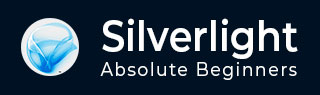
- Silverlight Tutorial
- Silverlight - Home
- Silverlight - Overview
- Silverlight - Environment Setup
- Silverlight - Getting Started
- Silverlight - XAML Overview
- Silverlight - Project Types
- Silverlight - Fixed Layouts
- Silverlight - Dynamic Layout
- Constrained vs. Unconstrained
- Silverlight - CSS
- Silverlight - Controls
- Silverlight - Buttons
- Silverlight - Content Model
- Silverlight - ListBox
- Silverlight - Templates
- Silverlight - Visual State
- Silverlight - Data Binding
- Silverlight - Browser Integration
- Silverlight - Out-of-Browser
- Silverlight - Applications, Resources
- Silverlight - File Access
- Silverlight - View Model
- Silverlight - Input Handling
- Silverlight - Isolated Storage
- Silverlight - Text
- Silverlight - Animation
- Silverlight - Video and Audio
- Silverlight - Printing
- Silverlight Useful Resources
- Silverlight - Quick Guide
- Silverlight - Useful Resources
- Silverlight - Discussion
Silverlight - File Access
In this chapter, we will see how Silverlight applications can access files on the end user's computer. There are three main ways to access files in Silverlight. The choice will depend on the reason you need to use files, and on whether you are writing a trusted application.
The most flexible option is to use the file dialog classes. With the Open and Save file dialogs, you can get access to any file that the end user chooses, as long as the user has appropriate permissions. User consent is central to this approach. The user has to choose which file to read, or when saving, they pick a file to overwrite or pick a location and a file name for you.
The second option is to use the various classes in the System.IO namespace. Silverlight offers classes such as FileStream, StreamWriter, FileInfo, Directory, and DirectoryInfo, all of which make it possible to write code that opens and accesses files without needing to get the user involved. That may be more convenient for the developer, but of course, most users would not want any old code downloaded as part of a web page to be able to search around in their files.
The third option is Isolated Storage, which we will discuss later.
Open & Save File Dialogs
SaveFileDialog
The SaveFileDialog class shows the standard operating system supplied user interface for choosing where to save a file.
Some important features are −
To use it, we create an instance of the SaveFileDialog class.
Calling ShowDialog, causes it to appear, and the return code tells us whether the user selected a place to save the file, or cancelled the dialog.
You might be wondering about the redundant-looking comparison with True there. If ShowDialog returns True value, which means the user has selected a file. So we can go on to call the OpenFile method, which returns us a Stream.
If we want to, we can discover the name the user chose. The dialog provides a property called SafeFileName, but that does not include the path. In any case, the only way to write data is to use the Stream returned by the dialog. From a developer’s perspective, this is just an ordinary .NET stream, so we can wrap it in a StreamWriter, to write text into it.
OpenFileDialog
The OpenFileDialog is similar in use to the SaveFileDialog. Obviously, you are always picking an existing file rather than a new one, but there is another important difference.
It offers a property called MultiSelect. If you set that to True, the user can choose multiple files. This means the dialog needs a slightly more complex API.
The SaveFileDialog only deals with one file at a time, but OpenFileDialog is able to cope with more, so it does not offer an OpenFile method. We need to expand the code. Depending on whether the dialog is in single file mode, or MultiSelect mode, you use either its File, or Files property.
Here, in the below given example, we are in single file mode. Hence, we use File, and we call OpenRead on the FileInfo object that returns.
In multiselect mode, we would use Files instead, which returns a collection of FileInfo objects.
FileStream
The second approach to file access as mentioned above is to use the FileStream class, or related types in the System.IO namespace directly. There is not very much to say about this, because for the most part, it is similar to file access with the full .NET Framework.
However, there are a couple of Silverlight-specific twists.
First, this approach lets you access files at any time without user intervention, and without any obvious visible indication of file activity, only trusted applications are allowed to use this technique. Remember, you need to run out of browser to get elevated trust.
