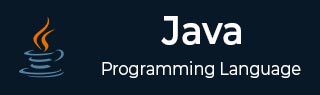
Java Tutorial
- Java - Home
- Java - Overview
- Java - History
- Java - Features
- Java vs C++
- Java Virtual Machine (JVM)
- Java - JDK vs JRE vs JVM
- Java - Hello World Program
- Java - Environment Setup
- Java - Basic Syntax
- Java - Variable Types
- Java - Data Types
- Java - Type Casting
- Java - Unicode System
- Java - Basic Operators
- Java - Comments
- Java - User Input
Java Control Statements
- Java - Loop Control
- Java - Decision Making
- Java - If-else
- Java - Switch
- Java - For Loops
- Java - For-Each Loops
- Java - While Loops
- Java - do-while Loops
- Java - Break
- Java - Continue
Object Oriented Programming
- Java - OOPs Concepts
- Java - Object & Classes
- Java - Class Attributes
- Java - Class Methods
- Java - Methods
- Java - Variables Scope
- Java - Constructors
- Java - Access Modifiers
- Java - Inheritance
- Java - Aggregation
- Java - Polymorphism
- Java - Overriding
- Java - Method Overloading
- Java - Dynamic Binding
- Java - Static Binding
- Java - Instance Initializer Block
- Java - Abstraction
- Java - Encapsulation
- Java - Interfaces
- Java - Packages
- Java - Inner Classes
- Java - Static Class
- Java - Anonymous Class
- Java - Singleton Class
- Java - Wrapper Classes
- Java - Enums
- Java - Enum Constructor
- Java - Enum Strings
Java Built-in Classes
- Java - Number
- Java - Boolean
- Java - Characters
- Java - Strings
- Java - Arrays
- Java - Date & Time
- Java - Math Class
Java File Handling
- Java - Files
- Java - Create a File
- Java - Write to File
- Java - Read Files
- Java - Delete Files
- Java - Directories
- Java - I/O Streams
Java Error & Exceptions
- Java - Exceptions
- Java - try-catch Block
- Java - try-with-resources
- Java - Multi-catch Block
- Java - Nested try Block
- Java - Finally Block
- Java - throw Exception
- Java - Exception Propagation
- Java - Built-in Exceptions
- Java - Custom Exception
Java Multithreading
- Java - Multithreading
- Java - Thread Life Cycle
- Java - Creating a Thread
- Java - Starting a Thread
- Java - Joining Threads
- Java - Naming Thread
- Java - Thread Scheduler
- Java - Thread Pools
- Java - Main Thread
- Java - Thread Priority
- Java - Daemon Threads
- Java - Thread Group
- Java - Shutdown Hook
Java Synchronization
- Java - Synchronization
- Java - Block Synchronization
- Java - Static Synchronization
- Java - Inter-thread Communication
- Java - Thread Deadlock
- Java - Interrupting a Thread
- Java - Thread Control
- Java - Reentrant Monitor
Java Networking
- Java - Networking
- Java - Socket Programming
- Java - URL Processing
- Java - URL Class
- Java - URLConnection Class
- Java - HttpURLConnection Class
- Java - Socket Class
- Java - Generics
Java Collections
Java List Interface
Java Queue Interface
Java Map Interface
- Java - Map Interface
- Java - HashMap
- Java - LinkedHashMap
- Java - WeakHashMap
- Java - EnumMap
- Java - SortedMap Interface
- Java - TreeMap
- Java - The IdentityHashMap Class
Java Set Interface
- Java - Set Interface
- Java - HashSet
- Java - EnumSet
- Java - LinkedHashSet
- Java - SortedSet Interface
- Java - TreeSet
Java Data Structures
- Java - Data Structures
- Java - Enumeration
- Java - BitSet Class
- Java - Dictionary
- Java - Hashtable
- Java - Properties
Java Collections Algorithms
Advanced Java
- Java - Command-Line Arguments
- Java - Lambda Expressions
- Java - Sending Email
- Java - Applet Basics
- Java - Javadoc Comments
- Java - Autoboxing and Unboxing
- Java - File Mismatch Method
- Java - REPL (JShell)
- Java - Multi-Release Jar Files
- Java - Private Interface Methods
- Java - Inner Class Diamond Operator
- Java - Multiresolution Image API
- Java - Collection Factory Methods
- Java - Module System
- Java - Nashorn JavaScript
- Java - Optional Class
- Java - Method References
- Java - Functional Interfaces
- Java - Default Methods
