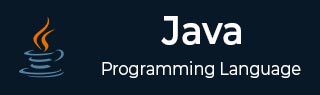
Java Tutorial
- Java - Home
- Java - Overview
- Java - History
- Java - Features
- Java vs C++
- Java Virtual Machine (JVM)
- Java - JDK vs JRE vs JVM
- Java - Hello World Program
- Java - Environment Setup
- Java - Basic Syntax
- Java - Variable Types
- Java - Data Types
- Java - Type Casting
- Java - Unicode System
- Java - Basic Operators
- Java - Comments
- Java - User Input
Java Control Statements
- Java - Loop Control
- Java - Decision Making
- Java - If-else
- Java - Switch
- Java - For Loops
- Java - For-Each Loops
- Java - While Loops
- Java - do-while Loops
- Java - Break
- Java - Continue
Object Oriented Programming
- Java - OOPs Concepts
- Java - Object & Classes
- Java - Class Attributes
- Java - Class Methods
- Java - Methods
- Java - Variables Scope
- Java - Constructors
- Java - Access Modifiers
- Java - Inheritance
- Java - Aggregation
- Java - Polymorphism
- Java - Overriding
- Java - Method Overloading
- Java - Dynamic Binding
- Java - Static Binding
- Java - Instance Initializer Block
- Java - Abstraction
- Java - Encapsulation
- Java - Interfaces
- Java - Packages
- Java - Inner Classes
- Java - Static Class
- Java - Anonymous Class
- Java - Singleton Class
- Java - Wrapper Classes
- Java - Enums
- Java - Enum Constructor
- Java - Enum Strings
Java Built-in Classes
- Java - Number
- Java - Boolean
- Java - Characters
- Java - Strings
- Java - Arrays
- Java - Date & Time
- Java - Math Class
Java File Handling
- Java - Files
- Java - Create a File
- Java - Write to File
- Java - Read Files
- Java - Delete Files
- Java - Directories
- Java - I/O Streams
Java Error & Exceptions
- Java - Exceptions
- Java - try-catch Block
- Java - try-with-resources
- Java - Multi-catch Block
- Java - Nested try Block
- Java - Finally Block
- Java - throw Exception
- Java - Exception Propagation
- Java - Built-in Exceptions
- Java - Custom Exception
Java Multithreading
- Java - Multithreading
- Java - Thread Life Cycle
- Java - Creating a Thread
- Java - Starting a Thread
- Java - Joining Threads
- Java - Naming Thread
- Java - Thread Scheduler
- Java - Thread Pools
- Java - Main Thread
- Java - Thread Priority
- Java - Daemon Threads
- Java - Thread Group
- Java - Shutdown Hook
Java Synchronization
- Java - Synchronization
- Java - Block Synchronization
- Java - Static Synchronization
- Java - Inter-thread Communication
- Java - Thread Deadlock
- Java - Interrupting a Thread
- Java - Thread Control
- Java - Reentrant Monitor
Java Networking
- Java - Networking
- Java - Socket Programming
- Java - URL Processing
- Java - URL Class
- Java - URLConnection Class
- Java - HttpURLConnection Class
- Java - Socket Class
- Java - Generics
Java Collections
Java List Interface
Java Queue Interface
Java Map Interface
- Java - Map Interface
- Java - HashMap
- Java - LinkedHashMap
- Java - WeakHashMap
- Java - EnumMap
- Java - SortedMap Interface
- Java - TreeMap
- Java - The IdentityHashMap Class
Java Set Interface
- Java - Set Interface
- Java - HashSet
- Java - EnumSet
- Java - LinkedHashSet
- Java - SortedSet Interface
- Java - TreeSet
Java Data Structures
- Java - Data Structures
- Java - Enumeration
- Java - BitSet Class
- Java - Dictionary
- Java - Hashtable
- Java - Properties
Java Collections Algorithms
Advanced Java
- Java - Command-Line Arguments
- Java - Lambda Expressions
- Java - Sending Email
- Java - Applet Basics
- Java - Javadoc Comments
- Java - Autoboxing and Unboxing
- Java - File Mismatch Method
- Java - REPL (JShell)
- Java - Multi-Release Jar Files
- Java - Private Interface Methods
- Java - Inner Class Diamond Operator
- Java - Multiresolution Image API
- Java - Collection Factory Methods
- Java - Module System
- Java - Nashorn JavaScript
- Java - Optional Class
- Java - Method References
- Java - Functional Interfaces
- Java - Default Methods
- Java - Base64 Encode Decode
- Java - Switch Expressions
- Java - Teeing Collectors
- Java - Microbenchmark
- Java - Text Blocks
- Java - Null Pointer Exception
- Java - Packaging Tools
- Java - Sealed Classes
- Java - Record Classes
- Java - Hidden Classes
- Java - Compact Number Formatting
- Java - Garbage Collection
- Java - JIT Compiler
Java Miscellaneous
- Java - Recursion
