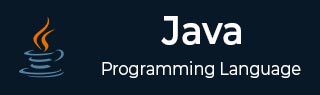
Java Tutorial
- Java - Home
- Java - Overview
- Java - History
- Java - Features
- Java vs C++
- Java Virtual Machine (JVM)
- Java - JDK vs JRE vs JVM
- Java - Hello World Program
- Java - Environment Setup
- Java - Basic Syntax
- Java - Variable Types
- Java - Data Types
- Java - Type Casting
- Java - Unicode System
- Java - Basic Operators
- Java - Comments
- Java - User Input
Java Control Statements
- Java - Loop Control
- Java - Decision Making
- Java - If-else
- Java - Switch
- Java - For Loops
- Java - For-Each Loops
- Java - While Loops
- Java - do-while Loops
- Java - Break
- Java - Continue
Object Oriented Programming
- Java - OOPs Concepts
- Java - Object & Classes
- Java - Class Attributes
- Java - Class Methods
- Java - Methods
- Java - Variables Scope
- Java - Constructors
- Java - Access Modifiers
- Java - Inheritance
- Java - Aggregation
- Java - Polymorphism
- Java - Overriding
- Java - Method Overloading
- Java - Dynamic Binding
- Java - Static Binding
- Java - Instance Initializer Block
- Java - Abstraction
- Java - Encapsulation
- Java - Interfaces
- Java - Packages
- Java - Inner Classes
- Java - Static Class
- Java - Anonymous Class
- Java - Singleton Class
- Java - Wrapper Classes
- Java - Enums
- Java - Enum Constructor
- Java - Enum Strings
Java Built-in Classes
- Java - Number
- Java - Boolean
- Java - Characters
- Java - Strings
- Java - Arrays
- Java - Date & Time
- Java - Math Class
Java File Handling
- Java - Files
- Java - Create a File
- Java - Write to File
- Java - Read Files
- Java - Delete Files
- Java - Directories
- Java - I/O Streams
Java Error & Exceptions
- Java - Exceptions
- Java - try-catch Block
- Java - try-with-resources
- Java - Multi-catch Block
- Java - Nested try Block
- Java - Finally Block
- Java - throw Exception
- Java - Exception Propagation
- Java - Built-in Exceptions
- Java - Custom Exception
Java Multithreading
- Java - Multithreading
- Java - Thread Life Cycle
- Java - Creating a Thread
- Java - Starting a Thread
- Java - Joining Threads
- Java - Naming Thread
- Java - Thread Scheduler
- Java - Thread Pools
- Java - Main Thread
- Java - Thread Priority
- Java - Daemon Threads
- Java - Thread Group
- Java - Shutdown Hook
Java Synchronization
- Java - Synchronization
- Java - Block Synchronization
- Java - Static Synchronization
- Java - Inter-thread Communication
- Java - Thread Deadlock
- Java - Interrupting a Thread
- Java - Thread Control
- Java - Reentrant Monitor
Java Networking
- Java - Networking
- Java - Socket Programming
- Java - URL Processing
- Java - URL Class
- Java - URLConnection Class
- Java - HttpURLConnection Class
- Java - Socket Class
- Java - Generics
Java Collections
Java List Interface
Java Queue Interface
Java Map Interface
- Java - Map Interface
- Java - HashMap
- Java - LinkedHashMap
- Java - WeakHashMap
- Java - EnumMap
- Java - SortedMap Interface
- Java - TreeMap
- Java - The IdentityHashMap Class
Java Set Interface
- Java - Set Interface
- Java - HashSet
- Java - EnumSet
- Java - LinkedHashSet
- Java - SortedSet Interface
- Java - TreeSet
Java Data Structures
- Java - Data Structures
- Java - Enumeration
- Java - BitSet Class
- Java - Dictionary
- Java - Hashtable
- Java - Properties
Java Collections Algorithms
Advanced Java
- Java - Command-Line Arguments
- Java - Lambda Expressions
- Java - Sending Email
- Java - Applet Basics
- Java - Javadoc Comments
- Java - Autoboxing and Unboxing
- Java - File Mismatch Method
- Java - REPL (JShell)
- Java - Multi-Release Jar Files
- Java - Private Interface Methods
- Java - Inner Class Diamond Operator
- Java - Multiresolution Image API
- Java - Collection Factory Methods
- Java - Module System
- Java - Nashorn JavaScript
- Java - Optional Class
- Java - Method References
- Java - Functional Interfaces
- Java - Default Methods
- Java - Base64 Encode Decode
- Java - Switch Expressions
- Java - Teeing Collectors
- Java - Microbenchmark
- Java - Text Blocks
- Java - Null Pointer Exception
- Java - Packaging Tools
- Java - Sealed Classes
- Java - Record Classes
- Java - Hidden Classes
- Java - Compact Number Formatting
- Java - Garbage Collection
- Java - JIT Compiler
Java Miscellaneous
- Java - Recursion
- Java - Regular Expressions
- Java - Serialization
- Java - Strings
- Java - Process API Improvements
- Java - Stream API Improvements
- Java - Enhanced @Deprecated Annotation
- Java - CompletableFuture API Improvements
- Java - Array Methods
- Java - Streams
- Java - Datetime Api
- Java 8 - New Features
- Java 9 - New Features
- Java 10 - New Features
- Java 11 - New Features
- Java 12 - New Features
Java APIs & Frameworks
Java Useful Resources
Java - Collection Interface
Collection Interface
The Collection interface is the foundation upon which the collections framework is built. It declares the core methods that all collections will have. There are several methods in the Collection interface to perform basic operations on collections. For example, adding, removing, and querying elements/objects.
