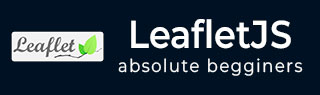
- LeafletJS Tutorial
- LeafletJS - Home
- LeafletJS - Getting Started
- LeafletJS - Markers
- LeafletJS - Vector Layers
- LeafletJS - Multi Polyline & Polygon
- LeafletJS - Layers Group
- LeafletJS - Event Handling
- LeafletJS - Overlays
- LeafletJS - Controls
- LeafletJS Useful Resources
- LeafletJS - Quick Guide
- LeafletJS - Useful Resources
- LeafletJS - Discussion
LeafletJS - Markers
To mark a single location on the map, leaflet provides markers. These markers use a standard symbol and these symbols can be customized. In this chapter, we will see how to add markers and how to customize, animate, and remove them.
Adding a Simple Marker
To add a marker to a map using Leaflet JavaScript library, follow the steps given below −
Step 1 − Create a Map object by passing a <div> element (String or object) and map options (optional).
Step 2 − Create a Layer object by passing the URL of the desired tile.
Step 3 − Add the layer object to the map using the addLayer() method of the Map class.
Step 4 − Instantiate the Marker class by passing a latlng object representing the position to be marked, as shown below.
// Creating a marker var marker = new L.Marker([17.385044, 78.486671]);
Step 5 − Add the marker object created in the previous steps to the map using the addTo() method of the Marker class.
// Adding marker to the map marker.addTo(map);
Example
The following code sets the marker on the city named Hyderabad (India).
<!DOCTYPE html> <html> <head> <title>Leaflet sample</title> <link rel = "stylesheet" href = "http://cdn.leafletjs.com/leaflet-0.7.3/leaflet.css"/> <script src = "http://cdn.leafletjs.com/leaflet-0.7.3/leaflet.js"></script> </head> <body> <div id = "map" style = "width:900px; height:580px"></div> <script> // Creating map options var mapOptions = { center: [17.385044, 78.486671], zoom: 10 } // Creating a map object var map = new L.map('map', mapOptions); // Creating a Layer object var layer = new L.TileLayer('http://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png'); // Adding layer to the map map.addLayer(layer); // Creating a marker var marker = L.marker([17.385044, 78.486671]); // Adding marker to the map marker.addTo(map); </script> </body> </html>
It generates the following output −
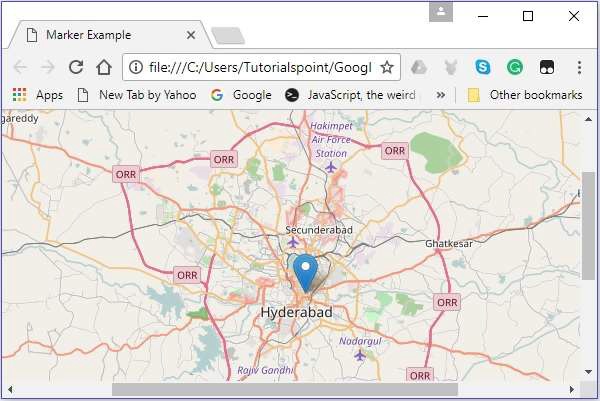
Binding Pop-ups to the Marker
To bind a simple popup displaying a message to a marker, follow the steps given below −
Step 1 − Create a Map object by passing a <div> element (String or object) and map options (optional).
Step 2 − Create a Layer object by passing the URL of the desired tile.
Step 3 − Add the layer object to the map using the addLayer() method of the Map class.
Step 4 − Instantiate the Marker class by passing a latlng object representing the position to be marked.
Step 5 − Attach popup to the marker using bindPopup() as shown below.
// Adding pop-up to the marker marker.bindPopup('Hi Welcome to Tutorialspoint').openPopup();
Step 6 − Finally, add the Marker object created in the previous steps to the map using the addTo() method of the Marker class.
Example
The following code sets the marker on the city Hyderabad (India) and adds a pop-up to it.
