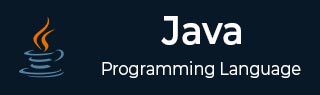
Java Tutorial
- Java - Home
- Java - Overview
- Java - History
- Java - Features
- Java vs C++
- Java Virtual Machine (JVM)
- Java - JDK vs JRE vs JVM
- Java - Hello World Program
- Java - Environment Setup
- Java - Basic Syntax
- Java - Variable Types
- Java - Data Types
- Java - Type Casting
- Java - Unicode System
- Java - Basic Operators
- Java - Comments
- Java - User Input
Java Control Statements
- Java - Loop Control
- Java - Decision Making
- Java - If-else
- Java - Switch
- Java - For Loops
- Java - For-Each Loops
- Java - While Loops
- Java - do-while Loops
- Java - Break
- Java - Continue
Object Oriented Programming
- Java - OOPs Concepts
- Java - Object & Classes
- Java - Class Attributes
- Java - Class Methods
- Java - Methods
- Java - Variables Scope
- Java - Constructors
- Java - Access Modifiers
- Java - Inheritance
- Java - Aggregation
- Java - Polymorphism
- Java - Overriding
- Java - Method Overloading
- Java - Dynamic Binding
- Java - Static Binding
- Java - Instance Initializer Block
- Java - Abstraction
- Java - Encapsulation
- Java - Interfaces
- Java - Packages
- Java - Inner Classes
- Java - Static Class
- Java - Anonymous Class
- Java - Singleton Class
- Java - Wrapper Classes
- Java - Enums
- Java - Enum Constructor
- Java - Enum Strings
Java Built-in Classes
- Java - Number
- Java - Boolean
- Java - Characters
- Java - Strings
- Java - Arrays
- Java - Date & Time
- Java - Math Class
Java File Handling
- Java - Files
- Java - Create a File
- Java - Write to File
- Java - Read Files
- Java - Delete Files
- Java - Directories
- Java - I/O Streams
Java Error & Exceptions
- Java - Exceptions
- Java - try-catch Block
- Java - try-with-resources
- Java - Multi-catch Block
- Java - Nested try Block
- Java - Finally Block
- Java - throw Exception
- Java - Exception Propagation
- Java - Built-in Exceptions
- Java - Custom Exception
Java Multithreading
- Java - Multithreading
- Java - Thread Life Cycle
- Java - Creating a Thread
- Java - Starting a Thread
- Java - Joining Threads
- Java - Naming Thread
- Java - Thread Scheduler
- Java - Thread Pools
- Java - Main Thread
- Java - Thread Priority
- Java - Daemon Threads
- Java - Thread Group
- Java - Shutdown Hook
Java Synchronization
- Java - Synchronization
- Java - Block Synchronization
- Java - Static Synchronization
- Java - Inter-thread Communication
- Java - Thread Deadlock
- Java - Interrupting a Thread
- Java - Thread Control
- Java - Reentrant Monitor
Java Networking
- Java - Networking
- Java - Socket Programming
- Java - URL Processing
- Java - URL Class
- Java - URLConnection Class
- Java - HttpURLConnection Class
- Java - Socket Class
- Java - Generics
Java Collections
Java List Interface
Java Queue Interface
Java Map Interface
- Java - Map Interface
- Java - HashMap
- Java - LinkedHashMap
- Java - WeakHashMap
- Java - EnumMap
- Java - SortedMap Interface
- Java - TreeMap
- Java - The IdentityHashMap Class
Java Set Interface
- Java - Set Interface
- Java - HashSet
- Java - EnumSet
- Java - LinkedHashSet
- Java - SortedSet Interface
- Java - TreeSet
Java Data Structures
- Java - Data Structures
- Java - Enumeration
- Java - BitSet Class
- Java - Dictionary
- Java - Hashtable
- Java - Properties
Java Collections Algorithms
Advanced Java
- Java - Command-Line Arguments
- Java - Lambda Expressions
- Java - Sending Email
- Java - Applet Basics
- Java - Javadoc Comments
- Java - Autoboxing and Unboxing
- Java - File Mismatch Method
- Java - REPL (JShell)
- Java - Multi-Release Jar Files
- Java - Private Interface Methods
- Java - Inner Class Diamond Operator
- Java - Multiresolution Image API
- Java - Collection Factory Methods
- Java - Module System
- Java - Nashorn JavaScript
- Java - Optional Class
- Java - Method References
- Java - Functional Interfaces
- Java - Default Methods
- Java - Base64 Encode Decode
- Java - Switch Expressions
- Java - Teeing Collectors
- Java - Microbenchmark
- Java - Text Blocks
- Java - Null Pointer Exception
- Java - Packaging Tools
- Java - Sealed Classes
- Java - Record Classes
- Java - Hidden Classes
- Java - Compact Number Formatting
- Java - Garbage Collection
- Java - JIT Compiler
Java Miscellaneous
- Java - Recursion
- Java - Regular Expressions
- Java - Serialization
- Java - Strings
- Java - Process API Improvements
- Java - Stream API Improvements
- Java - Enhanced @Deprecated Annotation
- Java - CompletableFuture API Improvements
- Java - Array Methods
- Java - Streams
- Java - Datetime Api
- Java 8 - New Features
- Java 9 - New Features
- Java 10 - New Features
- Java 11 - New Features
- Java 12 - New Features
Java APIs & Frameworks
Java Useful Resources
Java - The Map.Entry Interface
The Map.Entry interface enables you to work with a map entry.