- Java - Base64 Encode Decode
- Java - Switch Expressions
- Java - Teeing Collectors
- Java - Microbenchmark
- Java - Text Blocks
- Java - Null Pointer Exception
- Java - Packaging Tools
- Java - Sealed Classes
- Java - Record Classes
- Java - Hidden Classes
- Java - Compact Number Formatting
- Java - Garbage Collection
- Java - JIT Compiler
Java Miscellaneous
- Java - Recursion
- Java - Regular Expressions
- Java - Serialization
- Java - Strings
- Java - Process API Improvements
- Java - Stream API Improvements
- Java - Enhanced @Deprecated Annotation
- Java - CompletableFuture API Improvements
- Java - Array Methods
- Java - Streams
- Java - Datetime Api
- Java 8 - New Features
- Java 9 - New Features
- Java 10 - New Features
- Java 11 - New Features
- Java 12 - New Features
Java APIs & Frameworks
Java Useful Resources
Java - Directory Operations
Directory in Java
A directory is a File which can contain a list of other files and directories. You use File object to create directories, to list down files available in a directory. For complete detail, check a list of all the methods which you can call on File object and what are related to directories.
Creating Directories
There are two useful File utility methods, which can be used to create directories −
The mkdir() method creates a directory, returning true on success and false on failure. Failure indicates that the path specified in the File object already exists, or that the directory cannot be created because the entire path does not exist yet.
The mkdirs() method creates both a directory and all the parents of the directory.
Example to Create Directory in Java
Following example creates "/tmp/user/java/bin" directory −
package com.tutorialspoint; import java.io.File; public class DirectoryTest { public static void main(String args[]) { String dirname = "/tmp/user/java/bin"; File directory = new File(dirname); // Create directory now. directory.mkdirs(); // create new file object File file = new File("/tmp/user/java/bin"); System.out.println(file.exists()); } }
Output
Compile and execute the above code to create "/tmp/user/java/bin" folders.
true
Note − Java automatically takes care of path separators on UNIX and Windows as per conventions. If you use a forward slash (/) on a Windows version of Java, the path will still resolve correctly.
Listing (Reading) Directories
You can use list() method provided by File object to list down all the files and directories available in a directory as follows −
Example to Read (List) a Directory in Java
package com.tutorialspoint; import java.io.File; public class DirectoryTest { public static void main(String[] args) { File file = null; String[] paths; try { // create new file object file = new File("/tmp"); // array of files and directory paths = file.list(); // for each name in the path array for(String path:paths) { // prints filename and directory name System.out.println(path); } } catch (Exception e) { // if any error occurs e.printStackTrace(); } } }
Output
This will produce the following result based on the directories and files available in your /tmp directory −
user
Deleting Directories
You can use delete() method provided by File object to delete a directory as follows −
Example to Delete a Directory in Java
package com.tutorialspoint; import java.io.File; public class DirectoryTest { public static void main(String[] args) { File file = new File("/tmp/user/java/bin"); if(file.exists()) { boolean success = file.delete(); if (success) { System.out.println("The directory has been successfully deleted."); }else { System.out.println("The directory deletion failed."); } }else { System.out.println("The directory is not present."); } } }
Output
This will produce the following result based on the directories and files available in your /tmp directory −
The directory has been successfully deleted.