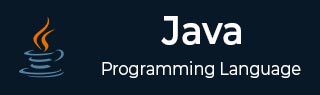
Java Tutorial
- Java - Home
- Java - Overview
- Java - History
- Java - Features
- Java vs C++
- Java Virtual Machine (JVM)
- Java - JDK vs JRE vs JVM
- Java - Hello World Program
- Java - Environment Setup
- Java - Basic Syntax
- Java - Variable Types
- Java - Data Types
- Java - Type Casting
- Java - Unicode System
- Java - Basic Operators
- Java - Comments
- Java - User Input
Java Control Statements
- Java - Loop Control
- Java - Decision Making
- Java - If-else
- Java - Switch
- Java - For Loops
- Java - For-Each Loops
- Java - While Loops
- Java - do-while Loops
- Java - Break
- Java - Continue
Object Oriented Programming
- Java - OOPs Concepts
- Java - Object & Classes
- Java - Class Attributes
- Java - Class Methods
- Java - Methods
- Java - Variables Scope
- Java - Constructors
- Java - Access Modifiers
- Java - Inheritance
- Java - Aggregation
- Java - Polymorphism
- Java - Overriding
- Java - Method Overloading
- Java - Dynamic Binding
- Java - Static Binding
- Java - Instance Initializer Block
- Java - Abstraction
- Java - Encapsulation
- Java - Interfaces
- Java - Packages
- Java - Inner Classes
- Java - Static Class
- Java - Anonymous Class
- Java - Singleton Class
- Java - Wrapper Classes
- Java - Enums
- Java - Enum Constructor
- Java - Enum Strings
Java Built-in Classes
- Java - Number
- Java - Boolean
- Java - Characters
- Java - Strings
- Java - Arrays
- Java - Date & Time
- Java - Math Class
Java File Handling
- Java - Files
- Java - Create a File
- Java - Write to File
- Java - Read Files
- Java - Delete Files
- Java - Directories
- Java - I/O Streams
Java Error & Exceptions
- Java - Exceptions
- Java - try-catch Block
- Java - try-with-resources
- Java - Multi-catch Block
- Java - Nested try Block
- Java - Finally Block
- Java - throw Exception
- Java - Exception Propagation
- Java - Built-in Exceptions
- Java - Custom Exception
Java Multithreading
- Java - Multithreading
- Java - Thread Life Cycle
- Java - Creating a Thread
- Java - Starting a Thread
- Java - Joining Threads
- Java - Naming Thread
- Java - Thread Scheduler
- Java - Thread Pools
- Java - Main Thread
- Java - Thread Priority
- Java - Daemon Threads
- Java - Thread Group
- Java - Shutdown Hook
Java Synchronization
- Java - Synchronization
- Java - Block Synchronization
- Java - Static Synchronization
- Java - Inter-thread Communication
- Java - Thread Deadlock
- Java - Interrupting a Thread
- Java - Thread Control
- Java - Reentrant Monitor
Java Networking
- Java - Networking
- Java - Socket Programming
- Java - URL Processing
- Java - URL Class
- Java - URLConnection Class
- Java - HttpURLConnection Class
- Java - Socket Class
- Java - Generics
Java Collections
Java List Interface
Java Queue Interface
Java Map Interface
- Java - Map Interface
- Java - HashMap
- Java - LinkedHashMap
- Java - WeakHashMap
- Java - EnumMap
- Java - SortedMap Interface
- Java - TreeMap
- Java - The IdentityHashMap Class
Java Set Interface
- Java - Set Interface
- Java - HashSet
- Java - EnumSet
- Java - LinkedHashSet
- Java - SortedSet Interface
- Java - TreeSet
Java Data Structures
- Java - Data Structures
- Java - Enumeration
- Java - BitSet Class
- Java - Dictionary
- Java - Hashtable
- Java - Properties
Java Collections Algorithms
Advanced Java
- Java - Command-Line Arguments
- Java - Lambda Expressions
- Java - Sending Email
- Java - Applet Basics
- Java - Javadoc Comments
- Java - Autoboxing and Unboxing
- Java - File Mismatch Method
- Java - REPL (JShell)
- Java - Multi-Release Jar Files
- Java - Private Interface Methods
- Java - Inner Class Diamond Operator
- Java - Multiresolution Image API
- Java - Collection Factory Methods
- Java - Module System
- Java - Nashorn JavaScript
- Java - Optional Class
- Java - Method References
- Java - Functional Interfaces
- Java - Default Methods
- Java - Base64 Encode Decode
- Java - Switch Expressions
- Java - Teeing Collectors
- Java - Microbenchmark
- Java - Text Blocks
- Java - Null Pointer Exception
- Java - Packaging Tools
- Java - Sealed Classes
- Java - Record Classes
- Java - Hidden Classes
- Java - Compact Number Formatting
- Java - Garbage Collection
- Java - JIT Compiler
Java Miscellaneous
- Java - Recursion
- Java - Regular Expressions
- Java - Serialization
- Java - Strings
- Java - Process API Improvements
- Java - Stream API Improvements
- Java - Enhanced @Deprecated Annotation
- Java - CompletableFuture API Improvements
- Java - Array Methods
- Java - Streams
- Java - Datetime Api
- Java 8 - New Features
- Java 9 - New Features
- Java 10 - New Features
- Java 11 - New Features
- Java 12 - New Features
Java APIs & Frameworks
Java Useful Resources
Java - The TreeMap Class
The TreeMap class implements the Map interface by using a tree. A TreeMap provides an efficient means of storing key/value pairs in sorted order, and allows rapid retrieval.
