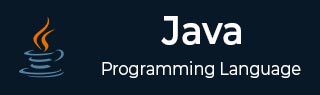
Java Tutorial
- Java - Home
- Java - Overview
- Java - History
- Java - Features
- Java vs C++
- Java Virtual Machine (JVM)
- Java - JDK vs JRE vs JVM
- Java - Hello World Program
- Java - Environment Setup
- Java - Basic Syntax
- Java - Variable Types
- Java - Data Types
- Java - Type Casting
- Java - Unicode System
- Java - Basic Operators
- Java - Comments
- Java - User Input
Java Control Statements
- Java - Loop Control
- Java - Decision Making
- Java - If-else
- Java - Switch
- Java - For Loops
- Java - For-Each Loops
- Java - While Loops
- Java - do-while Loops
- Java - Break
- Java - Continue
Object Oriented Programming
- Java - OOPs Concepts
- Java - Object & Classes
- Java - Class Attributes
- Java - Class Methods
- Java - Methods
- Java - Variables Scope
- Java - Constructors
- Java - Access Modifiers
- Java - Inheritance
- Java - Aggregation
- Java - Polymorphism
- Java - Overriding
- Java - Method Overloading
- Java - Dynamic Binding
- Java - Static Binding
- Java - Instance Initializer Block
- Java - Abstraction
- Java - Encapsulation
- Java - Interfaces
- Java - Packages
- Java - Inner Classes
- Java - Static Class
- Java - Anonymous Class
- Java - Singleton Class
- Java - Wrapper Classes
- Java - Enums
- Java - Enum Constructor
- Java - Enum Strings
Java Built-in Classes
- Java - Number
- Java - Boolean
- Java - Characters
- Java - Strings
- Java - Arrays
- Java - Date & Time
- Java - Math Class
Java File Handling
- Java - Files
- Java - Create a File
- Java - Write to File
- Java - Read Files
- Java - Delete Files
- Java - Directories
- Java - I/O Streams
Java Error & Exceptions
- Java - Exceptions
- Java - try-catch Block
- Java - try-with-resources
- Java - Multi-catch Block
- Java - Nested try Block
- Java - Finally Block
- Java - throw Exception
- Java - Exception Propagation
- Java - Built-in Exceptions
- Java - Custom Exception
Java Multithreading
- Java - Multithreading
- Java - Thread Life Cycle
- Java - Creating a Thread
- Java - Starting a Thread
- Java - Joining Threads
- Java - Naming Thread
- Java - Thread Scheduler
- Java - Thread Pools
- Java - Main Thread
- Java - Thread Priority
- Java - Daemon Threads
- Java - Thread Group
- Java - Shutdown Hook
Java Synchronization
- Java - Synchronization
- Java - Block Synchronization
- Java - Static Synchronization
- Java - Inter-thread Communication
- Java - Thread Deadlock
- Java - Interrupting a Thread
- Java - Thread Control
- Java - Reentrant Monitor
Java Networking
- Java - Networking
- Java - Socket Programming
- Java - URL Processing
- Java - URL Class
- Java - URLConnection Class
- Java - HttpURLConnection Class
- Java - Socket Class
- Java - Generics
Java Collections
Java List Interface
Java Queue Interface
Java Map Interface
- Java - Map Interface
- Java - HashMap
- Java - LinkedHashMap
- Java - WeakHashMap
- Java - EnumMap
- Java - SortedMap Interface
- Java - TreeMap
- Java - The IdentityHashMap Class
Java Set Interface
- Java - Set Interface
- Java - HashSet
- Java - EnumSet
- Java - LinkedHashSet
- Java - SortedSet Interface
- Java - TreeSet
Java Data Structures
- Java - Data Structures
- Java - Enumeration
- Java - BitSet Class
- Java - Dictionary
- Java - Hashtable
- Java - Properties
Java Collections Algorithms
Advanced Java
- Java - Command-Line Arguments
- Java - Lambda Expressions
- Java - Sending Email
- Java - Applet Basics
- Java - Javadoc Comments
- Java - Autoboxing and Unboxing
- Java - File Mismatch Method
- Java - REPL (JShell)
- Java - Multi-Release Jar Files
- Java - Private Interface Methods
- Java - Inner Class Diamond Operator
- Java - Multiresolution Image API
- Java - Collection Factory Methods
- Java - Module System
- Java - Nashorn JavaScript
- Java - Optional Class
- Java - Method References
- Java - Functional Interfaces
- Java - Default Methods
- Java - Base64 Encode Decode
- Java - Switch Expressions
- Java - Teeing Collectors
- Java - Microbenchmark
- Java - Text Blocks
- Java - Null Pointer Exception
- Java - Packaging Tools
- Java - Sealed Classes
- Java - Record Classes
- Java - Hidden Classes
- Java - Compact Number Formatting
- Java - Garbage Collection
- Java - JIT Compiler
Java Miscellaneous
- Java - Recursion
- Java - Regular Expressions
- Java - Serialization
- Java - Strings
- Java - Process API Improvements
- Java - Stream API Improvements
- Java - Enhanced @Deprecated Annotation
- Java - CompletableFuture API Improvements
- Java - Array Methods
- Java - Streams
- Java - Datetime Api
- Java 8 - New Features
- Java 9 - New Features
- Java 10 - New Features
- Java 11 - New Features
- Java 12 - New Features
Java APIs & Frameworks
Java Useful Resources
Java - SortedSet Interface
The SortedSet interface extends Set and declares the behavior of a set sorted in an ascending order. In addition to those methods defined by Set, the SortedSet interface declares the methods summarized in the following table −
Several methods throw a NoSuchElementException when no items are contained in the invoking set. A ClassCastException is thrown when an object is incompatible with the elements in a set.
