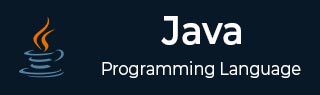
Java Tutorial
- Java - Home
- Java - Overview
- Java - History
- Java - Features
- Java vs C++
- Java Virtual Machine (JVM)
- Java - JDK vs JRE vs JVM
- Java - Hello World Program
- Java - Environment Setup
- Java - Basic Syntax
- Java - Variable Types
- Java - Data Types
- Java - Type Casting
- Java - Unicode System
- Java - Basic Operators
- Java - Comments
Java Control Statements
- Java - Loop Control
- Java - Decision Making
- Java - If-else
- Java - Switch
- Java - For Loops
- Java - For-Each Loops
- Java - While Loops
- Java - do-while Loops
- Java - Break
- Java - Continue
Object Oriented Programming
- Java - OOPs Concepts
- Java - Object & Classes
- Java - Class Attributes
- Java - Class Methods
- Java - Methods
- Java - Variables Scope
- Java - Constructors
- Java - Access Modifiers
- Java - Inheritance
- Java - Aggregation
- Java - Polymorphism
- Java - Overriding
- Java - Method Overloading
- Java - Dynamic Binding
- Java - Static Binding
- Java - Instance Initializer Block
- Java - Abstraction
- Java - Encapsulation
- Java - Interfaces
- Java - Packages
- Java - Inner Classes
- Java - Static Class
- Java - Anonymous Class
- Java - Singleton Class
- Java - Wrapper Classes
- Java - Enums
- Java - Enum Constructor
- Java - Enum Strings
Java Built-in Classes
Java File Handling
- Java - Files
- Java - Create a File
- Java - Write to File
- Java - Read Files
- Java - Delete Files
- Java - Directories
- Java - I/O Streams
Java Error & Exceptions
- Java - Exceptions
- Java - try-catch Block
- Java - try-with-resources
- Java - Multi-catch Block
- Java - Nested try Block
- Java - Finally Block
- Java - throw Exception
- Java - Exception Propagation
- Java - Built-in Exceptions
- Java - Custom Exception
Java Multithreading
- Java - Multithreading
- Java - Thread Life Cycle
- Java - Creating a Thread
- Java - Starting a Thread
- Java - Joining Threads
- Java - Naming Thread
- Java - Thread Scheduler
- Java - Thread Pools
- Java - Main Thread
- Java - Thread Priority
- Java - Daemon Threads
- Java - Thread Group
- Java - Shutdown Hook
Java Synchronization
- Java - Synchronization
- Java - Block Synchronization
- Java - Static Synchronization
- Java - Inter-thread Communication
- Java - Thread Deadlock
- Java - Interrupting a Thread
- Java - Thread Control
- Java - Reentrant Monitor
Java Networking
- Java - Networking
- Java - Socket Programming
- Java - URL Processing
- Java - URL Class
- Java - URLConnection Class
- Java - HttpURLConnection Class
- Java - Socket Class
- Java - Generics
Java Collections
Java Interfaces
- Java - List Interface
- Java - Queue Interface
- Java - Map Interface
- Java - SortedMap Interface
- Java - Set Interface
- Java - SortedSet Interface
Java Data Structures
Java Collections Algorithms
Advanced Java
- Java - Command-Line Arguments
- Java - Lambda Expressions
- Java - Sending Email
- Java - Applet Basics
- Java - Javadoc Comments
- Java - Autoboxing and Unboxing
- Java - File Mismatch Method
- Java - REPL (JShell)
- Java - Multi-Release Jar Files
- Java - Private Interface Methods
- Java - Inner Class Diamond Operator
- Java - Multiresolution Image API
- Java - Collection Factory Methods
- Java - Module System
- Java - Nashorn JavaScript
- Java - Optional Class
- Java - Method References
- Java - Functional Interfaces
- Java - Default Methods
- Java - Base64 Encode Decode
- Java - Switch Expressions
- Java - Teeing Collectors
- Java - Microbenchmark
- Java - Text Blocks
- Java - Dynamic CDS archive
- Java - Z Garbage Collector (ZGC)
- Java - Null Pointer Exception
- Java - Packaging Tools
- Java - Sealed Classes
- Java - Record Classes
- Java - Hidden Classes
- Java - Pattern Matching
- Java - Compact Number Formatting
- Java - Garbage Collection
- Java - JIT Compiler
Java Miscellaneous
- Java - Recursion
- Java - Regular Expressions
- Java - Serialization
- Java - Strings
- Java - Process API Improvements
- Java - Stream API Improvements
- Java - Enhanced @Deprecated Annotation
- Java - CompletableFuture API Improvements
- Java - Streams
- Java - Datetime Api
- Java 8 - New Features
- Java 9 - New Features
- Java 10 - New Features
- Java 11 - New Features
- Java 12 - New Features
- Java 13 - New Features
- Java 14 - New Features
- Java 15 - New Features
- Java 16 - New Features
Java APIs & Frameworks
