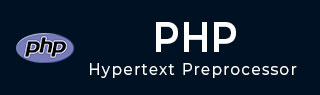
- PHP Tutorial
- PHP - Home
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Features
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP Operators
- PHP - Operators
- PHP - Arithmatic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - Loop Types
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP Arrays
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP AJAX
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP XML
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - JSON
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
- PHP Useful Resources
- PHP - Questions & Answers
- PHP - Quick Guide
- PHP - Useful Resources
- PHP - Discussion
PHP - Variable Scope
In PHP, the scope of a variable is the context within which it is defined and accessible to the extent in which it is accessible. Generally, a simple sequential PHP script that doesn’t have any loop or a function etc., has a single scope. Any variable declared inside the "<?php" and "?>" tag is available throughout the program from the point of definition onwards.
Based on the scope, a PHP variable can be any of these three types −
A variable in a main script is also made available to any other script incorporated with include or require statements.
Example
In the following example, a "test.php" script is included in the main script.
main.php
<?php $var=100; include "test.php"; ?>
test.php
<?php echo "value of \$var in test.php : " . $var; ?>
When the main script is executed, it will display the following output −
value of $var in test.php : 100
However, when the script has a user defined function, any variable inside has a local scope. As a result, a variable defined inside a function can't be accessed outside. Variables defined outside (above) the function have a global scope.
Example
Take a look at the following example −
<?php $var=100; // global variable function myfunction() { $var1="Hello"; // local variable echo "var=$var var1=$var1" . PHP_EOL; } myfunction(); echo "var=$var var1=$var1" . PHP_EOL; ?>
It will produce the following output −
var= var1=Hello var=100 var1= PHP Warning: Undefined variable $var in /home/cg/root/64504/main.php on line 5 PHP Warning: Undefined variable $var1 in /home/cg/root/64504/main.php on line 8
Note that a global variable is not automatically available within the local scope of a function. Also, the variable inside a function is not accessible outside.
The "global" Keyword
To enable access to a global variable inside local scope of a function, it should be explicitly done by using the "global" keyword.
Example
The PHP script is as follows −
<?php $a=10; $b=20; echo "Global variables before function call: a = $a b = $b" . PHP_EOL; function myfunction() { global $a, $b; $c=($a+$b)/2; echo "inside function a = $a b = $b c = $c" . PHP_EOL; $a=$a+10; } myfunction(); echo "Variables after function call: a = $a b = $b c = $c"; ?>
It will produce the following output −
Global variables before function call: a = 10 b = 20 inside function a = 10 b = 20 c = 15 Variables after function call: a = 20 b = 20 c = PHP Warning: Undefined variable $c in /home/cg/root/48499/main.php on line 12
Global variables can now be processed inside the function. Moreover, any changes made to the global variables inside the function will be reflected in the global namespace.
$GLOBALS Array
PHP stores all the global variables in an associative array called $GLOBALS. The name and value of the variables form the key-value pair.
Example
In the following PHP script, $GLOBALS array is used to access global variables −
<?php $a=10; $b=20; echo "Global variables before function call: a = $a b = $b" . PHP_EOL; function myfunction() { $c=($GLOBALS['a']+$GLOBALS['b'])/2; echo "c = $c" . PHP_EOL; $GLOBALS['a']+=10; } myfunction(); echo "Variables after function call: a = $a b = $b c = $c"; ?>
It will produce the following output −
Global variables before function call: a = 10 b = 20 c = 15 PHP Warning: Undefined variable $c in C:\xampp\htdocs\hello.php on line 12 Variables after function call: a = 20 b = 20 c =
Static Variable
A variable defined with static keyword is not initialized at every call to the function. Moreover, it retains its value of the previous call.
Example
Take a look at the following example −
<?php function myfunction() { static $x=0; echo "x = $x" . PHP_EOL; $x++; } for ($i=1; $i<=3; $i++) { echo "call to function :$i : "; myfunction(); } ?>
It will produce the following output −
call to function :1 : x = 0 call to function :2 : x = 1 call to function :3 : x = 2