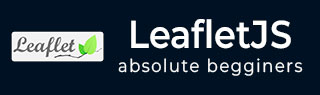
- LeafletJS Tutorial
- LeafletJS - Home
- LeafletJS - Getting Started
- LeafletJS - Markers
- LeafletJS - Vector Layers
- LeafletJS - Multi Polyline & Polygon
- LeafletJS - Layers Group
- LeafletJS - Event Handling
- LeafletJS - Overlays
- LeafletJS - Controls
- LeafletJS Useful Resources
- LeafletJS - Quick Guide
- LeafletJS - Useful Resources
- LeafletJS - Discussion
LeafletJS - Controls
Leaflet provides various controls such as zoom, attribution, scale, etc., where −
Zoom − By default, this control exists at the top left corner of the map. It has two buttons "+" and "–", using which you can zoom-in or zoom-out the map. You can remove the default zoom control by setting the zoomControl option of the map options to false.
Attribution − By default, this control exists at the bottom right corner of the map. It displays the attribution data in a small textbox. By default, it displays the text. You can remove the default attribution control by setting the attributionControl option of the map options to false.
Scale − By default, this control exists at the bottom left corner of the map. It displays the current center of the screen.
In this chapter, we will explain how you can create and add all these three controls to your map using Leaflet JavaScript library.
Zoom
To add a zoom control of your own to the map using Leaflet JavaScript library, follow the steps given below −
Step 1 − Create a Map object by passing a element (String or object) and map options (optional).
Step 2 − Create a Layer object by passing the URL of the desired tile.
Step 3 − Add the layer object to the map using the addLayer() method of the Map class.
Step 4 − Create the zoomOptions variable and define your own text values for the zoom-in and zoom-out options, instead of the default ones (+ and -).
Then, create the zoom control by passing the zoomOptions variable to L.control.zoom() as shown below.
// zoom control options var zoomOptions = { zoomInText: '1', zoomOutText: '0', }; // Creating zoom control var zoom = L.control.zoom(zoomOptions);
Step 5 − Add the zoom control object created in the previous step to the map using the addTo() method.
// Adding zoom control to the map zoom.addTo(map);
Example
Following is the code to add your own zoom control to your map, instead of the default one. Here, on pressing 1, the map zooms in, and on pressing 0, the map zooms out.
<!DOCTYPE html> <html> <head> <title>Zoom Example</title> <link rel = "stylesheet" href = "http://cdn.leafletjs.com/leaflet-0.7.3/leaflet.css"/> <script src = "http://cdn.leafletjs.com/leaflet-0.7.3/leaflet.js"></script> </head> <body> <div id = "map" style = "width:900px; height:580px"></div> <script> // Creating map options var mapOptions = { center: [17.385044, 78.486671], zoom: 10, zoomControl: false } var map = new L.map('map', mapOptions); // Creating a map object // Creating a Layer object var layer = new L.TileLayer('http://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png'); map.addLayer(layer); // Adding layer to the map // zoom control options var zoomOptions = { zoomInText: '1', zoomOutText: '0', }; var zoom = L.control.zoom(zoomOptions); // Creating zoom control zoom.addTo(map); // Adding zoom control to the map </script> </body> </html>
It generates the following output −
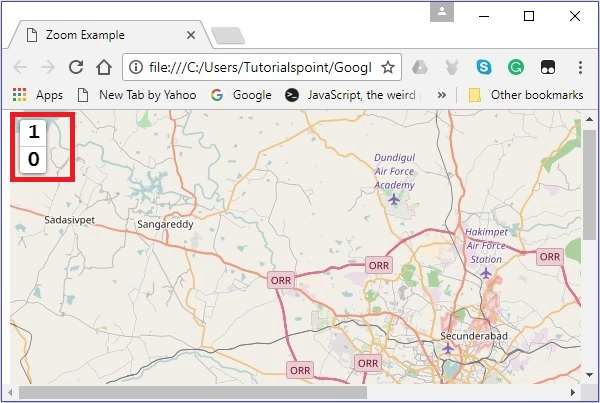
Attribution
To add an attribution of your own to the map using Leaflet JavaScript library, follow the steps given below −
Step 1 − Create a Map object by passing a <div> element (String or object) and map options (optional).
Step 2 − Create a Layer object by passing the URL of the desired tile.
Step 3 − Add the layer object to the map using the addLayer() method of the Map class.
Step 4 − Create the attrOptions variable and define your own prefix value instead of the default one (leaflet).