<!DOCTYPE html> <html> <head> <title>Binding pop-Ups to marker</title> <link rel = "stylesheet" href = "http://cdn.leafletjs.com/leaflet-0.7.3/leaflet.css"/> <script src = "http://cdn.leafletjs.com/leaflet-0.7.3/leaflet.js"></script> </head> <body> <div id = "map" style = "width:900px; height:580px"></div> <script> // Creating map options var mapOptions = { center: [17.385044, 78.486671], zoom: 15 } var map = new L.map('map', mapOptions); // Creating a map object // Creating a Layer object var layer = new L.TileLayer('http://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png'); map.addLayer(layer); // Adding layer to the map var marker = L.marker([17.438139, 78.395830]); // Creating a Marker // Adding popup to the marker marker.bindPopup('This is Tutorialspoint').openPopup(); marker.addTo(map); // Adding marker to the map </script> </body> </html>
It generates the following output
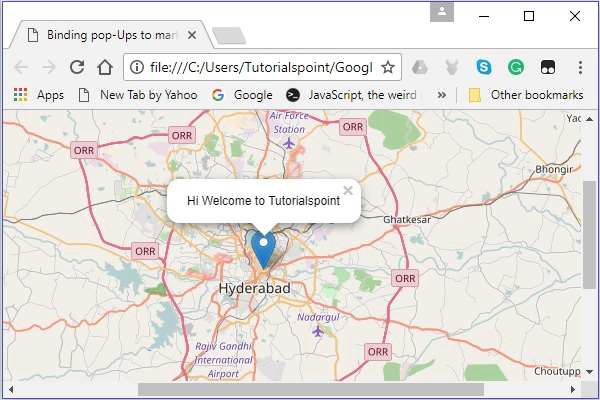
Marker Options
While creating a marker, you can also pass a marker options variable in addition to the latlang object. Using this variable, you can set values to various options of the marker such as icon, dragable, keyboard, title, alt, zInsexOffset, opacity, riseOnHover, riseOffset, pane, dragable, etc.
To create a map using map options, you need to follow the steps given below −
Step 1 − Create a Map object by passing a <div> element (String or object) and map options (optional).
Step 2 − Create a Layer object by passing the URL of the desired tile.
Step 3 − Add the layer object to the map using the addLayer() method of the Map class.
Step 4 − Create a variable for markerOptions and specify values to the required options.
Create a markerOptions object (it is created just like a literal) and set values for the options iconUrl and iconSize.
// Options for the marker var markerOptions = { title: "MyLocation", clickable: true, draggable: true }
Step 5 − Instantiate the Marker class by passing a latlng object representing the position to be marked and the options object, created in the previous step.
// Creating a marker var marker = L.marker([17.385044, 78.486671], markerOptions);
Step 6 − Finally, add the Marker object created in the previous steps to the map using the addTo() method of the Marker class.
Example
The following code sets the marker on the city Hyderabad (India). This marker is clickable, dragable with the title MyLocation.
<html> <head> <title>Marker Options Example</title> <link rel = "stylesheet" href = "http://cdn.leafletjs.com/leaflet-0.7.3/leaflet.css"/> <script src = "http://cdn.leafletjs.com/leaflet-0.7.3/leaflet.js"></script> </head> <body> <div id = "map" style = "width:900px; height:580px"></div> <script> // Creating map options var mapOptions = { center: [17.385044, 78.486671], zoom: 10 } // Creating a map object var map = new L.map('map', mapOptions); // Creating a Layer object var layer = new L.TileLayer('http://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png'); // Adding layer to the map map.addLayer(layer); // Creating a Marker var markerOptions = { title: "MyLocation", clickable: true, draggable: true } // Creating a marker var marker = L.marker([17.385044, 78.486671], markerOptions); // Adding marker to the map marker.addTo(map); </script> </body> </html>
It generates the following output
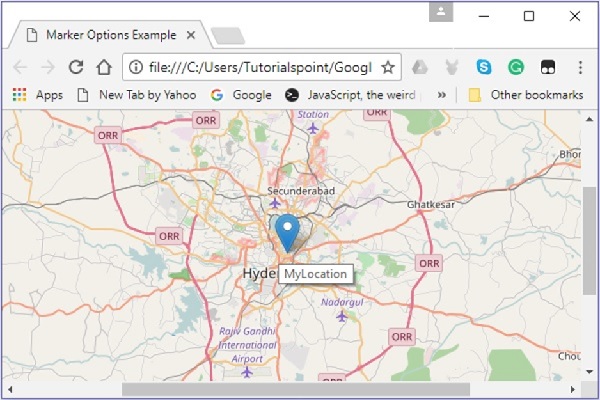
Marker Custom Icons
Instead of the default icon provided by the Leaflet library, you can also add your own icon. You can use the following steps to add a custom icon to the map instead of the default one.