You should note that, unlike a hash map, a tree map guarantees that its elements will be sorted in an ascending key order.
Following is the list of the constructors supported by the TreeMap class.
Sr.No. | Constructors & Description |
---|---|
1 | TreeMap( ) This constructor constructs an empty tree map that will be sorted using the natural order of its keys. |
2 | TreeMap(Comparator comp) This constructor constructs an empty tree-based map that will be sorted using the Comparator comp. |
3 | TreeMap(Map m) This constructor initializes a tree map with the entries from m, which will be sorted using the natural order of the keys. |
4 | TreeMap(SortedMap sm) This constructor initializes a tree map with the entries from the SortedMap sm, which will be sorted in the same order as sm. |
Apart from the methods inherited from its parent classes, TreeMap defines the following methods −
Sr.No. | Method & Description |
---|---|
1 | void clear() Removes all mappings from this TreeMap. |
2 | Object clone() Returns a shallow copy of this TreeMap instance. |
3 | Comparator comparator() Returns the comparator used to order this map, or null if this map uses its keys' natural order. |
4 | boolean containsKey(Object key) Returns true if this map contains a mapping for the specified key. |
5 | boolean containsValue(Object value) Returns true if this map maps one or more keys to the specified value. |
6 | Set entrySet() Returns a set view of the mappings contained in this map. |
7 | Object firstKey() Returns the first (lowest) key currently in this sorted map. |
8 | Object get(Object key) Returns the value to which this map maps the specified key. |
9 | SortedMap headMap(Object toKey) Returns a view of the portion of this map whose keys are strictly less than toKey. |
10 | Set keySet() Returns a Set view of the keys contained in this map. |
11 | Object lastKey() Returns the last (highest) key currently in this sorted map. |
12 | Object put(Object key, Object value) Associates the specified value with the specified key in this map. |
13 | void putAll(Map map) Copies all of the mappings from the specified map to this map. |
14 | Object remove(Object key) Removes the mapping for this key from this TreeMap if present. |
15 | int size() Returns the number of key-value mappings in this map. |
16 | SortedMap subMap(Object fromKey, Object toKey) Returns a view of the portion of this map whose keys range from fromKey, inclusive, to toKey, exclusive. |
17 | SortedMap tailMap(Object fromKey) Returns a view of the portion of this map whose keys are greater than or equal to fromKey. |
18 | Collection values() Returns a collection view of the values contained in this map. |
Example
The following program illustrates several of the methods supported by this collection −
import java.util.*; public class TreeMapDemo { public static void main(String args[]) { // Create a hash map TreeMap tm = new TreeMap(); // Put elements to the map tm.put("Zara", new Double(3434.34)); tm.put("Mahnaz", new Double(123.22)); tm.put("Ayan", new Double(1378.00)); tm.put("Daisy", new Double(99.22)); tm.put("Qadir", new Double(-19.08)); // Get a set of the entries Set set = tm.entrySet(); // Get an iterator Iterator i = set.iterator(); // Display elements while(i.hasNext()) { Map.Entry me = (Map.Entry)i.next(); System.out.print(me.getKey() + ": "); System.out.println(me.getValue()); } System.out.println(); // Deposit 1000 into Zara's account double balance = ((Double)tm.get("Zara")).doubleValue(); tm.put("Zara", new Double(balance + 1000)); System.out.println("Zara's new balance: " + tm.get("Zara")); } }
This will produce the following result −
Output
Ayan: 1378.0 Daisy: 99.22 Mahnaz: 123.22 Qadir: -19.08 Zara: 3434.34 Zara's new balance: 4434.34