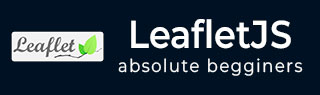
- LeafletJS Tutorial
- LeafletJS - Home
- LeafletJS - Getting Started
- LeafletJS - Markers
- LeafletJS - Vector Layers
- LeafletJS - Multi Polyline & Polygon
- LeafletJS - Layers Group
- LeafletJS - Event Handling
- LeafletJS - Overlays
- LeafletJS - Controls
- LeafletJS Useful Resources
- LeafletJS - Quick Guide
- LeafletJS - Useful Resources
- LeafletJS - Discussion
LeafletJS - Layers Group
Layer Groups
Using layer group, you can add multiple layers to a map and manage them as a single layer.
Follow the steps given below to create a LayerGroup and add it to the map.
Step 1 − Create a Map object by passing a <div> element (String or object) and map options (optional).
Step 2 − Create a Layer object by passing the URL of the desired tile.
Step 3 − Add the layer object to the map using the addLayer() method of the Map class.
Step 4 − Create elements (layers) such as markers, polygons, circles, etc., that are needed, by instantiating the respective classes as shown below.
// Creating markers var hydMarker = new L.Marker([17.385044, 78.486671]); var vskpMarker = new L.Marker([17.686816, 83.218482]); var vjwdMarker = new L.Marker([16.506174, 80.648015]); // Creating latlng object var latlngs = [ [17.385044, 78.486671], [16.506174, 80.648015], [17.686816, 83.218482] ]; // Creating a polygon var polygon = L.polygon(latlngs, {color: 'red'});
Step 5 − Create the Layer Group using l.layerGroup(). Pass the above created markers, polygons, etc., as shown below.
// Creating layer group var layerGroup = L.layerGroup([hydMarker, vskpMarker, vjwdMarker, polygon]);
Step 6 − Add the layer group created in the previous step using the addTo() method.
// Adding layer group to map layerGroup.addTo(map);
Example
The following code creates a layer group which holds 3 markers and a polygon, and adds it to the map.
<!DOCTYPE html> <html> <head> <title>Leaflet Layer Group</title> <link rel = "stylesheet" href = "http://cdn.leafletjs.com/leaflet-0.7.3/leaflet.css"/> <script src = "http://cdn.leafletjs.com/leaflet-0.7.3/leaflet.js"></script> </head> <body> <div id = "map" style = "width: 900px; height: 580px"></div> <script> // Creating map options var mapOptions = { center: [17.385044, 78.486671], zoom: 7 } var map = new L.map('map', mapOptions); // Creating a map object // Creating a Layer object var layer = new L.TileLayer('http://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png'); map.addLayer(layer); // Adding layer to the map // Creating markers var hydMarker = new L.Marker([17.385044, 78.486671]); var vskpMarker = new L.Marker([17.686816, 83.218482]); var vjwdMarker = new L.Marker([16.506174, 80.648015]); // Creating latlng object var latlngs = [ [17.385044, 78.486671], [16.506174, 80.648015], [17.686816, 83.218482] ]; // Creating a polygon var polygon = L.polygon(latlngs, {color: 'red'}); // Creating layer group var layerGroup = L.layerGroup([hydMarker, vskpMarker, vjwdMarker, polygon]); layerGroup.addTo(map); // Adding layer group to map </script> </body> </html>
It generates the following output −
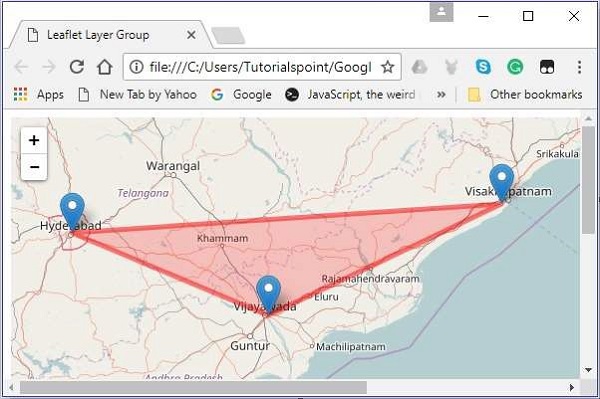
Adding a layer (element)
You can add a layer to the feature group using the addLayer() method. To this method, you need to pass the element that is to be added.
You can add a circle with the city Hyderabad at the center.
// Creating a circle var circle = L.circle([16.506174, 80.648015], 50000, {color: 'red', fillColor: '#f03', fillOpacity: 0} ); // Adding circle to the layer group layerGroup.addLayer(circle);
It will produce the following output. −
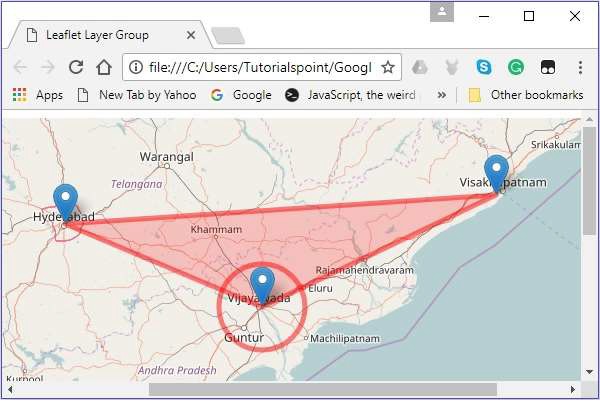
Removing a Layer (Element)
You can remove a layer from the feature group using the removeLayer() method. To this method, you need to pass the element that is to be removed.