- Java - Regular Expressions
- Java - Serialization
- Java - Strings
- Java - Process API Improvements
- Java - Stream API Improvements
- Java - Enhanced @Deprecated Annotation
- Java - CompletableFuture API Improvements
- Java - Array Methods
- Java - Streams
- Java - Datetime Api
- Java 8 - New Features
- Java 9 - New Features
- Java 10 - New Features
- Java 11 - New Features
- Java 12 - New Features
Java APIs & Frameworks
Java Useful Resources
Java - Enum String
Java enum is a special construct to represents a group of pre-defined constant strings and provides clarity in code while used as constants in application code. By default, an enum string representation is the same as its declaration. Consider the following example:
enum WEEKDAY { MONDAY, TUESDAY, WEDNESDAY, THRUSDAY, FRIDAY, SATURDAY, SUNDAY }
If we print the string representation of the above enum using the enum directly, using toString(), or using the name() method, it will print the same string as declared.
System.out.println(WEEKDAY.MONDAY); System.out.println(WEEKDAY.MONDAY.toString()); System.out.println(WEEKDAY.MONDAY.name());
It will print the result as shown below:
MONDAY MONDAY MONDAY
Overriding Enum toString() Method
Now in case, we want to change the default string representation to the enum's string representation, we can create an overridden toString() method for each value of the enum constructor as shown below:
enum WEEKDAY { MONDAY{ // overridden toString() method per value public String toString() { return "Day 1 of the Week: Monday"; } }; // or override toString() per enum // priority will be given to value level toString() method. public String toString() { return "Day 1 of the Week: Monday"; } }
In this case, we're overriding a default toString() method of the enum to give a custom description.
Example: Overriding toString() Method in Java
In this example, we've created an enum WEEKDAY. Using toString() method, we're setting a custom description of enum value.
package com.tutorialspoint; enum WEEKDAY { // enum value constants MONDAY, TUESDAY, WEDNESDAY, THRUSDAY, FRIDAY, SATURDAY, SUNDAY; // override the toString() method for custom description @Override public String toString() { return switch(this) { case MONDAY: yield "Day 1"; case TUESDAY:yield "Day 2"; case WEDNESDAY:yield "Day 3"; case THRUSDAY:yield "Day 4"; case FRIDAY:yield "Day 5"; case SATURDAY:yield "DAY 6"; case SUNDAY: yield "Day 7"; }; } } public class Tester { public static void main(String[] args) { // invoke toString() internally System.out.println(WEEKDAY.MONDAY); // invoke toString explicitly System.out.println(WEEKDAY.TUESDAY.toString()); // invoke name() method to get the default name System.out.println(WEEKDAY.WEDNESDAY.name()); } }
Output
Let us compile and run the above program, this will produce the following result −
Day 1 Day 2 WEDNESDAY
Example: Overriding toString() Method Per Value in Java
In this example, we've overridden toString() methods per value of this enum WEEKDAY. This way we can customize string representation per value in this way as well.
package com.tutorialspoint; enum WEEKDAY { // override the toString() method for custom description MONDAY{ @Override public String toString() { return "Day 1"; } }, TUESDAY{ @Override public String toString() { return "Day 2"; } }, WEDNESDAY{ @Override public String toString() { return "Day 3"; } }, THRUSDAY{ @Override public String toString() { return "Day 4"; } }, FRIDAY{ @Override public String toString() { return "Day 5"; } }, SATURDAY{ @Override public String toString() { return "Day 6"; } }, SUNDAY{ @Override public String toString() { return "Day 7"; } }; } public class Tester { public static void main(String[] args) { // invoke toString() internally System.out.println(WEEKDAY.MONDAY); // invoke toString explicitly System.out.println(WEEKDAY.TUESDAY.toString()); // invoke name() method to get the default name System.out.println(WEEKDAY.WEDNESDAY.name()); } }
Output
Let us compile and run the above program, this will produce the following result −
Day 1 Day 2 WEDNESDAY
enum name() method is final and cannot be overridden. It can be used to get the default name of the enum while string representation of enum is overridden by toString() method.