The second issue is that only files in certain specific folders are available. You can only read and write files that are under the User's Documents, Music, Pictures, or Video files. One reason for this is that Silverlight runs on multiple platforms, and the file system structure for, say, an Apple Mac, is very different from that of Windows. Hence, cross-platform file access has to work in terms of a limited set of folders that are available on all systems Silverlight supports.
Since these folders will be in different locations on different operating systems, and their location will typically vary from one user to another, you need to use the Environment.GetFolderPath method to discover the actual location at runtime.
You can inspect the directory structure beneath the starting points. The Directory and DirectoryInfo classes in the System.IO namespace lets you enumerate files and directories.
Consider a simple example in which file can open via OpenFileDialog and save some text to the file via SaveFileDialog.
Given below is the XAML code in which two buttons and a text box are created.
<UserControl x:Class = "FileDialogs.MainPage" xmlns = "http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x = "http://schemas.microsoft.com/winfx/2006/xaml" xmlns:d = "http://schemas.microsoft.com/expression/blend/2008" xmlns:mc = "http://schemas.openxmlformats.org/markup-compatibility/2006" mc:Ignorable = "d" d:DesignHeight = "300" d:DesignWidth = "400"> <Grid x:Name = "LayoutRoot" Background = "White"> <Grid.RowDefinitions> <RowDefinition Height = "Auto" /> <RowDefinition Height = "265*" /> </Grid.RowDefinitions> <Button x:Name = "saveFileButton" Content = "Save" Width = "75" FontSize = "20" HorizontalAlignment = "Left" VerticalAlignment = "Top" Margin = "12,12" Click = "saveFileButton_Click" /> <Button x:Name = "openFileButton" Content = "Open" Width = "75" FontSize = "20" HorizontalAlignment = "Left" VerticalAlignment = "Top" Margin = "101,12,0,0" Click = "openFileButton_Click" /> <TextBox x:Name = "contentTextBox" Grid.Row = "1" Margin = "12" FontSize = "20" /> </Grid> </UserControl>
Given below is C# code for click events implementation in which file is opened and saved.
using System; using System.Diagnostics; using System.IO; using System.Windows; using System.Windows.Controls; namespace FileDialogs { public partial class MainPage : UserControl { public MainPage() { InitializeComponent(); } private void saveFileButton_Click(object sender, RoutedEventArgs e) { var save = new SaveFileDialog(); save.Filter = "Text Files (*.txt)|*.txt|All Files (*.*)|*.*"; save.DefaultExt = ".txt"; if (save.ShowDialog() == true) { Debug.WriteLine(save.SafeFileName); using (Stream saveStream = save.OpenFile()) using (var w = new StreamWriter(saveStream)) { var fs = saveStream as FileStream; if (fs != null) { w.Write(contentTextBox.Text); } } } } private void openFileButton_Click(object sender, RoutedEventArgs e) { var open = new OpenFileDialog(); if (open.ShowDialog() == true) { using (Stream openStream = open.File.OpenRead()) { using (var read = new StreamReader(openStream)) { contentTextBox.Text = read.ReadToEnd(); } } } } } }
When the above code is compiled and executed, you will see the following webpage, which contains two buttons.
Click the Open button, which will open OpenFileDialog to select a text file.
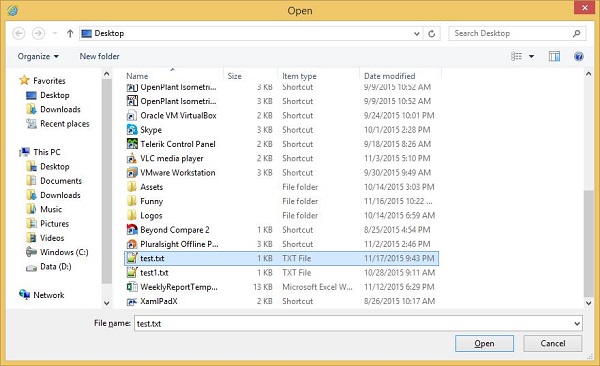
Select a text file and click Open, you will see the text on textbox.