You can remove the marker on the city named Vijayawada as shown below.
// Removing layer from map layerGroup.removeLayer(vjwdMarker);
It will produce the following output −
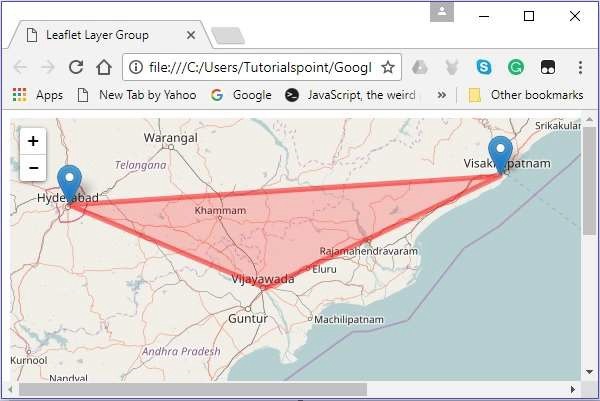
Feature Groups
It is similar to LayerGroup but it allows mouse events and bind popups to it. You can also set style to the entire group using setStyle() method.
Follow the steps given below to create a Feature Group and add it to the map.
Step 1 − Create a Map object by passing a <div> element (String or object) and map options (optional).
Step 2 − Create a Layer object by passing the URL of the desired tile.
Step 3 − Add the layer object to the map using the addLayer() method of the Map class.
Step 4 − Create elements (layers) such as markers, polygons, and circles that are needed, by instantiating the respective classes as shown below.
// Creating markers var hydMarker = new L.Marker([17.385044, 78.486671]); var vskpMarker = new L.Marker([17.686816, 83.218482]); var vjwdMarker = new L.Marker([16.506174, 80.648015]); // Creating latlng object var latlngs = [ [17.385044, 78.486671], [16.506174, 80.648015], [17.686816, 83.218482] ]; // Creating a polygon var polygon = L.polygon(latlngs, {color: 'red'});>
Step 5 − Create Feature Group using l.featureGroup(). Pass the above-created markers, polygons, etc., as shown below.
// Creating feature group var featureGroup = L.featureGroup([hydMarker, vskpMarker, vjwdMarker, polygon]);
Step 6 − If you set style to the feature group, it will be applied to each element (layer) in the group. You can do so using the setStyle() method and to this method, you need to pass values to the options such as color and opacity etc.
Set the style to the feature group created in the above step.
// Setting style to the feature group featureGroup.setStyle({color:'blue',opacity:.5});
Step 7 − Bind the popup using the bindPopup() method, as shown below.
// Binding popup to the feature group featureGroup.bindPopup("Feature Group");
Step 8 − Add the feature group created in the previous step using the addTo() method.
// Adding layer group to map featureGroup.addTo(map);
Example
The following code creates a feature group which holds 3 markers and a polygon, and adds it to the map.
<!DOCTYPE html> <html> <head> <title>Leaflet Feature Group</title> <link rel = "stylesheet" href = "http://cdn.leafletjs.com/leaflet-0.7.3/leaflet.css"/> <script src = "http://cdn.leafletjs.com/leaflet-0.7.3/leaflet.js"></script> </head> <body> <div id = "map" style = "width:900px; height:580px"></div> <script> // Creating map options var mapOptions = { center: [17.385044, 78.486671], zoom: 7 } var map = new L.map('map', mapOptions); // Creating a map object // Creating a Layer object var layer = new L.TileLayer('http://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png'); map.addLayer(layer); // Adding layer to the map // Creating markers var hydMarker = new L.Marker([17.385044, 78.486671]); var vskpMarker = new L.Marker([17.686816, 83.218482]); var vjwdMarker = new L.Marker([16.506174, 80.648015]); // Creating latlng object var latlngs = [ [17.385044, 78.486671], [16.506174, 80.648015], [17.686816, 83.218482] ]; var polygon = L.polygon(latlngs, {color: 'red'}); // Creating a polygon // Creating feature group var featureGroup = L.featureGroup([hydMarker, vskpMarker, vjwdMarker, polygon]); featureGroup.setStyle({color:'blue',opacity:.5}); featureGroup.bindPopup("Feature Group"); featureGroup.addTo(map); // Adding layer group to map </script> </body> </html>
It generates the following output −
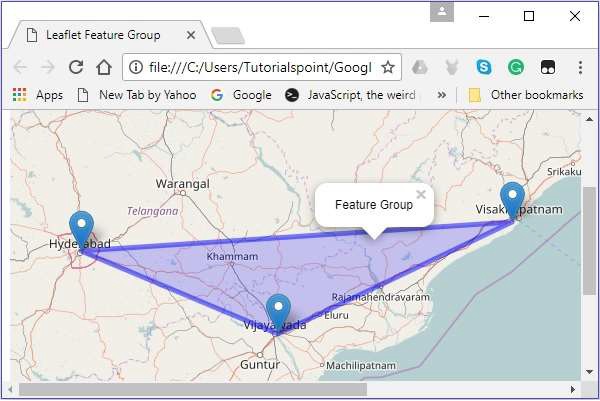
To Continue Learning Please Login