The entrySet( ) method declared by the Map interface returns a Set containing the map entries. Each of these set elements is a Map.Entry object.
Following table summarizes the methods declared by this interface −
Sr.No. | Method & Description |
---|---|
1 | boolean equals(Object obj) Returns true if obj is a Map.Entry whose key and value are equal to that of the invoking object. |
2 | Object getKey( ) Returns the key for this map entry. |
3 | Object getValue( ) Returns the value for this map entry. |
4 | int hashCode( ) Returns the hash code for this map entry. |
5 | Object setValue(Object v) Sets the value for this map entry to v. A ClassCastException is thrown if v is not the correct type for the map. A NullPointerException is thrown if v is null and the map does not permit null keys. An UnsupportedOperationException is thrown if the map cannot be changed. |
Example 1
Following is an example showing how Map.Entry can be used to get values of a map entry −
import java.util.HashMap; import java.util.Iterator; import java.util.Map; import java.util.Set; public class HashMapDemo { public static void main(String args[]) { // Create a hash map HashMap<String, Double> hm = new HashMap<>(); // Put elements to the map hm.put("Zara", Double.valueOf(3434.34)); hm.put("Mahnaz", Double.valueOf(123.22)); hm.put("Ayan", Double.valueOf(1378.00)); hm.put("Daisy", Double.valueOf(99.22)); hm.put("Qadir", Double.valueOf(-19.08)); // Get a set of the entries Set<Map.Entry<String, Double>> set = hm.entrySet(); // Get an iterator Iterator<Map.Entry<String, Double>> i = set.iterator(); // Display elements while(i.hasNext()) { Map.Entry<String, Double> me = i.next(); System.out.print(me.getKey() + ": "); System.out.println(me.getValue()); } } }
Output
Daisy: 99.22 Ayan: 1378.0 Zara: 3434.34 Qadir: -19.08 Mahnaz: 123.22
Example 2
Following is an example showing how Map.Entry can be used to set values of a map entry −
import java.util.HashMap; import java.util.Iterator; import java.util.Map; import java.util.Set; public class HashMapDemo { public static void main(String args[]) { // Create a hash map HashMap<String, Double> hm = new HashMap<>(); // Put elements to the map hm.put("Zara", Double.valueOf(3434.34)); hm.put("Mahnaz", Double.valueOf(123.22)); hm.put("Ayan", Double.valueOf(1378.00)); hm.put("Daisy", Double.valueOf(99.22)); hm.put("Qadir", Double.valueOf(-19.08)); // Get a set of the entries Set<Map.Entry<String, Double>> set = hm.entrySet(); // Get an iterator Iterator<Map.Entry<String, Double>> i = set.iterator(); // Display elements while(i.hasNext()) { Map.Entry<String, Double> me = i.next(); me.setValue(me.getValue() * 10); System.out.print(me.getKey() + ": "); System.out.println(me.getValue()); } } }
Output
Daisy: 992.2 Ayan: 13780.0 Zara: 34343.4 Qadir: -190.79999999999998 Mahnaz: 1232.2
Example 3
Following is an example showing how Map.Entry can be used to get key of a map entry −
import java.util.HashMap; import java.util.Iterator; import java.util.Map; import java.util.Set; public class HashMapDemo { public static void main(String args[]) { // Create a hash map HashMap<String, Double> hm = new HashMap<>(); // Put elements to the map hm.put("Zara", Double.valueOf(3434.34)); hm.put("Mahnaz", Double.valueOf(123.22)); hm.put("Ayan", Double.valueOf(1378.00)); hm.put("Daisy", Double.valueOf(99.22)); hm.put("Qadir", Double.valueOf(-19.08)); // Get a set of the entries Set<Map.Entry<String, Double>> set = hm.entrySet(); // Get an iterator Iterator<Map.Entry<String, Double>> i = set.iterator(); // Display elements while(i.hasNext()) { Map.Entry<String, Double> me = i.next(); System.out.println(me.getKey()); } } }
Output
Daisy Ayan Zara Qadir Mahnaz