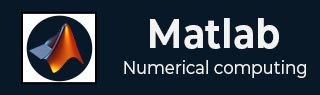
- Matlab Tutorial
- MATLAB - Home
- MATLAB - Overview
- MATLAB - Environment Setup
- MATLAB - Syntax
- MATLAB - Variables
- MATLAB - Commands
- MATLAB - M-Files
- MATLAB - Data Types
- MATLAB - Operators
- MATLAB - Decisions
- MATLAB - Loops
- MATLAB - Vectors
- MATLAB - Matrix
- MATLAB - Arrays
- MATLAB - Colon Notation
- MATLAB - Numbers
- MATLAB - Strings
- MATLAB - Functions
- MATLAB - Data Import
- MATLAB - Data Output
- MATLAB Advanced
- MATLAB - Plotting
- MATLAB - Graphics
- MATLAB - Algebra
- MATLAB - Calculus
- MATLAB - Differential
- MATLAB - Integration
- MATLAB - Polynomials
- MATLAB - Transforms
- MATLAB - GNU Octave
- MATLAB - Simulink
- MATLAB Useful Resources
- MATLAB - Quick Guide
- MATLAB - Useful Resources
- MATLAB - Discussion
MATLAB - Magnitude of a Vector
Magnitude of a vector v with elements v1, v2, v3, …, vn, is given by the equation −
|v| = √(v12 + v22 + v32 + … + vn2)
You need to take the following steps to calculate the magnitude of a vector −
Take the product of the vector with itself, using array multiplication (.*). This produces a vector sv, whose elements are squares of the elements of vector v.
sv = v.*v;
Use the sum function to get the sum of squares of elements of vector v. This is also called the dot product of vector v.
dp= sum(sv);
Use the sqrt function to get the square root of the sum which is also the magnitude of the vector v.
mag = sqrt(s);
Example
Create a script file with the following code −
v = [1: 2: 20]; sv = v.* v; %the vector with elements % as square of v's elements dp = sum(sv); % sum of squares -- the dot product mag = sqrt(dp); % magnitude disp('Magnitude:'); disp(mag);
When you run the file, it displays the following result −
Magnitude: 36.469
matlab_vectors.htm
Advertisements