Then, create the attribution control by passing the attrOptions variable to L.control.attribution() as shown below.
// Attribution options var attrOptions = { prefix: 'attribution sample' }; // Creating an attribution var attr = L.control.attribution(attrOptions);
Step 5 − Add the attribution control object created in the previous step to the map using the addTo() method.
// Adding attribution to the map attr.addTo(map);
Example
The following code adds our own attribution control to your map, instead of the default one. Here, instead the text attribution sample will be displayed.
<!DOCTYPE html> <html> <head> <title>Attribution Example</title> <link rel = "stylesheet" href = "http://cdn.leafletjs.com/leaflet-0.7.3/leaflet.css"/> <script src = "http://cdn.leafletjs.com/leaflet-0.7.3/leaflet.js"></script> </head> <body> <div id = "map" style = "width: 900px; height: 580px"></div> <script> // Creating map options var mapOptions = { center: [17.385044, 78.486671], zoom: 10, attributionControl: false } var map = new L.map('map', mapOptions); // Creating a map object // Creating a Layer object var layer = new L.TileLayer('http://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png'); map.addLayer(layer); // Adding layer to the map // Attribution options var attrOptions = { prefix: 'attribution sample' }; // Creating an attribution var attr = L.control.attribution(attrOptions); attr.addTo(map); // Adding attribution to the map </script> </body> </html>>
It generates the following output −
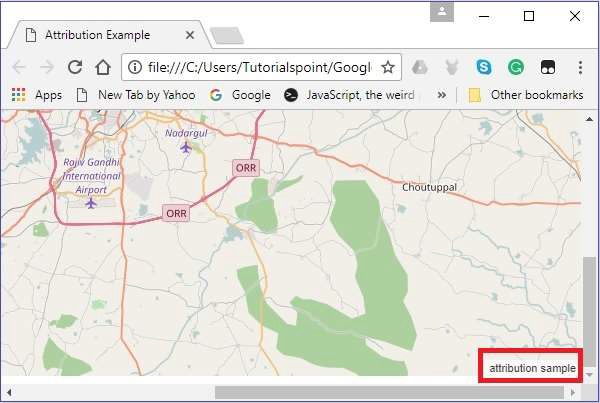
Scale
To add a scale control of your own to the map using Leaflet JavaScript library, follow the steps given below −
Step 1 − Create a Map object by passing a <div> element (String or object) and map options (optional).
Step 2 − Create a Layer object by passing the URL of the desired tile.
Step 3 − Add the layer object to the map using the addLayer() method of the Map class.
Step 4 − Create scale control by passing the using L.control.scale() as shown below.
// Creating scale control var scale = L.control.scale();
Step 5 − Add the scale control object created in the previous step to the map using the addTo() method as shown below.
// Adding scale control to the map scale.addTo(map);
Example
The following code adds scale control to your map.
<!DOCTYPE html> <html> <head> <title>Scale Example</title> <link rel = "stylesheet" href = "http://cdn.leafletjs.com/leaflet-0.7.3/leaflet.css" /> <script src = "http://cdn.leafletjs.com/leaflet-0.7.3/leaflet.js"></script> </head> <body> <div id = "map" style = "width:900px; height:580px"></div> <script> // Creating map options var mapOptions = { center: [17.385044, 78.486671], zoom: 10 } // Creating a map object var map = new L.map('map', mapOptions); // Creating a Layer object var layer = new L.TileLayer('http://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png'); map.addLayer(layer); // Adding layer to the map var scale = L.control.scale(); // Creating scale control scale.addTo(map); // Adding scale control to the map </script> </body> </html>
It generates the following output −
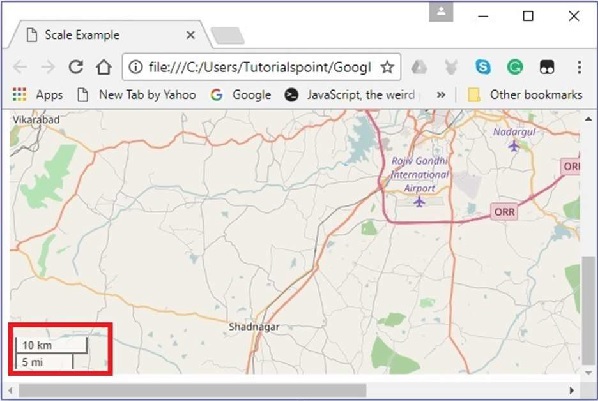