first case, $y is just a reference copy of $x. Hence, any change in var1 property reflects in both.
However, if we declare $y as a clone of $x, then any change in the original object is not reflected in its shallow copy.
Example
Take a look at the following example −
<?php class foo { var $var1 = 'Hello World'; } $x = new foo(); # shallow copy $y = clone $x; echo $x->var1 . " " . $y->var1 . PHP_EOL; $x->var1 = "Hello PHP"; echo $x->var1 . " " . $y->var1 . PHP_EOL; ?>
It will produce the following output −
Hello World Hello World Hello PHP Hello World
Example
In the following code, myclass has one of attributes as object of address class. An object of myclass is duplicated by assignment. Any change in the value of its embedded address object is reflected in both the objects, but change in the name property is not effected in the cloned object.
<?php class address { var $city="Nanded"; var $pin="431601"; function setaddr($arg1, $arg2) { $this->city=$arg1; $this->pin=$arg2; } } class myclass { var $name="Raja"; var $obj; function setname($arg) { $this->name=$arg; } } $obj1=new myclass(); $obj1->obj=new address(); echo "original object\n"; print_r($obj1); echo "\n"; $obj2=$obj1; # reference copy $obj1->setname("Ravi"); $obj1->obj->setaddr("Mumbai", "400001"); echo "after change: Original object\n"; print_r($obj1); echo "\nCopied object\n"; print_r($obj2); ?>
It will produce the following output −
original object myclass Object ( [name] => Raja [obj] => address Object ( [city] => Nanded [pin] => 431601 ) ) after change: Original object myclass Object ( [name] => Ravi [obj] => address Object ( [city] => Mumbai [pin] => 400001 ) ) Copied object myclass Object ( [name] => Ravi [obj] => address Object ( [city] => Mumbai [pin] => 400001 ) )
Using the "clone" Keyword
In a shallow copy, any properties of the original object that are references to other variables will remain references. The clone keyword does not copy the contained objects of the copied objects.
We now create a clone of myclass object, so that $obj2 is the clone of $obj1. We change the name property of $obj1 from Raja to Ravi, and also modify the embedded address object. The property change will not reflect in its clone, but the referred address object will be changed.
Example
Take a look at the following example −
<?php class address { var $city="Nanded"; var $pin="431601"; function setaddr($arg1, $arg2) { $this->city=$arg1; $this->pin=$arg2; } } class myclass { var $name="Raja"; var $obj; function setname($arg) { $this->name=$arg; } } $obj1=new myclass(); $obj1->obj=new address(); echo "original object\n"; print_r($obj1); echo "\n"; $obj2=clone $obj1; # clone copy $obj1->setname("Ravi"); $obj1->obj->setaddr("Mumbai", "400001"); echo "after change: Original object\n"; print_r($obj1); echo "\nCopied object\n"; print_r($obj2); ?>
It will produce the following output −
original object myclass Object ( [name] => Raja [obj] => address Object ( [city] => Nanded [pin] => 431601 ) ) after change: Original object myclass Object ( [name] => Ravi [obj] => address Object ( [city] => Mumbai [pin] => 400001 ) ) Copied object myclass Object ( [name] => Raja [obj] => address Object ( [city] => Mumbai [pin] => 400001 ) )
Using __clone() Method
The clone keyword creates a shallow copy of the object. When an object is cloned, PHP will perform a shallow copy of all of the object's properties. Any properties that are references to other variables will remain references. Hence, any changes done to the original object will also appear in the cloned object.
If you wish to prevent the copied object to update automatically, we need to create a deep copy of the object with the __clone() method. It is one of the magic methods in PHP.
Once the cloning is complete, if a __clone() method is defined, then the newly created object's __clone() method will be called, to allow any necessary properties that need to be changed.
Example
In the above example, we have an object of myclass, one of its attributes $obj holding the reference to an object of address class. To achieve a deep copy, we override the __clone() magic method in myclass.
<?php class address { var $city="Nanded"; var $pin="431601"; function setaddr($arg1, $arg2) { $this->city=$arg1; $this->pin=$arg2; } } class myclass { var $name="Raja"; var $obj; function setname($arg) { $this->name=$arg; } public function __clone() { $this->obj = clone $this->obj ; } } $obj1=new myclass(); $obj1->obj=new address(); echo "original object\n"; print_r($obj1); echo "\n"; $obj2=clone $obj1; # cloned deep copy $obj1->setname("Ravi"); $obj1->obj->setaddr("Mumbai", "400001"); echo "after change: Original object\n"; print_r($obj1); echo "\nCloned object\n"; print_r($obj2); ?>
You will now see that the changes in the original object (we change the address attributes) won’t reflect in the cloned object, as the following output shows −
original object myclass Object ( [name] => Raja [obj] => address Object ( [city] => Nanded [pin] => 431601 ) ) after change: Original object myclass Object ( [name] => Ravi [obj] => address Object ( [city] => Mumbai [pin] => 400001 ) ) Cloned object myclass Object ( [name] => Raja [obj] => address Object ( [city] => Nanded [pin] => 431601 ) )