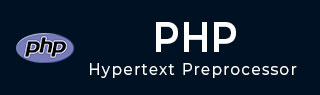
- PHP Tutorial
- PHP - Home
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Features
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP Operators
- PHP - Operators
- PHP - Arithmatic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - Loop Types
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP Arrays
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP AJAX
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP XML
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - JSON
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
- PHP Useful Resources
- PHP - Questions & Answers
- PHP - Quick Guide
- PHP - Useful Resources
- PHP - Discussion
PHP - Login Example
A typical PHP web application authenticates the user before logging in, by asking his credentials such as username and password. The credentials are then checked against the user data available with the server. In this example, the user data is available in the form of an associative array. The following PHP Login script is explained below −
HTML Form
The HTML part of the code presents a simple HTML form, that accepts username and password, and posts the data to itself.
<form action = "<?php echo htmlspecialchars($_SERVER['PHP_SELF']); ?>" method="post"> <div> <label for="username">Username:</label> <input type="text" name="username" id="name"> </div> <div> <label for="password">Password:</label> <input type="password" name="password" id="password"> </div> <section style="margin-left:2rem;"> <button type="submit" name="login">Login</button> </section> </form>
PHP Authentication
The PHP script parses the POST data, and checks if the username is present in the users array. If found, it further checks whether the password corresponds to the registered user in the array
<?php if (array_key_exists($user, $users)) { if ($users[$_POST['username']]==$_POST['password']) { $_SESSION['valid'] = true; $_SESSION['timeout'] = time(); $_SESSION['username'] = $_POST['username']; $msg = "You have entered correct username and password"; } else { $msg = "You have entered wrong Password"; } } else { $msg = "You have entered wrong user name"; } ?>
The username and the appropriate message is added to the $_SESSION array. The user is prompted with a respective message, whether the credentials entered by him are correct or not.
The Complete Code
Here is the complete code −
Login.php
<?php ob_start(); session_start(); ?> <html lang = "en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link rel="stylesheet" href="loginstyle.css"> <title>Login</title> </head> <body> <h2 style="margin-left:10rem; margin-top:5rem;">Enter Username and Password</h2> <?php $msg = ''; $users = ['user'=>"test", "manager"=>"secret", "guest"=>"abc123"]; if (isset($_POST['login']) && !empty($_POST['username']) && !empty($_POST['password'])) { $user=$_POST['username']; if (array_key_exists($user, $users)){ if ($users[$_POST['username']]==$_POST['password']){ $_SESSION['valid'] = true; $_SESSION['timeout'] = time(); $_SESSION['username'] = $_POST['username']; $msg = "You have entered correct username and password"; } else { $msg = "You have entered wrong Password"; } } else { $msg = "You have entered wrong user name"; } } ?> <h4 style="margin-left:10rem; color:red;"><?php echo $msg; ?></h4> <br/><br/> <form action = "<?php echo htmlspecialchars($_SERVER['PHP_SELF']); ?>" method="post"> <div> <label for="username">Username:</label> <input type="text" name="username" id="name"> </div> <div> <label for="password">Password:</label> <input type="password" name="password" id="password"> </div> <section style="margin-left:2rem;"> <button type="submit" name="login">Login</button> </section> </form> <p style="margin-left: 2rem;"> <a href = "logout.php" tite = "Logout">Click here to clean Session.</a> </p> </div> </body> </html>
Logout.php
To logout, the user clicks on the link to logout.php
<?php session_start(); unset($_SESSION["username"]); unset($_SESSION["password"]); echo '<h4>You have cleaned session</h4>'; header('Refresh: 2; URL = login.php'); ?>
Start the application by entering "http://localhost/login.php". Here are the different scenarios −
Correct Username and Password
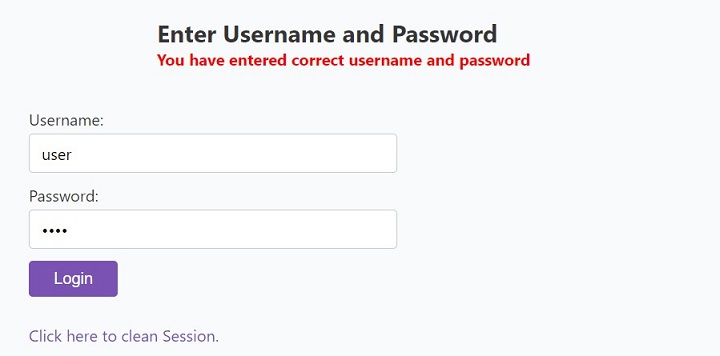
Incorrect Password
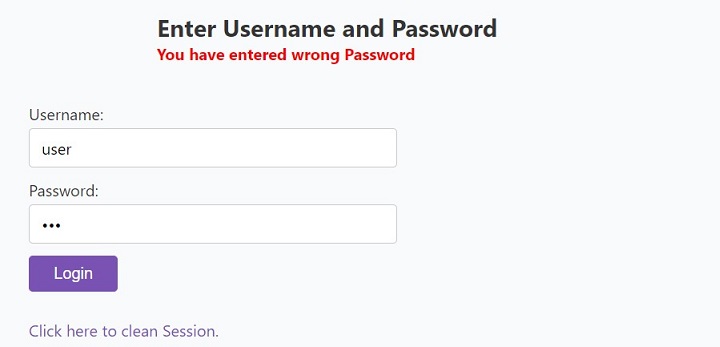
Incorrect Username
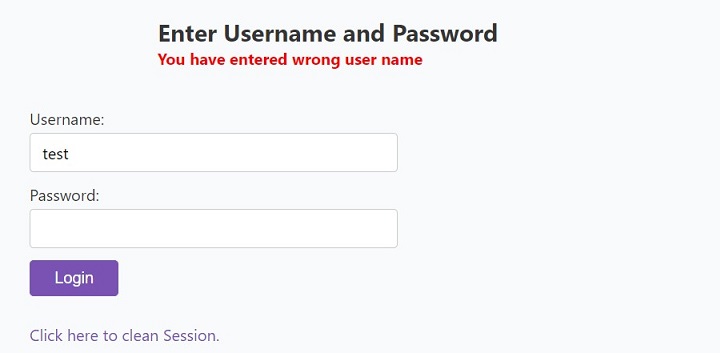
When the user clicks the link at the bottom, the session variables are removed, and the login screen reappears.