Collection Interface Methods
The following is the list of the methods provided by the Collection Interface -
Sr.No. | Method & Description |
---|---|
1 | boolean add(Object obj) Adds obj to the invoking collection. Returns true if obj was added to the collection. Returns false if obj is already a member of the collection, or if the collection does not allow duplicates. |
2 | boolean addAll(Collection c) Adds all the elements of c to the invoking collection. Returns true if the operation succeeds (i.e., the elements were added). Otherwise, returns false. |
3 | void clear( ) Removes all elements from the invoking collection. |
4 | boolean contains(Object obj) Returns true if obj is an element of the invoking collection. Otherwise, returns false. |
5 | boolean containsAll(Collection c) Returns true if the invoking collection contains all elements of c. Otherwise, returns false. |
6 | boolean equals(Object obj) Returns true if the invoking collection and obj are equal. Otherwise, returns false. |
7 | int hashCode( ) Returns the hash code for the invoking collection. |
8 | boolean isEmpty( ) Returns true if the invoking collection is empty. Otherwise, returns false. |
9 | Iterator iterator( ) Returns an iterator for the invoking collection. |
10 | boolean remove(Object obj) Removes one instance of obj from the invoking collection. Returns true if the element was removed. Otherwise, returns false. |
11 | boolean removeAll(Collection c) Removes all elements of c from the invoking collection. Returns true if the collection changed (i.e., elements were removed). Otherwise, returns false. |
12 | boolean retainAll(Collection c) Removes all elements from the invoking collection except those in c. Returns true if the collection changed (i.e., elements were removed). Otherwise, returns false. |
13 | int size( ) Returns the number of elements held in the invoking collection. |
14 | Object[ ] toArray( ) Returns an array that contains all the elements stored in the invoking collection. The array elements are copies of the collection elements. |
15 | Object[ ] toArray(Object array[ ]) Returns an array containing only those collection elements whose type matches that of array. |
Example of Collection Interface in Java
Following is an example to explain few methods from various class implementations of the above collection methods −
import java.util.*; public class CollectionsDemo { public static void main(String[] args) { // ArrayList List a1 = new ArrayList(); a1.add("Zara"); a1.add("Mahnaz"); a1.add("Ayan"); System.out.println(" ArrayList Elements"); System.out.print("\t" + a1); // LinkedList List l1 = new LinkedList(); l1.add("Zara"); l1.add("Mahnaz"); l1.add("Ayan"); System.out.println(); System.out.println(" LinkedList Elements"); System.out.print("\t" + l1); // HashSet Set s1 = new HashSet(); s1.add("Zara"); s1.add("Mahnaz"); s1.add("Ayan"); System.out.println(); System.out.println(" Set Elements"); System.out.print("\t" + s1); // HashMap Map m1 = new HashMap(); m1.put("Zara", "8"); m1.put("Mahnaz", "31"); m1.put("Ayan", "12"); m1.put("Daisy", "14"); System.out.println(); System.out.println(" Map Elements"); System.out.print("\t" + m1); } }
This will produce the following result −
Output
ArrayList Elements [Zara, Mahnaz, Ayan] LinkedList Elements [Zara, Mahnaz, Ayan] Set Elements [Ayan, Zara, Mahnaz] Map Elements {Daisy = 14, Ayan = 12, Zara = 8, Mahnaz = 31}