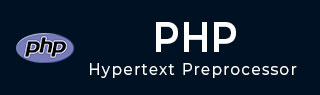
- PHP Tutorial
- PHP - Home
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP Operators
- PHP - Operators
- PHP - Arithmatic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP Arrays
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP AJAX
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP XML
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - JSON
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
- PHP Useful Resources
- PHP - Questions & Answers
- PHP - Useful Resources
- PHP - Discussion
PHP mysqli_change_user() Function
Definition and Usage
The mysqli_change_user() function accepts a connection object, user name, password and, a database name as parameters, changes the user and database in the given connection object to the specified user and database.
Syntax
mysqli_change_user($con, $user, $password, $database);
Parameters
Sr.No | Parameter & Description |
---|---|
1 |
con(Mandatory) This is an object representing a connection to MySQL Server. |
2 |
user(Optional) This is a name of a MySQL user to which you need to change. |
3 |
password(Optional) This is a password of the specified MySQL user |
3 |
database(Optional) This represents the name of the database to which you need to change. If you pass NULL as a value to this parameter, this function just changes the user without selecting the database. |
Return Values
The PHP mysqli_change_user() function returns a boolean value which is true if the database changed of successfully and false if not.
PHP Version
This function was first introduced in PHP Version 5 and works works in all the later versions.
Example
Following example demonstrates the usage of the mysqli_change_user() function (in procedural style) −
<?php //Creating a connection $con = mysqli_connect("localhost", "root", "password", "mydb"); $res = mysqli_change_user($con, "Tutorialspoint", "abc123", "mydb"); if($res){ print("User changed successfully"); }else{ print("Sorry Couldn't change the user"); } //Closing the connection mysqli_close($con); ?>
This will produce following result −
User changed successfully
Example
In object oriented style the syntax of this function is $con->change_user(); Following is the example of this function in object oriented style $minus;
<?php $host = "localhost"; $username = "root"; $passwd = "password"; $dbname = "mydb"; //Creating a connection $con = new mysqli($host, $username, $passwd, $dbname); $res = $con->change_user("Tutorialspoint", "abc123", "mydb"); if($res){ print("User changed successfully"); }else{ print("Sorry couldn't change the user"); } //Closing the connection $res = $con -> close(); ?>
This will produce following result −
User changed successfully
Example
You can verify the database name after change as shown below −
//Creating a connection $con = mysqli_connect("localhost", "root", "password", "mydb"); //Changing the database $res = mysqli_change_user($con, "Tutorialspoint", "abc123", "mydb"); $list = mysqli_query($con, "SELECT DATABASE()"); if($list) { $row = mysqli_fetch_row($list); print("Current Database: ". $row[0]); } //Closing the connection mysqli_close($con); ?>
This will produce following result −
Current Database: mydb
Example
<?php $connection = mysqli_connect("localhost","root","password","mydb"); if (mysqli_connect_errno($connection)){ echo "Failed to connect to MySQL: " . mysqli_connect_error(); } mysqli_change_user($connection, "myuser", "abc123", "sampledb"); mysqli_close($connection); ?>