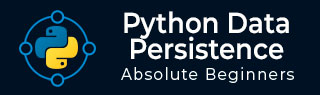
- Python Data Persistence Tutorial
- Python Data Persistence - Home
- Python Data Persistence - Introduction
- Python Data Persistence - File API
- File Handling with os Module
- Python Data Persistence - Object Serialization
- Python Data Persistence - Pickle Module
- Python Data Persistence - Marshal Module
- Python Data Persistence - Shelve Module
- Python Data Persistence - dbm Package
- Python Data Persistence - CSV Module
- Python Data Persistence - JSON Module
- Python Data Persistence - XML Parsers
- Python Data Persistence - Plistlib Module
- Python Data Persistence - Sqlite3 Module
- Python Data Persistence - SQLAlchemy
- Python Data Persistence - PyMongo module
- Python Data Persistence - Cassandra Driver
- Data Persistence - ZODB
- Data Persistence - Openpyxl Module
- Python Data Persistence Resources
- Python Data Persistence - Quick Guide
- Python Data Persistence - Useful Resources
- Python Data Persistence - Discussion
File Handling with os Module
In addition to File object returned by open() function, file IO operations can also be performed using Python's built-in library has os module that provides useful operating system dependent functions. These functions perform low level read/write operations on file.
The open() function from os module is similar to the built-in open(). However, it doesn't return a file object but a file descriptor, a unique integer corresponding to file opened. File descriptor's values 0, 1 and 2 represent stdin, stdout, and stderr streams. Other files will be given incremental file descriptor from 2 onwards.
As in case of open() built-in function, os.open() function also needs to specify file access mode. Following table lists various modes as defined in os module.
Sr.No. | Os Module & Description |
---|---|
1 |
os.O_RDONLY Open for reading only |
2 |
os.O_WRONLY Open for writing only |
3 |
os.O_RDWR Open for reading and writing |
4 |
os.O_NONBLOCK Do not block on open |
5 |
os.O_APPEND Append on each write |
6 |
os.O_CREAT Create file if it does not exist |
7 |
os.O_TRUNC Truncate size to 0 |
8 |
os.O_EXCL Error if create and file exists |
To open a new file for writing data in it, specify O_WRONLY as well as O_CREAT modes by inserting pipe (|) operator. The os.open() function returns a file descriptor.
f=os.open("test.dat", os.O_WRONLY|os.O_CREAT)
Note that, data is written to disk file in the form of byte string. Hence, a normal string is converted to byte string by using encode() function as earlier.
data="Hello World".encode('utf-8')
The write() function in os module accepts this byte string and file descriptor.
os.write(f,data)
Don’t forget to close the file using close() function.
os.close(f)
To read contents of a file using os.read() function, use following statements:
f=os.open("test.dat", os.O_RDONLY) data=os.read(f,20) print (data.decode('utf-8'))
Note that, the os.read() function needs file descriptor and number of bytes to be read (length of byte string).
If you want to open a file for simultaneous read/write operations, use O_RDWR mode. Following table shows important file operation related functions in os module.
Sr.No | Functions & Description |
---|---|
1 |
os.close(fd) Close the file descriptor. |
2 |
os.open(file, flags[, mode]) Open the file and set various flags according to flags and possibly its mode according to mode. |
3 |
os.read(fd, n) Read at most n bytes from file descriptor fd. Return a string containing the bytes read. If the end of the file referred to by fd has been reached, an empty string is returned. |
4 |
os.write(fd, str) Write the string str to file descriptor fd. Return the number of bytes actually written. |