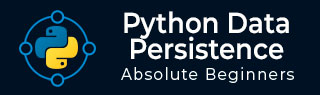
- Python Data Persistence Tutorial
- Python Data Persistence - Home
- Python Data Persistence - Introduction
- Python Data Persistence - File API
- File Handling with os Module
- Python Data Persistence - Object Serialization
- Python Data Persistence - Pickle Module
- Python Data Persistence - Marshal Module
- Python Data Persistence - Shelve Module
- Python Data Persistence - dbm Package
- Python Data Persistence - CSV Module
- Python Data Persistence - JSON Module
- Python Data Persistence - XML Parsers
- Python Data Persistence - Plistlib Module
- Python Data Persistence - Sqlite3 Module
- Python Data Persistence - SQLAlchemy
- Python Data Persistence - PyMongo module
- Python Data Persistence - Cassandra Driver
- Data Persistence - ZODB
- Data Persistence - Openpyxl Module
- Python Data Persistence Resources
- Python Data Persistence - Quick Guide
- Python Data Persistence - Useful Resources
- Python Data Persistence - Discussion
Python Data Persistence - JSON Module
JSON stands for JavaScript Object Notation. It is a lightweight data interchange format. It is a language-independent and cross platform text format, supported by many programming languages. This format is used for data exchange between the web server and clients.
JSON format is similar to pickle. However, pickle serialization is Python specific whereas JSON format is implemented by many languages hence has become universal standard. Functionality and interface of json module in Python’s standard library is similar to pickle and marshal modules.
Just as in pickle module, the json module also provides dumps() and loads() function for serialization of Python object into JSON encoded string, and dump() and load() functions write and read serialized Python objects to/from file.
dumps() − This function converts the object into JSON format.
loads() − This function converts a JSON string back to Python object.
Following example demonstrates basic usage of these functions −
import json data=['Rakesh',{'marks':(50,60,70)}] s=json.dumps(data) json.loads(s)
The dumps() function can take optional sort_keys argument. By default, it is False. If set to True, the dictionary keys appear in sorted order in the JSON string.
The dumps() function has another optional parameter called indent which takes a number as value. It decides length of each segment of formatted representation of json string, similar to print output.
The json module also has object oriented API corresponding to above functions. There are two classes defined in the module – JSONEncoder and JSONDecoder.
JSONEncoder class
Object of this class is encoder for Python data structures. Each Python data type is converted in corresponding JSON type as shown in following table −
Python | JSON |
---|---|
Dict | object |
list, tuple | array |
Str | string |
int, float, int- & float-derived Enums | number |
True | true |
False | false |
None | null |
The JSONEncoder class is instantiated by JSONEncoder() constructor. Following important methods are defined in encoder class −
Sr.No. | Methods & Description |
---|---|
1 |
encode() serializes Python object into JSON format |
2 |
iterencode() Encodes the object and returns an iterator yielding encoded form of each item in the object. |
3 |
indent Determines indent level of encoded string |
4 |
sort_keys is either true or false to make keys appear in sorted order or not. |
5 |
Check_circular if True, check for circular reference in container type object |
Following example encodes Python list object.
e=json.JSONEncoder() e.encode(data)
JSONDecoder class
Object of this class helps in decoded in json string back to Python data structure. Main method in this class is decode(). Following example code retrieves Python list object from encoded string in earlier step.
d=json.JSONDecoder() d.decode(s)
The json module defines load() and dump() functions to write JSON data to a file like object – which may be a disk file or a byte stream and read data back from them.
dump()
This function writes JSONed Python object data to a file. The file must be opened with ‘w’ mode.
import json data=['Rakesh', {'marks': (50, 60, 70)}] fp=open('json.txt','w') json.dump(data,fp) fp.close()
This code will create ‘json.txt’ in current directory. It shows the contents as follows −
["Rakesh", {"marks": [50, 60, 70]}]
load()
This function loads JSON data from the file and returns Python object from it. The file must be opened with read permission (should have ‘r’ mode).
Example
fp=open('json.txt','r') ret=json.load(fp) print (ret) fp.close()
Output
['Rakesh', {'marks': [50, 60, 70]}]
The json.tool module also has a command-line interface that validates data in file and prints JSON object in a pretty formatted manner.
C:\python37>python -m json.tool json.txt [ "Rakesh", { "marks": [ 50, 60, 70 ] } ]