Java Class References
- Java - Scanner Class
- Java - Arrays Class
- Java - Strings
- Java - Date & Time
- Java - ArrayList
- Java - Vector Class
- Java - Stack Class
- Java - PriorityQueue
- Java - LinkedList
- Java - ArrayDeque
- Java - HashMap
- Java - LinkedHashMap
- Java - WeakHashMap
- Java - EnumMap
- Java - TreeMap
- Java - The IdentityHashMap Class
- Java - HashSet
- Java - EnumSet
- Java - LinkedHashSet
- Java - TreeSet
- Java - BitSet Class
- Java - Dictionary
- Java - Hashtable
- Java - Properties
- Java - Collection Interface
- Java - Array Methods
Java Useful Resources
Java - URL getContent(Class<?>[] classes) Method
Description
The Java URL getContent(Class<?>[] classes) method returns the contents of this URL. This method is a shorthand for. −
openConnection().getContent(classes)
Declaration
Following is the declaration for java.net.URL.getContent() method
public final Object getContent(Class<?>[] classes)
Parameters
classes − an array of Java types
Return Value
the contents of this URL that is the first match of the types specified in the classes array. null if none of the requested types are supported.
Exception
IOException − if an I/O exception occurs.
Example 1
The following example shows the usage of Java URL getContent(Class<?>[] classes) method for a valid url. In this example, we're creating an instances of URL class. Now using getContent() method, we're getting the content and printing its type −
package com.tutorialspoint; import java.io.IOException; import java.io.InputStream; import java.net.URL; public class UrlDemo { public static void main(String [] args) { try { URL url = new URL("https","www.tutorialspoint.com","/index.htm"); Class<?>[] classes = {InputStream.class}; Object content = url.getContent(classes); System.out.println(content); } catch (IOException e) { e.printStackTrace(); } } }
Let us compile and run the above program, this will produce the following result −
Output
sun.net.www.protocol.http.HttpURLConnection$HttpInputStream@6df97b55
Example 2
The following example shows the usage of Java URL getContent(Class<?>[] classes) method for a invalid url. In this example, we're creating an instances of URL class. Now using getContent() method, we're are trying to get the content and an exception is raised and printed −
package com.tutorialspoint; import java.io.IOException; import java.io.InputStream; import java.net.URL; public class UrlDemo { public static void main(String [] args) { try { URL url = new URL("https","www.tutorialspoint.com","index.htm"); Class<?>[] classes = {InputStream.class}; Object content = url.getContent(classes); System.out.println(content); } catch (IOException e) { e.printStackTrace(); } } }
Let us compile and run the above program, this will produce the following result −
Output
java.io.IOException: Server returned HTTP response code: 400 for URL: https://www.tutorialspoint.comindex.htm at sun.net.www.protocol.http.HttpURLConnection.getInputStream0(Unknown Source) at sun.net.www.protocol.http.HttpURLConnection.getInputStream(Unknown Source) at java.net.URLConnection.getContent(Unknown Source) at sun.net.www.protocol.https.HttpsURLConnectionImpl.getContent(Unknown Source) at java.net.URL.getContent(Unknown Source) at com.tutorialspoint.UrlDemo.main(UrlDemo.java:10)
Example 3
The following example shows the usage of Java URL getContent(Class<?>[] classes) method for a valid url. In this example, we're creating an instances of URL class using protocol, host and file name. Now using getContent() method, we're we're getting the content and printing its type then using openConnection() method, we're getting the content length and printed it in output as shown below −
package com.tutorialspoint; import java.io.IOException; import java.io.InputStream; import java.net.URL; public class UrlDemo { public static void main(String [] args) { try { URL url = new URL("https","www.tutorialspoint.com","/index.htm"); Class<?>[] classes = {InputStream.class}; Object content = url.getContent(classes); System.out.println(content.getClass()); System.out.println(url.openConnection().getContentLength()); } catch (IOException e) { e.printStackTrace(); } } }
Let us compile and run the above program, this will produce the following result −
Output
class sun.net.www.protocol.http.HttpURLConnection$HttpInputStream 293637