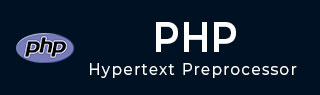
- PHP Tutorial
- PHP - Home
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP Operators
- PHP - Operators
- PHP - Arithmatic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP Arrays
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP AJAX
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP XML
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - JSON
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
- PHP Useful Resources
- PHP - Questions & Answers
- PHP - Useful Resources
- PHP - Discussion
PHP mysqli_warning_count() Function
Definition and Usage
if your last MySQLi function call executes MySQL query and if it generates any errors. The mysqli_warning_count() function counts the number of errors generated by the last executed query and, returns the result.
Syntax
mysqli_warning_count($con)
Parameters
Sr.No | Parameter & Description |
---|---|
1 |
con(Mandatory) This is an object representing a connection to MySQL Server. |
Return Values
PHP mysqli_warning_count() function returns an integer value representing the number of warnings generated during the execution of last query. If there are no warnings during the last execution this function returns 0
PHP Version
This function was first introduced in PHP Version 5 and works works in all the later versions.
Assume we have created a table named Emp as follows −
CREATE TABLE EMP( ID TINYINT, First_Name VARCHAR(50) Not NULL, Last_Name VARCHAR(10) Not NULL, Date_Of_Birth date, Salary Int(255) );
Example
Following example demonstrates the usage of the mysqli_warning_count() function (in procedural style) −
<?php //Creating a connection $con = mysqli_connect("localhost", "root", "password", "mydb"); //Inserting a record into the employee table $sql = "INSERT IGNORE into emp values(1, 'Sanjay', NULL, DATE('1981-12-05'), 2566)"; mysqli_query($con, $sql); //Number of Warnings $count = mysqli_warning_count($con); print("Number of Warnings: ".$count ."\n"); $sql = "INSERT IGNORE into emp values (15, 'Swetha', 'Yellapragada', DATE('1990-11-25'), 9986), (15, NULL, 'Prayaga', DATE('1990-11-25'), 9986)"; mysqli_query($con, $sql); //Number of Warnings $count = mysqli_warning_count($con); print("Number of Warnings: ".$count); //Closing the connection mysqli_close($con); ?>
This will produce following result −
Number of Warnings: 1 Number of Warnings: 2
Example
In object oriented style the syntax of this function is $con -> warning_count, Where, $con is the connection object −
<?php //Creating a connection $con = new mysqli("localhost", "root", "password", "mydb"); //Inserting a record into the employee table $con -> query("INSERT IGNORE into emp values(1, 'Sanjay', NULL, DATE('1981-12-05'), 2566)"); //Number of Warnings $count1 = $con->warning_count; print("Number of Warnings: ".$count1."\n"); //Inserting a record into the employee table $con -> query("INSERT IGNORE into emp values(15, 'Swetha', 'Yellapragada', DATE('1990-11-25'), 9986), (15, NULL, 'Prayaga', DATE('1990-11-25'), 9986)"); //Number of Warnings $count2 = $con->warning_count; print("Number of Warnings: ".$count2); //Closing the connection $con -> close(); ?>
This will produce following result −
Number of Warnings: 0 Number of Warnings: 2
Example
Following is another example of the function mysqli_warning_count() −
<?php //Creating a connection $con = mysqli_connect("localhost", "root", "password", "mydb"); //Warning count for proper query mysqli_query($con, "SELECT * FROM EMP"); print("No.Of Warnings (proper query): ".mysqli_warning_count($con)."\n"); //Query to DROP an unknown table mysqli_query($con, "drop table if exists WrongTable"); print("No.Of Warnings: ".mysqli_warning_count($con)."\n"); //Warnings of before last statement mysqli_query($con, "INSERT IGNORE into emp values(107, 'Sunitha', NULL, DATE('1981-12-05'), 2566)"); mysqli_query($con, "INSERT IGNORE into emp values(7, 'Mohit', 'Sharma', DATE('1981-12-05'), 2566)"); print("No.Of Warnings (if before last query contains errors): ".mysqli_warning_count($con)."\n"); //Closing the connection mysqli_close($con); ?>
This will produce following result −
Insert ID (select query): 0 Insert ID: (multiple inserts) 6 Insert ID (update query): 0 Insert ID: (table with out auto incremented key) 0
Example
<?php $servername = "localhost"; $username = "root"; $password = "password"; $dbname = "mydb"; $conn = new mysqli($servername, $username, $password, $dbname); if (!$conn->real_connect($servername, $username, $password, $dbname)) { die('Connect Error (' . mysqli_connect_errno() . ') '. mysqli_connect_error()); } print("Database connected" ."\n"); mysqli_query($conn, "CREATE TABLE sample (ID INT, Name VARCHAR(20))"); $query = "INSERT IGNORE INTO sample (id,name) VALUES( 1,'Rajesh Ramayan Kootrapally')"; mysqli_query($conn, $query); $warnings = mysqli_warning_count($conn); print("No.Of warnings in the query:".$warnings."\n"); if ($warnings) { if ($result = mysqli_query($conn, "SHOW WARNINGS")) { $row = mysqli_fetch_row($result); printf("%s (%d): %s\n", $row[0], $row[1], $row[2]); mysqli_free_result($result); } } mysqli_close($conn); ?>
This will produce following result −
Database connected No.Of warnings in the query:1 Warning (1265): Data truncated for column 'Name' at row 1