Step 1 − Create a Map object by passing a <div> element (String or object) and map options (optional).
Step 2 − Create a Layer object by passing the URL of the desired tile.
Step 3 − Add the layer object to the map using the addLayer() method of the Map class.
Step 4 − Create a variable for markerOptions and specify values to the required options −
iconUrl − As a value to this option, you need to pass a String object specifying the path of the image which you want to use as an icon.
iconSize − Using this option, you can specify the size of the icon.
Note − In addition to these, you can also set values to other options such as iconSize, shadowSize, iconAnchor, shadowAnchor, and popupAnchor.
Create a custom icon using L.icon() by passing the above options variable as shown below.
// Icon options var iconOptions = { iconUrl: 'logo.png', iconSize: [50, 50] } // Creating a custom icon var customIcon = L.icon(iconOptions);
Step 5 − Create a variable for markerOptions and specify values to the required options. In addition to these, specify the icon by passing the icon variable created in the previous step as a value.
// Options for the marker var markerOptions = { title: "MyLocation", clickable: true, draggable: true, icon: customIcon }
Step 6 − Instantiate the Marker class by passing a latlng object representing the position to be marked and the options object created in the previous step.
// Creating a marker var marker = L.marker([17.438139, 78.395830], markerOptions);
Step 7 − Finally, add the Marker object created in the previous steps to the map using the addTo() method of the Marker class.
Example
The following code sets the marker on the location of Tutorialspoint. Here we are using the logo of Tutorialspoint instead of the default marker.
<!DOCTYPE html> <html> <head> <title>Marker Custom Icons Example</title> <link rel = "stylesheet" href = "http://cdn.leafletjs.com/leaflet-0.7.3/leaflet.css"/> <script src = "http://cdn.leafletjs.com/leaflet-0.7.3/leaflet.js"></script> </head> <body> <div id = "map" style = "width:900px; height:580px"></div> <script> // Creating map options var mapOptions = { center: [17.438139, 78.395830], zoom: 10 } // Creating a map object var map = new L.map('map', mapOptions); // Creating a Layer object var layer = new L.TileLayer('http://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png'); // Adding layer to the map map.addLayer(layer); // Icon options var iconOptions = { iconUrl: 'logo.png', iconSize: [50, 50] } // Creating a custom icon var customIcon = L.icon(iconOptions); // Creating Marker Options var markerOptions = { title: "MyLocation", clickable: true, draggable: true, icon: customIcon } // Creating a Marker var marker = L.marker([17.438139, 78.395830], markerOptions); // Adding popup to the marker marker.bindPopup('Hi welcome to Tutorialspoint').openPopup(); // Adding marker to the map marker.addTo(map); </script> </body> </html>
It generates the following output
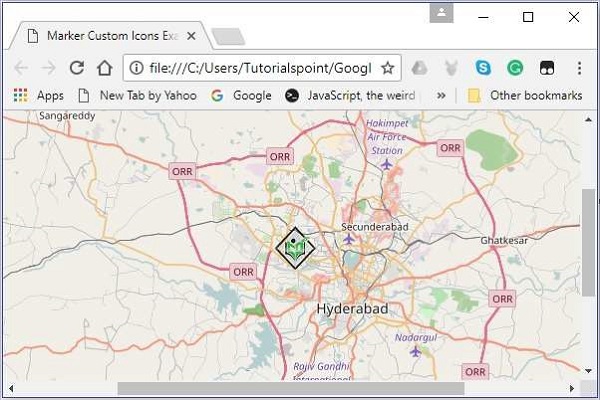