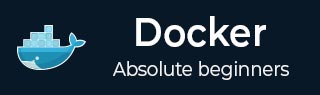
- Docker Tutorial
- Docker - Home
- Docker - Overview
- Docker - Installing Docker on Linux
- Docker - Installation
- Docker - Hub
- Docker - Images
- Docker - Containers
- Docker - Working With Containers
- Docker - Architecture
- Docker - Container & Hosts
- Docker - Configuring
- Docker - Containers & Shells
- Docker - File
- Docker - Building Files
- Docker - Public Repositories
- Docker - Managing Ports
- Docker - Private Registries
- Building a Web Server Docker File
- Docker - Instruction Commands
- Docker - Container Linking
- Docker - Storage
- Docker - Networking
- Docker - Setting Node.js
- Docker - Setting MongoDB
- Docker - Setting NGINX
- Docker - Toolbox
- Docker - Setting ASP.Net
- Docker - Cloud
- Docker - Logging
- Docker - Compose
- Docker - Continuous Integration
- Docker - Kubernetes Architecture
- Docker - Working of Kubernetes
- Docker Useful Resources
- Docker - Quick Guide
- Docker - Useful Resources
- Docker - Discussion
Docker - File
In the earlier chapters, we have seen the various Image files such as Centos which get downloaded from Docker hub from which you can spin up containers. An example is again shown below.
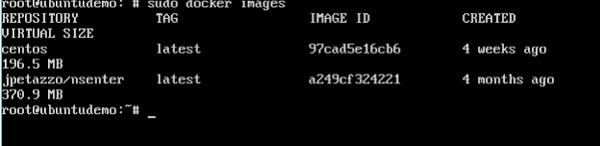
If we use the Docker images command, we can see the existing images in our system. From the above screenshot, we can see that there are two images: centos and nsenter.
But Docker also gives you the capability to create your own Docker images, and it can be done with the help of Docker Files. A Docker File is a simple text file with instructions on how to build your images.
The following steps explain how you should go about creating a Docker File.
Step 1 − Create a file called Docker File and edit it using vim. Please note that the name of the file has to be "Dockerfile" with "D" as capital.

Step 2 − Build your Docker File using the following instructions.
#This is a sample Image FROM ubuntu MAINTAINER demousr@gmail.com RUN apt-get update RUN apt-get install –y nginx CMD [“echo”,”Image created”]
The following points need to be noted about the above file −
The first line "#This is a sample Image" is a comment. You can add comments to the Docker File with the help of the # command
The next line has to start with the FROM keyword. It tells docker, from which base image you want to base your image from. In our example, we are creating an image from the ubuntu image.
The next command is the person who is going to maintain this image. Here you specify the MAINTAINER keyword and just mention the email ID.
The RUN command is used to run instructions against the image. In our case, we first update our Ubuntu system and then install the nginx server on our ubuntu image.
The last command is used to display a message to the user.
Step 3 − Save the file. In the next chapter, we will discuss how to build the image.
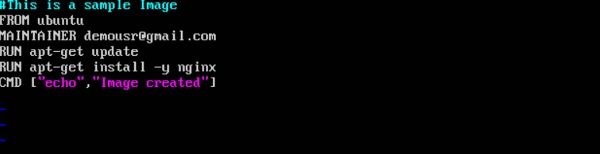