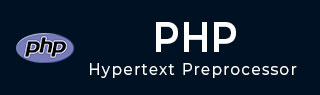
- PHP Tutorial
- PHP - Home
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Features
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP Operators
- PHP - Operators
- PHP - Arithmatic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - Loop Types
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP Arrays
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP AJAX
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP XML
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - JSON
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
- PHP Useful Resources
- PHP - Questions & Answers
- PHP - Quick Guide
- PHP - Useful Resources
- PHP - Discussion
PHP - Ds Set::contains() Function
The PHP Ds\Set::contains() function determines whether the current set contains all the specified values. This function returns a boolean value 'true' if the given value is present in this set; 'false' otherwise.
The values of the type object are supported. If an object implements Ds\Hashable, its equals function will determine whether two objects are equal. If an object doesn't implement Ds\Hashable, objects are only equal if they are references to the same instance.
Syntax
Following is the syntax of the PHP Ds\Set::contains() function −
public Ds\Set::contains(mixed ...$values): bool
Parameters
Following is the parameter of this function −
- values − The values need to be checked.
Return value
This function returns 'true' if any of the given values are persent in the set, and 'false' otherwise.
Example 1
The following is the basic example of the PHP Ds\Set::contains() function −
<?php $set = new \Ds\Set([1, 2, 3, 4, 5]); echo "The set elements are: \n"; var_dump($set); $val = 5; echo "The value need to determine: ". $val; #using contains() function echo "\nIs set contains value " .$val. "? "; var_dump($set->contains($val)); ?>
Output
The above program generates the following output −
The set elements are: object(Ds\Set)#1 (5) { [0]=> int(1) [1]=> int(2) [2]=> int(3) [3]=> int(4) [4]=> int(5) } The value need to determine: 5 Is set contains value 5? bool(true)
Example 2
The following is another example of the PHP Ds\Set::contains() function. We use this function to check whether this set (["Tutorials", "Point", "India"]) contains the specified elements "Hello" or not −
<?php $set = new \Ds\Set(["Tutorials", "Point", "India"]); echo "The set elements are: \n"; var_dump($set); $val = "Hello"; echo "The value need to determine: ". $val; #using contains() function echo "\nIs set contains value " .$val. "? "; var_dump($set->contains($val)); ?>
Output
After executing the above program, it will display the following output −
The set elements are: object(Ds\Set)#1 (3) { [0]=> string(9) "Tutorials" [1]=> string(5) "Point" [2]=> string(5) "India" } The value need to determine: Hello Is set contains value Hello? bool(false)
Example 3
In the example below, we are using the contains() method to check whether this set (['a', 1, 'b', 2, 'c', 3, 'd', 4, 'e', 5]) contains the specified values "1", "a", "e", "10", and "g" or not −
<?php $set = new \Ds\Set(['a', 1, 'b', 2, 'c', 3, 'd', 4, 'e', 5]); echo "The set elements are: \n"; var_dump($set); $val1 = 1; $val2 = "a"; $val3 = "e"; $val4 = 10; $val5 = "g"; echo "The values need to determine: ". $val1 . ", ". $val2 . ", ". $val3 . ", ". $val4 . ", ". $val5; #using contains() function echo "\nIs set contains value " .$val1. "? "; var_dump($set->contains($val1)); echo "\nIs set contains value " .$val2. "? "; var_dump($set->contains($val2)); echo "\nIs set contains value " .$val3. "? "; var_dump($set->contains($val3)); echo "\nIs set contains value " .$val4. "? "; var_dump($set->contains($val4)); echo "\nIs set contains value " .$val5. "? "; var_dump($set->contains($val5)); ?>
Output
On executing the above program, it will display the following output −
The set elements are: object(Ds\Set)#1 (10) { [0]=> string(1) "a" [1]=> int(1) [2]=> string(1) "b" [3]=> int(2) [4]=> string(1) "c" [5]=> int(3) [6]=> string(1) "d" [7]=> int(4) [8]=> string(1) "e" [9]=> int(5) } The values need to determine: 1, a, e, 10, g Is set contains value 1? bool(true) Is set contains value a? bool(true) Is set contains value e? bool(true) Is set contains value 10? bool(false) Is set contains value g? bool(false)