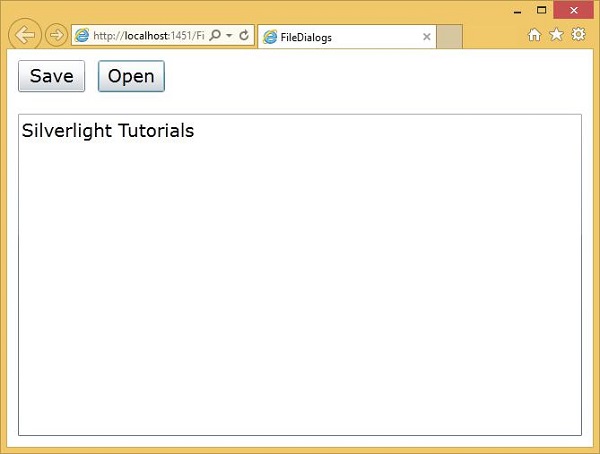
To save text to the file, update the text.
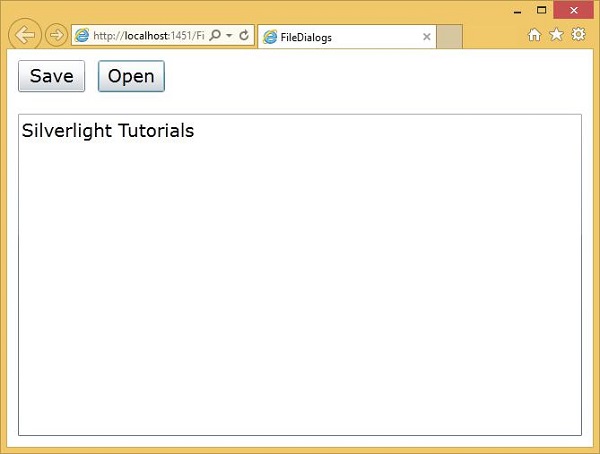
Click the Save button to save the changes to either new text file or existing file.
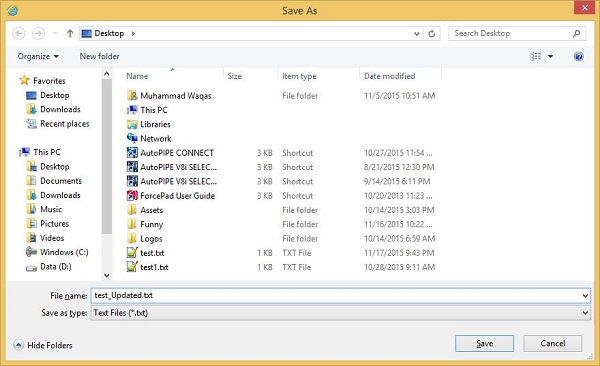
To save changes to the existing text file, select the text file in SaveFileDialog, but if you want to save changes to the new file write the file name and click the Save button.
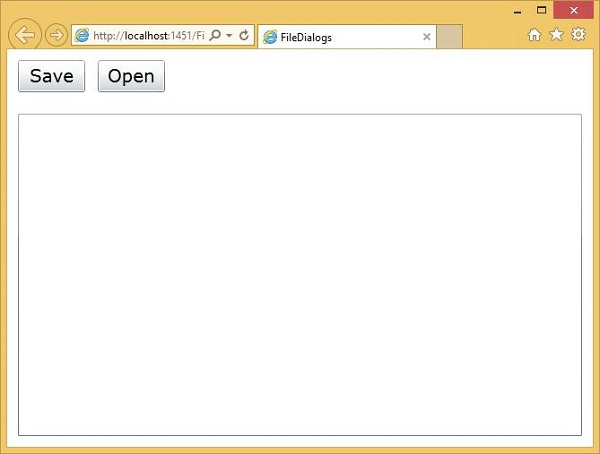