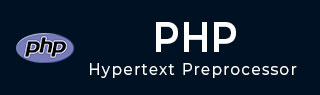
- PHP Tutorial
- PHP - Home
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Features
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP Operators
- PHP - Operators
- PHP - Arithmatic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - Loop Types
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP Arrays
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP AJAX
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP XML
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - JSON
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
- PHP Useful Resources
- PHP - Questions & Answers
- PHP - Quick Guide
- PHP - Useful Resources
- PHP - Discussion
PHP - Ds\Collection::toArray() Function
The PHP Ds\Collection::toArray() function is used to convert the current collection into an array and returns the collection elements in an array format. Whatever the initial input such as set, deck, stack, vector, etc., this function converts them directly into an array format.
In PHP, an array is a special variable that can hold multiple values under a single name. You can access the values by referring to an index number or name.
Syntax
Following is the syntax of the PHP Ds\Collection::toArray() function −
abstract public array Ds\Collection::toArray( void )
Parameters
This function does not accept any parameter.
Return value
This function returns the elements of the current collection in array format.
Example 1
The following program demonstrates the usage of the PHP Ds\Collection::toArray() function −
<?php $collection = new \Ds\Vector([10, 15, 20, 25, 30]); echo "The vector elements are: \n"; print_r($collection); #using the toArray() function echo "An array elements are: \n"; var_dump($collection->toArray()); ?>
Output
The above program produces the following output −
The vector elements are: Ds\Vector Object ( [0] => 10 [1] => 15 [2] => 20 [3] => 25 [4] => 30 ) An array elements are: array(5) { [0]=> int(10) [1]=> int(15) [2]=> int(20) [3]=> int(25) [4]=> int(30) }
Example 2
Following is another example of the PHP Ds\Collection::toArray() function. We use this function to convert the current collection into an array format −
<?php $set = new \Ds\Set(); $set->add('Sunday'); $set->add('Monday'); $set->add('Tuesday'); $set->add('Wednesday'); $set->add('Thursday'); $set->add('Friday'); $set->add('Saturday'); echo "The set elements are: \n"; print_r($set); #using toArray() function $res=$set->toArray(); echo "An array elements are: \n"; print_r($res); ?>
Output
After executing the above program, it will display the following output −
The set elements are: Ds\Set Object ( [0] => Sunday [1] => Monday [2] => Tuesday [3] => Wednesday [4] => Thursday [5] => Friday [6] => Saturday ) An array elements are: Array ( [0] => Sunday [1] => Monday [2] => Tuesday [3] => Wednesday [4] => Thursday [5] => Friday [6] => Saturday )
Example 3
In the below example, we use the PHP Ds\Collection::toArray() function to convert the current collection into an array format −
<?php $seq = new \Ds\Vector(["test_string", 1525, false]); echo "The vector elements are: \n"; var_dump($seq); #using toArray() function $res = $seq->toArray(); echo "An array elements are: \n"; var_dump($res); ?>
Output
Once the above program is executed, it will generate the following output −
The vector elements are: object(Ds\Vector)#1 (3) { [0]=> string(11) "test_string" [1]=> int(1525) [2]=> bool(false) } An array elements are: array(3) { [0]=> string(11) "test_string" [1]=> int(1525) [2]=> bool(false) }
Example 4
Let's create another collection named stack([]) and use the same toArray() function to convert it into the array format −
<?php $stack = new \Ds\Stack(); $stack->push(2412); $stack->push(1262); $stack->push(457, 57, 58584); $stack->push(...[558, 6566]); echo "Stack elements are: \n"; print_r($stack); #using toArray() function $res=$stack->toArray(); echo "An array elements are: \n"; print_r($res); ?>
Output
On executing the above program, it displays the following output −
Stack elements are: Ds\Stack Object ( [0] => 6566 [1] => 558 [2] => 58584 [3] => 57 [4] => 457 [5] => 1262 [6] => 2412 ) An array elements are: Array ( [0] => 6566 [1] => 558 [2] => 58584 [3] => 57 [4] => 457 [5] => 1262 [6] => 2412 )