A NullPointerException is thrown if an attempt is made to use a null object and null is not allowed in the set.
SortedSet Interface Methods
Sr.No. | Method & Description |
---|---|
1 | Comparator comparator( ) Returns the invoking sorted set's comparator. If the natural ordering is used for this set, null is returned. |
2 | Object first( ) Returns the first element in the invoking sorted set. |
3 | SortedSet headSet(Object end) Returns a SortedSet containing those elements less than end that are contained in the invoking sorted set. Elements in the returned sorted set are also referenced by the invoking sorted set. |
4 | Object last( ) Returns the last element in the invoking sorted set. |
5 | SortedSet subSet(Object start, Object end) Returns a SortedSet that includes those elements between start and end.1. Elements in the returned collection are also referenced by the invoking object. |
6 | SortedSet tailSet(Object start) Returns a SortedSet that contains those elements greater than or equal to start that are contained in the sorted set. Elements in the returned set are also referenced by the invoking object. |
Operations on SortedSet Interface
Creating a SortedSet
TreeSet class implements the SortedSet interface. We can use the TreeSet constructor to create a SortedSet instance. Following is the syntax to create a SortedSet instance:
Syntax
// Create the sorted set SortedSet<String> set = new TreeSet<>();
Here we're creating a sorted set of String values. This map will store the unique string values. If a duplicate value is added, then that will be discarded.
Adding Value to a SortedSet
SortedSet provides an add() method, which can be used to add value to a SortedSet instance. Whenever a value is added to the set, it is checked against the existing values. If the set is modified then method will return true otherwise false will be returned.
Syntax
public boolean add(E e)
Where E represents the element to be added. If element is already present, then no action will be performed and method will return false.
Example
// Add elements to the set set.add("b"); set.add("c"); set.add("a");
Getting value from a SortedSet
In order to get values from a SortedSet, we've to get the iterator object from the SortedSet using the iterator() method. Once the iterator object is available then that object can be used to retrieve values present in the SortedSet.
Example
// Iterating over the elements in the set Iterator it = set.iterator(); while (it.hasNext()) { // Get element Object element = it.next(); System.out.println(element.toString()); }
Deleting a value from a SortedSet
Using the remove(value) method, we can remove the value/object stored in the SortedSet easily.
Syntax
public boolean remove(Object value)
if value is not present in the set, then it will return false otherwise it will remove the value and return true.
set.remove("a");
Iterating SortedSet
SortedSet entries can be easily navigated. SortedSet provides a method iterator() which provides an iterator to navigate all the entries of the set.
Syntax
public Iterator<E> iterator()
Where E is the type of objects to be iterated.
Example
// Iterating over the elements in the set Iterator it = set.iterator(); while (it.hasNext()) { // Get element Object element = it.next(); System.out.println(element.toString()); }
Examples of SortedSet Interface
Adding Element to a SortedSet Example
SortedSet have its implementation in various classes like TreeSet. Following is an example of a TreeSet class with add operation−
import java.util.Iterator; import java.util.SortedSet; import java.util.TreeSet; public class SortedSetDemo { public static void main(String[] args) { // Create the sorted set SortedSet<String> set = new TreeSet<>(); // Add elements to the set set.add("b"); set.add("c"); set.add("a"); // Iterating over the elements in the set Iterator it = set.iterator(); while (it.hasNext()) { // Get element Object element = it.next(); System.out.println(element.toString()); } } }
Output
a b c
Removing Element from a SortedSet Example
SortedSet have its implementation in various classes like TreeSet. Following is an example of a TreeSet class with add and remove operation −
import java.util.Iterator; import java.util.SortedSet; import java.util.TreeSet; public class SortedSetDemo { public static void main(String[] args) { // Create the sorted set SortedSet<String> set = new TreeSet<>(); // Add elements to the set set.add("b"); set.add("c"); set.add("a"); set.add("d"); set.add("e"); set.add("f"); // remove elements set.remove("c"); set.remove("f"); // Iterating over the elements in the set Iterator it = set.iterator(); while (it.hasNext()) { // Get element Object element = it.next(); System.out.println(element.toString()); } } }
Output
a b d e
Clearing a SortedSet Example
SortedSet have its implementation in various classes like TreeSet. Following is an example of a TreeSet class with add and clear operation −
import java.util.Iterator; import java.util.SortedSet; import java.util.TreeSet; public class SortedSetDemo { public static void main(String[] args) { // Create the sorted set SortedSet<String> set = new TreeSet<>(); // Add elements to the set set.add("b"); set.add("c"); set.add("a"); set.add("d"); set.add("e"); set.add("f"); System.out.println(set); // remove elements set.clear(); System.out.println(set); } }
Output
[a, b, c, d, e, f] []
Advantages of SortedSet Interface
- SortedSet ensures that the map is always sorted in ascending order of the values. Whenever a key-value pair is added to the SortedSet, it is re-sorted
- Being sorted and unique, SortedSet is very efficient in searches
- We can customize the sorting mechanism by using a comparator on the value type.
Disadvantages of SortedSet Interface
- As a SortedSet instance has to be sorted every time an entry is added or changed, it becomes a performance bottleneck where changes are very frequent. In such cases, SortedSet is not preferred.
- As SortedSet maintains unique records only, we cannot use this collection where duplicate entries can occur in the data set.